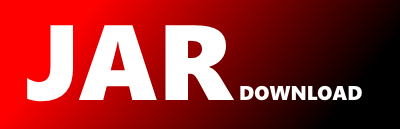
com.pulumi.awsnative.sns.kotlin.SubscriptionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.sns.kotlin
import com.pulumi.awsnative.sns.SubscriptionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Any
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::SNS::Subscription
* @property deliveryPolicy The delivery policy JSON assigned to the subscription. Enables the subscriber to define the message delivery retry strategy in the case of an HTTP/S endpoint subscribed to the topic.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
* @property endpoint The subscription's endpoint. The endpoint value depends on the protocol that you specify.
* @property filterPolicy The filter policy JSON assigned to the subscription. Enables the subscriber to filter out unwanted messages.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
* @property filterPolicyScope This attribute lets you choose the filtering scope by using one of the following string value types: MessageAttributes (default) and MessageBody.
* @property protocol The subscription's protocol.
* @property rawMessageDelivery When set to true, enables raw message delivery. Raw messages don't contain any JSON formatting and can be sent to Amazon SQS and HTTP/S endpoints.
* @property redrivePolicy When specified, sends undeliverable messages to the specified Amazon SQS dead-letter queue. Messages that can't be delivered due to client errors are held in the dead-letter queue for further analysis or reprocessing.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
* @property region For cross-region subscriptions, the region in which the topic resides.If no region is specified, AWS CloudFormation uses the region of the caller as the default.
* @property replayPolicy Specifies whether Amazon SNS resends the notification to the subscription when a message's attribute changes.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
* @property subscriptionRoleArn This property applies only to Amazon Data Firehose delivery stream subscriptions.
* @property topicArn The ARN of the topic to subscribe to.
*/
public data class SubscriptionArgs(
public val deliveryPolicy: Output? = null,
public val endpoint: Output? = null,
public val filterPolicy: Output? = null,
public val filterPolicyScope: Output? = null,
public val protocol: Output? = null,
public val rawMessageDelivery: Output? = null,
public val redrivePolicy: Output? = null,
public val region: Output? = null,
public val replayPolicy: Output? = null,
public val subscriptionRoleArn: Output? = null,
public val topicArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.sns.SubscriptionArgs =
com.pulumi.awsnative.sns.SubscriptionArgs.builder()
.deliveryPolicy(deliveryPolicy?.applyValue({ args0 -> args0 }))
.endpoint(endpoint?.applyValue({ args0 -> args0 }))
.filterPolicy(filterPolicy?.applyValue({ args0 -> args0 }))
.filterPolicyScope(filterPolicyScope?.applyValue({ args0 -> args0 }))
.protocol(protocol?.applyValue({ args0 -> args0 }))
.rawMessageDelivery(rawMessageDelivery?.applyValue({ args0 -> args0 }))
.redrivePolicy(redrivePolicy?.applyValue({ args0 -> args0 }))
.region(region?.applyValue({ args0 -> args0 }))
.replayPolicy(replayPolicy?.applyValue({ args0 -> args0 }))
.subscriptionRoleArn(subscriptionRoleArn?.applyValue({ args0 -> args0 }))
.topicArn(topicArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SubscriptionArgs].
*/
@PulumiTagMarker
public class SubscriptionArgsBuilder internal constructor() {
private var deliveryPolicy: Output? = null
private var endpoint: Output? = null
private var filterPolicy: Output? = null
private var filterPolicyScope: Output? = null
private var protocol: Output? = null
private var rawMessageDelivery: Output? = null
private var redrivePolicy: Output? = null
private var region: Output? = null
private var replayPolicy: Output? = null
private var subscriptionRoleArn: Output? = null
private var topicArn: Output? = null
/**
* @param value The delivery policy JSON assigned to the subscription. Enables the subscriber to define the message delivery retry strategy in the case of an HTTP/S endpoint subscribed to the topic.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("kgnchfbtrrmbiyrw")
public suspend fun deliveryPolicy(`value`: Output) {
this.deliveryPolicy = value
}
/**
* @param value The subscription's endpoint. The endpoint value depends on the protocol that you specify.
*/
@JvmName("honnbswirjhiwpit")
public suspend fun endpoint(`value`: Output) {
this.endpoint = value
}
/**
* @param value The filter policy JSON assigned to the subscription. Enables the subscriber to filter out unwanted messages.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("mdipupcfhmeakkcj")
public suspend fun filterPolicy(`value`: Output) {
this.filterPolicy = value
}
/**
* @param value This attribute lets you choose the filtering scope by using one of the following string value types: MessageAttributes (default) and MessageBody.
*/
@JvmName("pugaaaowljymnfwg")
public suspend fun filterPolicyScope(`value`: Output) {
this.filterPolicyScope = value
}
/**
* @param value The subscription's protocol.
*/
@JvmName("oghbfapxdridiqlw")
public suspend fun protocol(`value`: Output) {
this.protocol = value
}
/**
* @param value When set to true, enables raw message delivery. Raw messages don't contain any JSON formatting and can be sent to Amazon SQS and HTTP/S endpoints.
*/
@JvmName("blslxdkdnctrhrvo")
public suspend fun rawMessageDelivery(`value`: Output) {
this.rawMessageDelivery = value
}
/**
* @param value When specified, sends undeliverable messages to the specified Amazon SQS dead-letter queue. Messages that can't be delivered due to client errors are held in the dead-letter queue for further analysis or reprocessing.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("fochovogekeqpwbk")
public suspend fun redrivePolicy(`value`: Output) {
this.redrivePolicy = value
}
/**
* @param value For cross-region subscriptions, the region in which the topic resides.If no region is specified, AWS CloudFormation uses the region of the caller as the default.
*/
@JvmName("lrwxluorsggtmwfn")
public suspend fun region(`value`: Output) {
this.region = value
}
/**
* @param value Specifies whether Amazon SNS resends the notification to the subscription when a message's attribute changes.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("qmctktvgvkbhlnaj")
public suspend fun replayPolicy(`value`: Output) {
this.replayPolicy = value
}
/**
* @param value This property applies only to Amazon Data Firehose delivery stream subscriptions.
*/
@JvmName("arqjbwkvwbujliop")
public suspend fun subscriptionRoleArn(`value`: Output) {
this.subscriptionRoleArn = value
}
/**
* @param value The ARN of the topic to subscribe to.
*/
@JvmName("dsgdikidpmprvfjh")
public suspend fun topicArn(`value`: Output) {
this.topicArn = value
}
/**
* @param value The delivery policy JSON assigned to the subscription. Enables the subscriber to define the message delivery retry strategy in the case of an HTTP/S endpoint subscribed to the topic.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("fflnxygnktxqbpjk")
public suspend fun deliveryPolicy(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deliveryPolicy = mapped
}
/**
* @param value The subscription's endpoint. The endpoint value depends on the protocol that you specify.
*/
@JvmName("qumquyafrytrhali")
public suspend fun endpoint(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.endpoint = mapped
}
/**
* @param value The filter policy JSON assigned to the subscription. Enables the subscriber to filter out unwanted messages.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("mawxmwlocvmiilrw")
public suspend fun filterPolicy(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filterPolicy = mapped
}
/**
* @param value This attribute lets you choose the filtering scope by using one of the following string value types: MessageAttributes (default) and MessageBody.
*/
@JvmName("mmfunycvexclalfg")
public suspend fun filterPolicyScope(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.filterPolicyScope = mapped
}
/**
* @param value The subscription's protocol.
*/
@JvmName("vqcfrmbhoialnyvn")
public suspend fun protocol(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value When set to true, enables raw message delivery. Raw messages don't contain any JSON formatting and can be sent to Amazon SQS and HTTP/S endpoints.
*/
@JvmName("hshdglcddvsjnapb")
public suspend fun rawMessageDelivery(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rawMessageDelivery = mapped
}
/**
* @param value When specified, sends undeliverable messages to the specified Amazon SQS dead-letter queue. Messages that can't be delivered due to client errors are held in the dead-letter queue for further analysis or reprocessing.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("imlnelinivhlihkd")
public suspend fun redrivePolicy(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.redrivePolicy = mapped
}
/**
* @param value For cross-region subscriptions, the region in which the topic resides.If no region is specified, AWS CloudFormation uses the region of the caller as the default.
*/
@JvmName("uulsrrqnnpxynprx")
public suspend fun region(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.region = mapped
}
/**
* @param value Specifies whether Amazon SNS resends the notification to the subscription when a message's attribute changes.
* Search the [CloudFormation User Guide](https://docs.aws.amazon.com/cloudformation/) for `AWS::SNS::Subscription` for more information about the expected schema for this property.
*/
@JvmName("aqxwyffveuyvwkbs")
public suspend fun replayPolicy(`value`: Any?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.replayPolicy = mapped
}
/**
* @param value This property applies only to Amazon Data Firehose delivery stream subscriptions.
*/
@JvmName("ayohjovajydgtocd")
public suspend fun subscriptionRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.subscriptionRoleArn = mapped
}
/**
* @param value The ARN of the topic to subscribe to.
*/
@JvmName("ahrwepktqqklhtye")
public suspend fun topicArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.topicArn = mapped
}
internal fun build(): SubscriptionArgs = SubscriptionArgs(
deliveryPolicy = deliveryPolicy,
endpoint = endpoint,
filterPolicy = filterPolicy,
filterPolicyScope = filterPolicyScope,
protocol = protocol,
rawMessageDelivery = rawMessageDelivery,
redrivePolicy = redrivePolicy,
region = region,
replayPolicy = replayPolicy,
subscriptionRoleArn = subscriptionRoleArn,
topicArn = topicArn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy