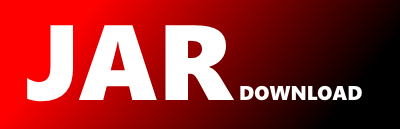
com.pulumi.awsnative.ssm.kotlin.outputs.GetAssociationResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ssm.kotlin.outputs
import com.pulumi.awsnative.ssm.kotlin.enums.AssociationComplianceSeverity
import com.pulumi.awsnative.ssm.kotlin.enums.AssociationSyncCompliance
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
*
* @property applyOnlyAtCronInterval By default, when you create a new association, the system runs it immediately after it is created and then according to the schedule you specified. Specify this option if you don't want an association to run immediately after you create it. This parameter is not supported for rate expressions.
* @property associationId Unique identifier of the association.
* @property associationName The name of the association.
* @property automationTargetParameterName Choose the parameter that will define how your automation will branch out. This target is required for associations that use an Automation runbook and target resources by using rate controls. Automation is a capability of AWS Systems Manager .
* @property calendarNames The names or Amazon Resource Names (ARNs) of the Change Calendar type documents your associations are gated under. The associations only run when that Change Calendar is open. For more information, see [AWS Systems Manager Change Calendar](https://docs.aws.amazon.com/systems-manager/latest/userguide/systems-manager-change-calendar) .
* @property complianceSeverity The severity level that is assigned to the association.
* @property documentVersion The version of the SSM document to associate with the target.
* @property instanceId The ID of the instance that the SSM document is associated with.
* @property maxConcurrency The maximum number of targets allowed to run the association at the same time. You can specify a number, for example 10, or a percentage of the target set, for example 10%. The default value is 100%, which means all targets run the association at the same time.
* If a new managed node starts and attempts to run an association while Systems Manager is running `MaxConcurrency` associations, the association is allowed to run. During the next association interval, the new managed node will process its association within the limit specified for `MaxConcurrency` .
* @property maxErrors The number of errors that are allowed before the system stops sending requests to run the association on additional targets. You can specify either an absolute number of errors, for example 10, or a percentage of the target set, for example 10%. If you specify 3, for example, the system stops sending requests when the fourth error is received. If you specify 0, then the system stops sending requests after the first error is returned. If you run an association on 50 managed nodes and set `MaxError` to 10%, then the system stops sending the request when the sixth error is received.
* Executions that are already running an association when `MaxErrors` is reached are allowed to complete, but some of these executions may fail as well. If you need to ensure that there won't be more than max-errors failed executions, set `MaxConcurrency` to 1 so that executions proceed one at a time.
* @property name The name of the SSM document.
* @property outputLocation An Amazon Simple Storage Service (Amazon S3) bucket where you want to store the output details of the request.
* @property parameters Parameter values that the SSM document uses at runtime.
* @property scheduleExpression A Cron or Rate expression that specifies when the association is applied to the target.
* @property scheduleOffset Number of days to wait after the scheduled day to run an association.
* @property syncCompliance The mode for generating association compliance. You can specify `AUTO` or `MANUAL` . In `AUTO` mode, the system uses the status of the association execution to determine the compliance status. If the association execution runs successfully, then the association is `COMPLIANT` . If the association execution doesn't run successfully, the association is `NON-COMPLIANT` .
* In `MANUAL` mode, you must specify the `AssociationId` as a parameter for the `PutComplianceItems` API action. In this case, compliance data is not managed by State Manager. It is managed by your direct call to the `PutComplianceItems` API action.
* By default, all associations use `AUTO` mode.
* @property targets The targets that the SSM document sends commands to.
*/
public data class GetAssociationResult(
public val applyOnlyAtCronInterval: Boolean? = null,
public val associationId: String? = null,
public val associationName: String? = null,
public val automationTargetParameterName: String? = null,
public val calendarNames: List? = null,
public val complianceSeverity: AssociationComplianceSeverity? = null,
public val documentVersion: String? = null,
public val instanceId: String? = null,
public val maxConcurrency: String? = null,
public val maxErrors: String? = null,
public val name: String? = null,
public val outputLocation: AssociationInstanceAssociationOutputLocation? = null,
public val parameters: Map>? = null,
public val scheduleExpression: String? = null,
public val scheduleOffset: Int? = null,
public val syncCompliance: AssociationSyncCompliance? = null,
public val targets: List? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.ssm.outputs.GetAssociationResult): GetAssociationResult = GetAssociationResult(
applyOnlyAtCronInterval = javaType.applyOnlyAtCronInterval().map({ args0 -> args0 }).orElse(null),
associationId = javaType.associationId().map({ args0 -> args0 }).orElse(null),
associationName = javaType.associationName().map({ args0 -> args0 }).orElse(null),
automationTargetParameterName = javaType.automationTargetParameterName().map({ args0 ->
args0
}).orElse(null),
calendarNames = javaType.calendarNames().map({ args0 -> args0 }),
complianceSeverity = javaType.complianceSeverity().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.ssm.kotlin.enums.AssociationComplianceSeverity.Companion.toKotlin(args0)
})
}).orElse(null),
documentVersion = javaType.documentVersion().map({ args0 -> args0 }).orElse(null),
instanceId = javaType.instanceId().map({ args0 -> args0 }).orElse(null),
maxConcurrency = javaType.maxConcurrency().map({ args0 -> args0 }).orElse(null),
maxErrors = javaType.maxErrors().map({ args0 -> args0 }).orElse(null),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
outputLocation = javaType.outputLocation().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.ssm.kotlin.outputs.AssociationInstanceAssociationOutputLocation.Companion.toKotlin(args0)
})
}).orElse(null),
parameters = javaType.parameters().map({ args0 ->
args0.key.to(
args0.value.map({ args0 ->
args0
}),
)
}).toMap(),
scheduleExpression = javaType.scheduleExpression().map({ args0 -> args0 }).orElse(null),
scheduleOffset = javaType.scheduleOffset().map({ args0 -> args0 }).orElse(null),
syncCompliance = javaType.syncCompliance().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.ssm.kotlin.enums.AssociationSyncCompliance.Companion.toKotlin(args0)
})
}).orElse(null),
targets = javaType.targets().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.ssm.kotlin.outputs.AssociationTarget.Companion.toKotlin(args0)
})
}),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy