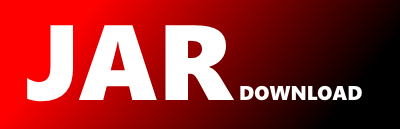
com.pulumi.awsnative.ssmcontacts.kotlin.inputs.RotationRecurrenceSettingsArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.ssmcontacts.kotlin.inputs
import com.pulumi.awsnative.ssmcontacts.inputs.RotationRecurrenceSettingsArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Information about when an on-call rotation is in effect and how long the rotation period lasts.
* @property dailySettings Information about on-call rotations that recur daily.
* @property monthlySettings Information about on-call rotations that recur monthly.
* @property numberOfOnCalls Number of Oncalls per shift.
* @property recurrenceMultiplier The number of days, weeks, or months a single rotation lasts.
* @property shiftCoverages Information about the days of the week included in on-call rotation coverage.
* @property weeklySettings Information about on-call rotations that recur weekly.
*/
public data class RotationRecurrenceSettingsArgs(
public val dailySettings: Output>? = null,
public val monthlySettings: Output>? = null,
public val numberOfOnCalls: Output? = null,
public val recurrenceMultiplier: Output? = null,
public val shiftCoverages: Output>? = null,
public val weeklySettings: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.ssmcontacts.inputs.RotationRecurrenceSettingsArgs =
com.pulumi.awsnative.ssmcontacts.inputs.RotationRecurrenceSettingsArgs.builder()
.dailySettings(dailySettings?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.monthlySettings(
monthlySettings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.numberOfOnCalls(numberOfOnCalls?.applyValue({ args0 -> args0 }))
.recurrenceMultiplier(recurrenceMultiplier?.applyValue({ args0 -> args0 }))
.shiftCoverages(
shiftCoverages?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.weeklySettings(
weeklySettings?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [RotationRecurrenceSettingsArgs].
*/
@PulumiTagMarker
public class RotationRecurrenceSettingsArgsBuilder internal constructor() {
private var dailySettings: Output>? = null
private var monthlySettings: Output>? = null
private var numberOfOnCalls: Output? = null
private var recurrenceMultiplier: Output? = null
private var shiftCoverages: Output>? = null
private var weeklySettings: Output>? = null
/**
* @param value Information about on-call rotations that recur daily.
*/
@JvmName("ybfyqxdtokybnwtf")
public suspend fun dailySettings(`value`: Output>) {
this.dailySettings = value
}
@JvmName("axnaehrkcmcgqrgq")
public suspend fun dailySettings(vararg values: Output) {
this.dailySettings = Output.all(values.asList())
}
/**
* @param values Information about on-call rotations that recur daily.
*/
@JvmName("eqrlgcfjlxvdlxld")
public suspend fun dailySettings(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy