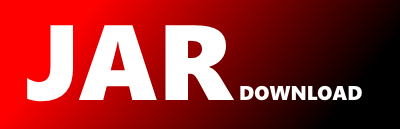
com.pulumi.awsnative.securityhub.kotlin.OrganizationConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.securityhub.kotlin
import com.pulumi.awsnative.securityhub.OrganizationConfigurationArgs.builder
import com.pulumi.awsnative.securityhub.kotlin.enums.OrganizationConfigurationAutoEnableStandards
import com.pulumi.awsnative.securityhub.kotlin.enums.OrganizationConfigurationConfigurationType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* The AWS::SecurityHub::OrganizationConfiguration resource represents the configuration of your organization in Security Hub. Only the Security Hub administrator account can create Organization Configuration resource in each region and can opt-in to Central Configuration only in the aggregation region of FindingAggregator.
* @property autoEnable Whether to automatically enable Security Hub in new member accounts when they join the organization.
* @property autoEnableStandards Whether to automatically enable Security Hub default standards in new member accounts when they join the organization.
* @property configurationType Indicates whether the organization uses local or central configuration.
*/
public data class OrganizationConfigurationArgs(
public val autoEnable: Output? = null,
public val autoEnableStandards: Output? = null,
public val configurationType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.securityhub.OrganizationConfigurationArgs =
com.pulumi.awsnative.securityhub.OrganizationConfigurationArgs.builder()
.autoEnable(autoEnable?.applyValue({ args0 -> args0 }))
.autoEnableStandards(
autoEnableStandards?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.configurationType(
configurationType?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [OrganizationConfigurationArgs].
*/
@PulumiTagMarker
public class OrganizationConfigurationArgsBuilder internal constructor() {
private var autoEnable: Output? = null
private var autoEnableStandards: Output? = null
private var configurationType: Output? = null
/**
* @param value Whether to automatically enable Security Hub in new member accounts when they join the organization.
*/
@JvmName("rkjhwyjipemuoybk")
public suspend fun autoEnable(`value`: Output) {
this.autoEnable = value
}
/**
* @param value Whether to automatically enable Security Hub default standards in new member accounts when they join the organization.
*/
@JvmName("xbewxutsxepiojci")
public suspend fun autoEnableStandards(`value`: Output) {
this.autoEnableStandards = value
}
/**
* @param value Indicates whether the organization uses local or central configuration.
*/
@JvmName("waigmdbgsedfjowh")
public suspend fun configurationType(`value`: Output) {
this.configurationType = value
}
/**
* @param value Whether to automatically enable Security Hub in new member accounts when they join the organization.
*/
@JvmName("uknworjcrjckadoa")
public suspend fun autoEnable(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoEnable = mapped
}
/**
* @param value Whether to automatically enable Security Hub default standards in new member accounts when they join the organization.
*/
@JvmName("rxeiryimreohobgi")
public suspend fun autoEnableStandards(`value`: OrganizationConfigurationAutoEnableStandards?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.autoEnableStandards = mapped
}
/**
* @param value Indicates whether the organization uses local or central configuration.
*/
@JvmName("ycvxywcqdpjvobfj")
public suspend fun configurationType(`value`: OrganizationConfigurationConfigurationType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.configurationType = mapped
}
internal fun build(): OrganizationConfigurationArgs = OrganizationConfigurationArgs(
autoEnable = autoEnable,
autoEnableStandards = autoEnableStandards,
configurationType = configurationType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy