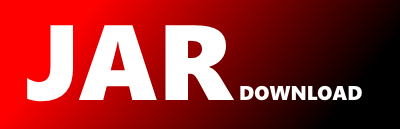
com.pulumi.awsnative.shield.kotlin.Protection.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.shield.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.shield.kotlin.outputs.ProtectionApplicationLayerAutomaticResponseConfiguration
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.shield.kotlin.outputs.ProtectionApplicationLayerAutomaticResponseConfiguration.Companion.toKotlin as protectionApplicationLayerAutomaticResponseConfigurationToKotlin
/**
* Builder for [Protection].
*/
@PulumiTagMarker
public class ProtectionResourceBuilder internal constructor() {
public var name: String? = null
public var args: ProtectionArgs = ProtectionArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend ProtectionArgsBuilder.() -> Unit) {
val builder = ProtectionArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): Protection {
val builtJavaResource = com.pulumi.awsnative.shield.Protection(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return Protection(builtJavaResource)
}
}
/**
* Enables AWS Shield Advanced for a specific AWS resource. The resource can be an Amazon CloudFront distribution, Amazon Route 53 hosted zone, AWS Global Accelerator standard accelerator, Elastic IP Address, Application Load Balancer, or a Classic Load Balancer. You can protect Amazon EC2 instances and Network Load Balancers by association with protected Amazon EC2 Elastic IP addresses.
*/
public class Protection internal constructor(
override val javaResource: com.pulumi.awsnative.shield.Protection,
) : KotlinCustomResource(javaResource, ProtectionMapper) {
/**
* The automatic application layer DDoS mitigation settings for the protection. This configuration determines whether Shield Advanced automatically manages rules in the web ACL in order to respond to application layer events that Shield Advanced determines to be DDoS attacks.
* If you use AWS CloudFormation to manage the web ACLs that you use with Shield Advanced automatic mitigation, see the additional guidance about web ACL management in the `AWS::WAFv2::WebACL` resource description.
*/
public val applicationLayerAutomaticResponseConfiguration:
Output?
get() = javaResource.applicationLayerAutomaticResponseConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
protectionApplicationLayerAutomaticResponseConfigurationToKotlin(args0)
})
}).orElse(null)
})
/**
* The Amazon Resource Names (ARNs) of the health check to associate with the protection.
*/
public val healthCheckArns: Output>?
get() = javaResource.healthCheckArns().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* Friendly name for the Protection.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The ARN (Amazon Resource Name) of the protection.
*/
public val protectionArn: Output
get() = javaResource.protectionArn().applyValue({ args0 -> args0 })
/**
* The unique identifier (ID) of the protection.
*/
public val protectionId: Output
get() = javaResource.protectionId().applyValue({ args0 -> args0 })
/**
* The ARN (Amazon Resource Name) of the resource to be protected.
*/
public val resourceArn: Output
get() = javaResource.resourceArn().applyValue({ args0 -> args0 })
/**
* One or more tag key-value pairs for the Protection object.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
}
public object ProtectionMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.shield.Protection::class == javaResource::class
override fun map(javaResource: Resource): Protection = Protection(
javaResource as
com.pulumi.awsnative.shield.Protection,
)
}
/**
* @see [Protection].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [Protection].
*/
public suspend fun protection(name: String, block: suspend ProtectionResourceBuilder.() -> Unit): Protection {
val builder = ProtectionResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [Protection].
* @param name The _unique_ name of the resulting resource.
*/
public fun protection(name: String): Protection {
val builder = ProtectionResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy