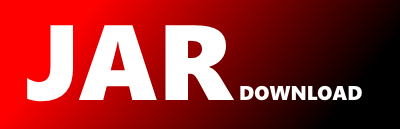
com.pulumi.awsnative.scheduler.kotlin.inputs.ScheduleTargetArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.scheduler.kotlin.inputs
import com.pulumi.awsnative.scheduler.inputs.ScheduleTargetArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* The schedule target.
* @property arn The Amazon Resource Name (ARN) of the target.
* @property deadLetterConfig An object that contains information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue.
* @property ecsParameters The templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation.
* @property eventBridgeParameters The templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation.
* @property input The text, or well-formed JSON, passed to the target. If you are configuring a templated Lambda, AWS Step Functions, or Amazon EventBridge target, the input must be a well-formed JSON. For all other target types, a JSON is not required. If you do not specify anything for this field, EventBridge Scheduler delivers a default notification to the target.
* @property kinesisParameters The templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation.
* @property retryPolicy A `RetryPolicy` object that includes information about the retry policy settings, including the maximum age of an event, and the maximum number of times EventBridge Scheduler will try to deliver the event to a target.
* @property roleArn The Amazon Resource Name (ARN) of the IAM role to be used for this target when the schedule is triggered.
* @property sageMakerPipelineParameters The templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation.
* @property sqsParameters The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Contains the message group ID to use when the target is a FIFO queue. If you specify an Amazon SQS FIFO queue as a target, the queue must have content-based deduplication enabled. For more information, see [Using the Amazon SQS message deduplication ID](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/using-messagededuplicationid-property.html) in the *Amazon SQS Developer Guide* .
*/
public data class ScheduleTargetArgs(
public val arn: Output,
public val deadLetterConfig: Output? = null,
public val ecsParameters: Output? = null,
public val eventBridgeParameters: Output? = null,
public val input: Output? = null,
public val kinesisParameters: Output? = null,
public val retryPolicy: Output? = null,
public val roleArn: Output,
public val sageMakerPipelineParameters: Output? = null,
public val sqsParameters: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.scheduler.inputs.ScheduleTargetArgs =
com.pulumi.awsnative.scheduler.inputs.ScheduleTargetArgs.builder()
.arn(arn.applyValue({ args0 -> args0 }))
.deadLetterConfig(deadLetterConfig?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ecsParameters(ecsParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.eventBridgeParameters(
eventBridgeParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.input(input?.applyValue({ args0 -> args0 }))
.kinesisParameters(kinesisParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retryPolicy(retryPolicy?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.roleArn(roleArn.applyValue({ args0 -> args0 }))
.sageMakerPipelineParameters(
sageMakerPipelineParameters?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.sqsParameters(sqsParameters?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [ScheduleTargetArgs].
*/
@PulumiTagMarker
public class ScheduleTargetArgsBuilder internal constructor() {
private var arn: Output? = null
private var deadLetterConfig: Output? = null
private var ecsParameters: Output? = null
private var eventBridgeParameters: Output? = null
private var input: Output? = null
private var kinesisParameters: Output? = null
private var retryPolicy: Output? = null
private var roleArn: Output? = null
private var sageMakerPipelineParameters: Output? = null
private var sqsParameters: Output? = null
/**
* @param value The Amazon Resource Name (ARN) of the target.
*/
@JvmName("wsmdxunqjvtgadmx")
public suspend fun arn(`value`: Output) {
this.arn = value
}
/**
* @param value An object that contains information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue.
*/
@JvmName("qxvxoymdhxljbkie")
public suspend fun deadLetterConfig(`value`: Output) {
this.deadLetterConfig = value
}
/**
* @param value The templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation.
*/
@JvmName("jywrlekrhejoobny")
public suspend fun ecsParameters(`value`: Output) {
this.ecsParameters = value
}
/**
* @param value The templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation.
*/
@JvmName("hucupykvhtpaefeq")
public suspend fun eventBridgeParameters(`value`: Output) {
this.eventBridgeParameters = value
}
/**
* @param value The text, or well-formed JSON, passed to the target. If you are configuring a templated Lambda, AWS Step Functions, or Amazon EventBridge target, the input must be a well-formed JSON. For all other target types, a JSON is not required. If you do not specify anything for this field, EventBridge Scheduler delivers a default notification to the target.
*/
@JvmName("okjovmeknevvwlcn")
public suspend fun input(`value`: Output) {
this.input = value
}
/**
* @param value The templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation.
*/
@JvmName("thxcvljksxrotqsp")
public suspend fun kinesisParameters(`value`: Output) {
this.kinesisParameters = value
}
/**
* @param value A `RetryPolicy` object that includes information about the retry policy settings, including the maximum age of an event, and the maximum number of times EventBridge Scheduler will try to deliver the event to a target.
*/
@JvmName("haihajjhnixsvclb")
public suspend fun retryPolicy(`value`: Output) {
this.retryPolicy = value
}
/**
* @param value The Amazon Resource Name (ARN) of the IAM role to be used for this target when the schedule is triggered.
*/
@JvmName("vkutywljjmklfsjs")
public suspend fun roleArn(`value`: Output) {
this.roleArn = value
}
/**
* @param value The templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation.
*/
@JvmName("mfmwbrubpkckqtkc")
public suspend fun sageMakerPipelineParameters(`value`: Output) {
this.sageMakerPipelineParameters = value
}
/**
* @param value The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Contains the message group ID to use when the target is a FIFO queue. If you specify an Amazon SQS FIFO queue as a target, the queue must have content-based deduplication enabled. For more information, see [Using the Amazon SQS message deduplication ID](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/using-messagededuplicationid-property.html) in the *Amazon SQS Developer Guide* .
*/
@JvmName("riyfmbjoaqfbmvbf")
public suspend fun sqsParameters(`value`: Output) {
this.sqsParameters = value
}
/**
* @param value The Amazon Resource Name (ARN) of the target.
*/
@JvmName("bjwhrnitsrgjlfgy")
public suspend fun arn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.arn = mapped
}
/**
* @param value An object that contains information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue.
*/
@JvmName("qnskgsoattneihap")
public suspend fun deadLetterConfig(`value`: ScheduleDeadLetterConfigArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deadLetterConfig = mapped
}
/**
* @param argument An object that contains information about an Amazon SQS queue that EventBridge Scheduler uses as a dead-letter queue for your schedule. If specified, EventBridge Scheduler delivers failed events that could not be successfully delivered to a target to the queue.
*/
@JvmName("mkijbiiltybincih")
public suspend fun deadLetterConfig(argument: suspend ScheduleDeadLetterConfigArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleDeadLetterConfigArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.deadLetterConfig = mapped
}
/**
* @param value The templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation.
*/
@JvmName("brrmbtwkqlgkbdhf")
public suspend fun ecsParameters(`value`: ScheduleEcsParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ecsParameters = mapped
}
/**
* @param argument The templated target type for the Amazon ECS [`RunTask`](https://docs.aws.amazon.com/AmazonECS/latest/APIReference/API_RunTask.html) API operation.
*/
@JvmName("ptxoykbrpmjmecex")
public suspend fun ecsParameters(argument: suspend ScheduleEcsParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleEcsParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.ecsParameters = mapped
}
/**
* @param value The templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation.
*/
@JvmName("xqmsgdfnqatnikyu")
public suspend fun eventBridgeParameters(`value`: ScheduleEventBridgeParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.eventBridgeParameters = mapped
}
/**
* @param argument The templated target type for the EventBridge [`PutEvents`](https://docs.aws.amazon.com/eventbridge/latest/APIReference/API_PutEvents.html) API operation.
*/
@JvmName("ffmsfwfhxcjsemmi")
public suspend fun eventBridgeParameters(argument: suspend ScheduleEventBridgeParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleEventBridgeParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.eventBridgeParameters = mapped
}
/**
* @param value The text, or well-formed JSON, passed to the target. If you are configuring a templated Lambda, AWS Step Functions, or Amazon EventBridge target, the input must be a well-formed JSON. For all other target types, a JSON is not required. If you do not specify anything for this field, EventBridge Scheduler delivers a default notification to the target.
*/
@JvmName("oegxkbgqswghogyp")
public suspend fun input(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.input = mapped
}
/**
* @param value The templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation.
*/
@JvmName("hdvgypedbjyucmpv")
public suspend fun kinesisParameters(`value`: ScheduleKinesisParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.kinesisParameters = mapped
}
/**
* @param argument The templated target type for the Amazon Kinesis [`PutRecord`](https://docs.aws.amazon.com/kinesis/latest/APIReference/API_PutRecord.html) API operation.
*/
@JvmName("iturcqigvutdgyqx")
public suspend fun kinesisParameters(argument: suspend ScheduleKinesisParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleKinesisParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.kinesisParameters = mapped
}
/**
* @param value A `RetryPolicy` object that includes information about the retry policy settings, including the maximum age of an event, and the maximum number of times EventBridge Scheduler will try to deliver the event to a target.
*/
@JvmName("pdmrepaycxqohihm")
public suspend fun retryPolicy(`value`: ScheduleRetryPolicyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retryPolicy = mapped
}
/**
* @param argument A `RetryPolicy` object that includes information about the retry policy settings, including the maximum age of an event, and the maximum number of times EventBridge Scheduler will try to deliver the event to a target.
*/
@JvmName("esibuymjjijgusgl")
public suspend fun retryPolicy(argument: suspend ScheduleRetryPolicyArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleRetryPolicyArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.retryPolicy = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of the IAM role to be used for this target when the schedule is triggered.
*/
@JvmName("cgklisasbhlfowgs")
public suspend fun roleArn(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.roleArn = mapped
}
/**
* @param value The templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation.
*/
@JvmName("dafthyagkoopktfu")
public suspend fun sageMakerPipelineParameters(`value`: ScheduleSageMakerPipelineParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sageMakerPipelineParameters = mapped
}
/**
* @param argument The templated target type for the Amazon SageMaker [`StartPipelineExecution`](https://docs.aws.amazon.com/sagemaker/latest/APIReference/API_StartPipelineExecution.html) API operation.
*/
@JvmName("ochmuntlafpfrrnx")
public suspend fun sageMakerPipelineParameters(argument: suspend ScheduleSageMakerPipelineParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleSageMakerPipelineParametersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.sageMakerPipelineParameters = mapped
}
/**
* @param value The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Contains the message group ID to use when the target is a FIFO queue. If you specify an Amazon SQS FIFO queue as a target, the queue must have content-based deduplication enabled. For more information, see [Using the Amazon SQS message deduplication ID](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/using-messagededuplicationid-property.html) in the *Amazon SQS Developer Guide* .
*/
@JvmName("qgdxvjxvhybsgjky")
public suspend fun sqsParameters(`value`: ScheduleSqsParametersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sqsParameters = mapped
}
/**
* @param argument The templated target type for the Amazon SQS [`SendMessage`](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/APIReference/API_SendMessage.html) API operation. Contains the message group ID to use when the target is a FIFO queue. If you specify an Amazon SQS FIFO queue as a target, the queue must have content-based deduplication enabled. For more information, see [Using the Amazon SQS message deduplication ID](https://docs.aws.amazon.com/AWSSimpleQueueService/latest/SQSDeveloperGuide/using-messagededuplicationid-property.html) in the *Amazon SQS Developer Guide* .
*/
@JvmName("tfdxuyppdrfconcf")
public suspend fun sqsParameters(argument: suspend ScheduleSqsParametersArgsBuilder.() -> Unit) {
val toBeMapped = ScheduleSqsParametersArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.sqsParameters = mapped
}
internal fun build(): ScheduleTargetArgs = ScheduleTargetArgs(
arn = arn ?: throw PulumiNullFieldException("arn"),
deadLetterConfig = deadLetterConfig,
ecsParameters = ecsParameters,
eventBridgeParameters = eventBridgeParameters,
input = input,
kinesisParameters = kinesisParameters,
retryPolicy = retryPolicy,
roleArn = roleArn ?: throw PulumiNullFieldException("roleArn"),
sageMakerPipelineParameters = sageMakerPipelineParameters,
sqsParameters = sqsParameters,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy