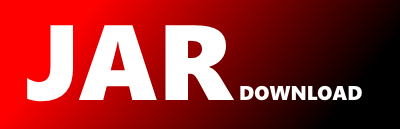
com.pulumi.awsnative.servicecatalog.kotlin.ServiceActionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.servicecatalog.kotlin
import com.pulumi.awsnative.servicecatalog.ServiceActionArgs.builder
import com.pulumi.awsnative.servicecatalog.kotlin.enums.ServiceActionAcceptLanguage
import com.pulumi.awsnative.servicecatalog.kotlin.enums.ServiceActionDefinitionType
import com.pulumi.awsnative.servicecatalog.kotlin.inputs.ServiceActionDefinitionParameterArgs
import com.pulumi.awsnative.servicecatalog.kotlin.inputs.ServiceActionDefinitionParameterArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Resource Schema for AWS::ServiceCatalog::ServiceAction
* @property acceptLanguage The language code.
* - `en` - English (default)
* - `jp` - Japanese
* - `zh` - Chinese
* @property definition A map that defines the self-service action.
* @property definitionType The self-service action definition type. For example, `SSM_AUTOMATION` .
* @property description The self-service action description.
* @property name The self-service action name.
*/
public data class ServiceActionArgs(
public val acceptLanguage: Output? = null,
public val definition: Output>? = null,
public val definitionType: Output? = null,
public val description: Output? = null,
public val name: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.servicecatalog.ServiceActionArgs =
com.pulumi.awsnative.servicecatalog.ServiceActionArgs.builder()
.acceptLanguage(acceptLanguage?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.definition(
definition?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.definitionType(definitionType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.description(description?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ServiceActionArgs].
*/
@PulumiTagMarker
public class ServiceActionArgsBuilder internal constructor() {
private var acceptLanguage: Output? = null
private var definition: Output>? = null
private var definitionType: Output? = null
private var description: Output? = null
private var name: Output? = null
/**
* @param value The language code.
* - `en` - English (default)
* - `jp` - Japanese
* - `zh` - Chinese
*/
@JvmName("dpktqqeranowlwit")
public suspend fun acceptLanguage(`value`: Output) {
this.acceptLanguage = value
}
/**
* @param value A map that defines the self-service action.
*/
@JvmName("mmgmhlkisaaqthot")
public suspend fun definition(`value`: Output>) {
this.definition = value
}
@JvmName("ghefqdclevapoxsn")
public suspend fun definition(vararg values: Output) {
this.definition = Output.all(values.asList())
}
/**
* @param values A map that defines the self-service action.
*/
@JvmName("ijrvehkqgdgsurac")
public suspend fun definition(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy