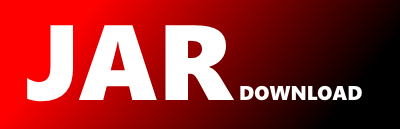
com.pulumi.awsnative.stepfunctions.kotlin.outputs.GetStateMachineResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.stepfunctions.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property arn Returns the ARN of the resource.
* @property definitionString The Amazon States Language definition of the state machine. The state machine definition must be in JSON. See [Amazon States Language](https://docs.aws.amazon.com/step-functions/latest/dg/concepts-amazon-states-language.html) .
* @property encryptionConfiguration Encryption configuration for the state machine.
* @property loggingConfiguration Defines what execution history events are logged and where they are logged.
* > By default, the `level` is set to `OFF` . For more information see [Log Levels](https://docs.aws.amazon.com/step-functions/latest/dg/cloudwatch-log-level.html) in the AWS Step Functions User Guide.
* @property name Returns the name of the state machine. For example:
* `{ "Fn::GetAtt": ["MyStateMachine", "Name"] }`
* Returns the name of your state machine:
* `HelloWorld-StateMachine`
* If you did not specify the name it will be similar to the following:
* `MyStateMachine-1234abcdefgh`
* For more information about using `Fn::GetAtt` , see [Fn::GetAtt](https://docs.aws.amazon.com/AWSCloudFormation/latest/UserGuide/intrinsic-function-reference-getatt.html) .
* @property roleArn The Amazon Resource Name (ARN) of the IAM role to use for this state machine.
* @property stateMachineRevisionId Identifier for a state machine revision, which is an immutable, read-only snapshot of a state machine’s definition and configuration.
* @property tags The list of tags to add to a resource.
* Tags may only contain Unicode letters, digits, white space, or these symbols: `_ . : / = + - @` .
* @property tracingConfiguration Selects whether or not the state machine's AWS X-Ray tracing is enabled.
*/
public data class GetStateMachineResult(
public val arn: String? = null,
public val definitionString: String? = null,
public val encryptionConfiguration: StateMachineEncryptionConfiguration? = null,
public val loggingConfiguration: StateMachineLoggingConfiguration? = null,
public val name: String? = null,
public val roleArn: String? = null,
public val stateMachineRevisionId: String? = null,
public val tags: List? = null,
public val tracingConfiguration: StateMachineTracingConfiguration? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.stepfunctions.outputs.GetStateMachineResult): GetStateMachineResult = GetStateMachineResult(
arn = javaType.arn().map({ args0 -> args0 }).orElse(null),
definitionString = javaType.definitionString().map({ args0 -> args0 }).orElse(null),
encryptionConfiguration = javaType.encryptionConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.stepfunctions.kotlin.outputs.StateMachineEncryptionConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
loggingConfiguration = javaType.loggingConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.stepfunctions.kotlin.outputs.StateMachineLoggingConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
name = javaType.name().map({ args0 -> args0 }).orElse(null),
roleArn = javaType.roleArn().map({ args0 -> args0 }).orElse(null),
stateMachineRevisionId = javaType.stateMachineRevisionId().map({ args0 -> args0 }).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
tracingConfiguration = javaType.tracingConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.stepfunctions.kotlin.outputs.StateMachineTracingConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy