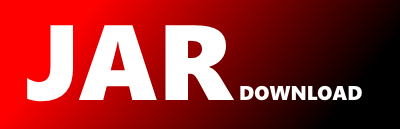
com.pulumi.awsnative.ssmcontacts.kotlin.ContactChannelArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ssmcontacts.kotlin
import com.pulumi.awsnative.ssmcontacts.ContactChannelArgs.builder
import com.pulumi.awsnative.ssmcontacts.kotlin.enums.ContactChannelChannelType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* Resource Type definition for AWS::SSMContacts::ContactChannel
* @property channelAddress The details that SSM Incident Manager uses when trying to engage the contact channel.
* @property channelName The device name. String of 6 to 50 alphabetical, numeric, dash, and underscore characters.
* @property channelType Device type, which specify notification channel. Currently supported values: "SMS", "VOICE", "EMAIL", "CHATBOT.
* @property contactId ARN of the contact resource
* @property deferActivation If you want to activate the channel at a later time, you can choose to defer activation. SSM Incident Manager can't engage your contact channel until it has been activated.
*/
public data class ContactChannelArgs(
public val channelAddress: Output? = null,
public val channelName: Output? = null,
public val channelType: Output? = null,
public val contactId: Output? = null,
public val deferActivation: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ssmcontacts.ContactChannelArgs =
com.pulumi.awsnative.ssmcontacts.ContactChannelArgs.builder()
.channelAddress(channelAddress?.applyValue({ args0 -> args0 }))
.channelName(channelName?.applyValue({ args0 -> args0 }))
.channelType(channelType?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.contactId(contactId?.applyValue({ args0 -> args0 }))
.deferActivation(deferActivation?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ContactChannelArgs].
*/
@PulumiTagMarker
public class ContactChannelArgsBuilder internal constructor() {
private var channelAddress: Output? = null
private var channelName: Output? = null
private var channelType: Output? = null
private var contactId: Output? = null
private var deferActivation: Output? = null
/**
* @param value The details that SSM Incident Manager uses when trying to engage the contact channel.
*/
@JvmName("dfhqtfodrkrccphw")
public suspend fun channelAddress(`value`: Output) {
this.channelAddress = value
}
/**
* @param value The device name. String of 6 to 50 alphabetical, numeric, dash, and underscore characters.
*/
@JvmName("kuoocbaovqcvtjtr")
public suspend fun channelName(`value`: Output) {
this.channelName = value
}
/**
* @param value Device type, which specify notification channel. Currently supported values: "SMS", "VOICE", "EMAIL", "CHATBOT.
*/
@JvmName("uyovnabfniuwxfvk")
public suspend fun channelType(`value`: Output) {
this.channelType = value
}
/**
* @param value ARN of the contact resource
*/
@JvmName("xrbgoummhqbbtduu")
public suspend fun contactId(`value`: Output) {
this.contactId = value
}
/**
* @param value If you want to activate the channel at a later time, you can choose to defer activation. SSM Incident Manager can't engage your contact channel until it has been activated.
*/
@JvmName("jcjiborpcfblgpru")
public suspend fun deferActivation(`value`: Output) {
this.deferActivation = value
}
/**
* @param value The details that SSM Incident Manager uses when trying to engage the contact channel.
*/
@JvmName("hbuvhbhwesornmtl")
public suspend fun channelAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelAddress = mapped
}
/**
* @param value The device name. String of 6 to 50 alphabetical, numeric, dash, and underscore characters.
*/
@JvmName("blrgqhtysnjfsoyq")
public suspend fun channelName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelName = mapped
}
/**
* @param value Device type, which specify notification channel. Currently supported values: "SMS", "VOICE", "EMAIL", "CHATBOT.
*/
@JvmName("lrwkubcjrwewrqin")
public suspend fun channelType(`value`: ContactChannelChannelType?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelType = mapped
}
/**
* @param value ARN of the contact resource
*/
@JvmName("xsrptffjpohwsraf")
public suspend fun contactId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.contactId = mapped
}
/**
* @param value If you want to activate the channel at a later time, you can choose to defer activation. SSM Incident Manager can't engage your contact channel until it has been activated.
*/
@JvmName("aqliwkumlsykwpcu")
public suspend fun deferActivation(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.deferActivation = mapped
}
internal fun build(): ContactChannelArgs = ContactChannelArgs(
channelAddress = channelAddress,
channelName = channelName,
channelType = channelType,
contactId = contactId,
deferActivation = deferActivation,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy