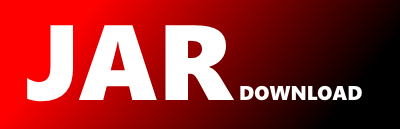
com.pulumi.awsnative.supportapp.kotlin.SlackChannelConfigurationArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.supportapp.kotlin
import com.pulumi.awsnative.supportapp.SlackChannelConfigurationArgs.builder
import com.pulumi.awsnative.supportapp.kotlin.enums.SlackChannelConfigurationNotifyOnCaseSeverity
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
* An AWS Support App resource that creates, updates, lists and deletes Slack channel configurations.
* ## Example Usage
* ### Example
* No Java example available.
* ### Example
* No Java example available.
* @property channelId The channel ID in Slack, which identifies a channel within a workspace.
* @property channelName The channel name in Slack.
* @property channelRoleArn The Amazon Resource Name (ARN) of an IAM role that grants the AWS Support App access to perform operations for AWS services.
* @property notifyOnAddCorrespondenceToCase Whether to notify when a correspondence is added to a case.
* @property notifyOnCaseSeverity The severity level of a support case that a customer wants to get notified for.
* @property notifyOnCreateOrReopenCase Whether to notify when a case is created or reopened.
* @property notifyOnResolveCase Whether to notify when a case is resolved.
* @property teamId The team ID in Slack, which uniquely identifies a workspace.
*/
public data class SlackChannelConfigurationArgs(
public val channelId: Output? = null,
public val channelName: Output? = null,
public val channelRoleArn: Output? = null,
public val notifyOnAddCorrespondenceToCase: Output? = null,
public val notifyOnCaseSeverity: Output? = null,
public val notifyOnCreateOrReopenCase: Output? = null,
public val notifyOnResolveCase: Output? = null,
public val teamId: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.supportapp.SlackChannelConfigurationArgs =
com.pulumi.awsnative.supportapp.SlackChannelConfigurationArgs.builder()
.channelId(channelId?.applyValue({ args0 -> args0 }))
.channelName(channelName?.applyValue({ args0 -> args0 }))
.channelRoleArn(channelRoleArn?.applyValue({ args0 -> args0 }))
.notifyOnAddCorrespondenceToCase(notifyOnAddCorrespondenceToCase?.applyValue({ args0 -> args0 }))
.notifyOnCaseSeverity(
notifyOnCaseSeverity?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.notifyOnCreateOrReopenCase(notifyOnCreateOrReopenCase?.applyValue({ args0 -> args0 }))
.notifyOnResolveCase(notifyOnResolveCase?.applyValue({ args0 -> args0 }))
.teamId(teamId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SlackChannelConfigurationArgs].
*/
@PulumiTagMarker
public class SlackChannelConfigurationArgsBuilder internal constructor() {
private var channelId: Output? = null
private var channelName: Output? = null
private var channelRoleArn: Output? = null
private var notifyOnAddCorrespondenceToCase: Output? = null
private var notifyOnCaseSeverity: Output? = null
private var notifyOnCreateOrReopenCase: Output? = null
private var notifyOnResolveCase: Output? = null
private var teamId: Output? = null
/**
* @param value The channel ID in Slack, which identifies a channel within a workspace.
*/
@JvmName("vwfbtxxmgmwtachd")
public suspend fun channelId(`value`: Output) {
this.channelId = value
}
/**
* @param value The channel name in Slack.
*/
@JvmName("bgallnlvtdsvgjbo")
public suspend fun channelName(`value`: Output) {
this.channelName = value
}
/**
* @param value The Amazon Resource Name (ARN) of an IAM role that grants the AWS Support App access to perform operations for AWS services.
*/
@JvmName("ojteqysofipjwniu")
public suspend fun channelRoleArn(`value`: Output) {
this.channelRoleArn = value
}
/**
* @param value Whether to notify when a correspondence is added to a case.
*/
@JvmName("lpqkwhbunpxoffst")
public suspend fun notifyOnAddCorrespondenceToCase(`value`: Output) {
this.notifyOnAddCorrespondenceToCase = value
}
/**
* @param value The severity level of a support case that a customer wants to get notified for.
*/
@JvmName("oeilyvdvjjdfufrt")
public suspend fun notifyOnCaseSeverity(`value`: Output) {
this.notifyOnCaseSeverity = value
}
/**
* @param value Whether to notify when a case is created or reopened.
*/
@JvmName("brosqsxsilenrefc")
public suspend fun notifyOnCreateOrReopenCase(`value`: Output) {
this.notifyOnCreateOrReopenCase = value
}
/**
* @param value Whether to notify when a case is resolved.
*/
@JvmName("gwyfoctjjnwprmum")
public suspend fun notifyOnResolveCase(`value`: Output) {
this.notifyOnResolveCase = value
}
/**
* @param value The team ID in Slack, which uniquely identifies a workspace.
*/
@JvmName("mahjsmdpdhvntnnq")
public suspend fun teamId(`value`: Output) {
this.teamId = value
}
/**
* @param value The channel ID in Slack, which identifies a channel within a workspace.
*/
@JvmName("pbkjdpccgfoudbnc")
public suspend fun channelId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelId = mapped
}
/**
* @param value The channel name in Slack.
*/
@JvmName("cbgvyraodeuxsbfb")
public suspend fun channelName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelName = mapped
}
/**
* @param value The Amazon Resource Name (ARN) of an IAM role that grants the AWS Support App access to perform operations for AWS services.
*/
@JvmName("prbfdkkqdrdiwdps")
public suspend fun channelRoleArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.channelRoleArn = mapped
}
/**
* @param value Whether to notify when a correspondence is added to a case.
*/
@JvmName("jxfuuuspqinsuhrj")
public suspend fun notifyOnAddCorrespondenceToCase(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notifyOnAddCorrespondenceToCase = mapped
}
/**
* @param value The severity level of a support case that a customer wants to get notified for.
*/
@JvmName("fdsjahgngetjaxji")
public suspend fun notifyOnCaseSeverity(`value`: SlackChannelConfigurationNotifyOnCaseSeverity?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notifyOnCaseSeverity = mapped
}
/**
* @param value Whether to notify when a case is created or reopened.
*/
@JvmName("phkvdlxvqopmvfpn")
public suspend fun notifyOnCreateOrReopenCase(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notifyOnCreateOrReopenCase = mapped
}
/**
* @param value Whether to notify when a case is resolved.
*/
@JvmName("cpqlylpgohdplohn")
public suspend fun notifyOnResolveCase(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.notifyOnResolveCase = mapped
}
/**
* @param value The team ID in Slack, which uniquely identifies a workspace.
*/
@JvmName("olcaqdnyytobymtl")
public suspend fun teamId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.teamId = mapped
}
internal fun build(): SlackChannelConfigurationArgs = SlackChannelConfigurationArgs(
channelId = channelId,
channelName = channelName,
channelRoleArn = channelRoleArn,
notifyOnAddCorrespondenceToCase = notifyOnAddCorrespondenceToCase,
notifyOnCaseSeverity = notifyOnCaseSeverity,
notifyOnCreateOrReopenCase = notifyOnCreateOrReopenCase,
notifyOnResolveCase = notifyOnResolveCase,
teamId = teamId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy