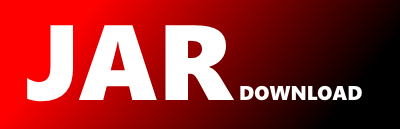
com.pulumi.awsnative.synthetics.kotlin.inputs.CanaryCodeArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.synthetics.kotlin.inputs
import com.pulumi.awsnative.synthetics.inputs.CanaryCodeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property handler The entry point to use for the source code when running the canary. For canaries that use the `syn-python-selenium-1.0` runtime or a `syn-nodejs.puppeteer` runtime earlier than `syn-nodejs.puppeteer-3.4` , the handler must be specified as `*fileName* .handler` . For `syn-python-selenium-1.1` , `syn-nodejs.puppeteer-3.4` , and later runtimes, the handler can be specified as `*fileName* . *functionName*` , or you can specify a folder where canary scripts reside as `*folder* / *fileName* . *functionName*` .
* @property s3Bucket If your canary script is located in S3, specify the bucket name here. The bucket must already exist.
* @property s3Key The S3 key of your script. For more information, see [Working with Amazon S3 Objects](https://docs.aws.amazon.com/AmazonS3/latest/dev/UsingObjects.html) .
* @property s3ObjectVersion The S3 version ID of your script.
* @property script If you input your canary script directly into the canary instead of referring to an S3 location, the value of this parameter is the script in plain text. It can be up to 5 MB.
* @property sourceLocationArn The ARN of the Lambda layer where Synthetics stores the canary script code.
*/
public data class CanaryCodeArgs(
public val handler: Output,
public val s3Bucket: Output? = null,
public val s3Key: Output? = null,
public val s3ObjectVersion: Output? = null,
public val script: Output? = null,
public val sourceLocationArn: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.synthetics.inputs.CanaryCodeArgs =
com.pulumi.awsnative.synthetics.inputs.CanaryCodeArgs.builder()
.handler(handler.applyValue({ args0 -> args0 }))
.s3Bucket(s3Bucket?.applyValue({ args0 -> args0 }))
.s3Key(s3Key?.applyValue({ args0 -> args0 }))
.s3ObjectVersion(s3ObjectVersion?.applyValue({ args0 -> args0 }))
.script(script?.applyValue({ args0 -> args0 }))
.sourceLocationArn(sourceLocationArn?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [CanaryCodeArgs].
*/
@PulumiTagMarker
public class CanaryCodeArgsBuilder internal constructor() {
private var handler: Output? = null
private var s3Bucket: Output? = null
private var s3Key: Output? = null
private var s3ObjectVersion: Output? = null
private var script: Output? = null
private var sourceLocationArn: Output? = null
/**
* @param value The entry point to use for the source code when running the canary. For canaries that use the `syn-python-selenium-1.0` runtime or a `syn-nodejs.puppeteer` runtime earlier than `syn-nodejs.puppeteer-3.4` , the handler must be specified as `*fileName* .handler` . For `syn-python-selenium-1.1` , `syn-nodejs.puppeteer-3.4` , and later runtimes, the handler can be specified as `*fileName* . *functionName*` , or you can specify a folder where canary scripts reside as `*folder* / *fileName* . *functionName*` .
*/
@JvmName("yuviecahskjcffei")
public suspend fun handler(`value`: Output) {
this.handler = value
}
/**
* @param value If your canary script is located in S3, specify the bucket name here. The bucket must already exist.
*/
@JvmName("grqaophdaloafqoc")
public suspend fun s3Bucket(`value`: Output) {
this.s3Bucket = value
}
/**
* @param value The S3 key of your script. For more information, see [Working with Amazon S3 Objects](https://docs.aws.amazon.com/AmazonS3/latest/dev/UsingObjects.html) .
*/
@JvmName("huboxhoujccpxigf")
public suspend fun s3Key(`value`: Output) {
this.s3Key = value
}
/**
* @param value The S3 version ID of your script.
*/
@JvmName("cvmxuucthuyvtrqc")
public suspend fun s3ObjectVersion(`value`: Output) {
this.s3ObjectVersion = value
}
/**
* @param value If you input your canary script directly into the canary instead of referring to an S3 location, the value of this parameter is the script in plain text. It can be up to 5 MB.
*/
@JvmName("cidmopjybhoxkddd")
public suspend fun script(`value`: Output) {
this.script = value
}
/**
* @param value The ARN of the Lambda layer where Synthetics stores the canary script code.
*/
@JvmName("lgkodlnwpqhrpfme")
public suspend fun sourceLocationArn(`value`: Output) {
this.sourceLocationArn = value
}
/**
* @param value The entry point to use for the source code when running the canary. For canaries that use the `syn-python-selenium-1.0` runtime or a `syn-nodejs.puppeteer` runtime earlier than `syn-nodejs.puppeteer-3.4` , the handler must be specified as `*fileName* .handler` . For `syn-python-selenium-1.1` , `syn-nodejs.puppeteer-3.4` , and later runtimes, the handler can be specified as `*fileName* . *functionName*` , or you can specify a folder where canary scripts reside as `*folder* / *fileName* . *functionName*` .
*/
@JvmName("dapwqwrdlcoggnnb")
public suspend fun handler(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.handler = mapped
}
/**
* @param value If your canary script is located in S3, specify the bucket name here. The bucket must already exist.
*/
@JvmName("pjasddobldjosdly")
public suspend fun s3Bucket(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3Bucket = mapped
}
/**
* @param value The S3 key of your script. For more information, see [Working with Amazon S3 Objects](https://docs.aws.amazon.com/AmazonS3/latest/dev/UsingObjects.html) .
*/
@JvmName("rkxwqvummcvgrqgy")
public suspend fun s3Key(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3Key = mapped
}
/**
* @param value The S3 version ID of your script.
*/
@JvmName("uspnfncsphftkowu")
public suspend fun s3ObjectVersion(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.s3ObjectVersion = mapped
}
/**
* @param value If you input your canary script directly into the canary instead of referring to an S3 location, the value of this parameter is the script in plain text. It can be up to 5 MB.
*/
@JvmName("biarxrxcwefufbce")
public suspend fun script(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.script = mapped
}
/**
* @param value The ARN of the Lambda layer where Synthetics stores the canary script code.
*/
@JvmName("lyhmqybgntohilfi")
public suspend fun sourceLocationArn(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceLocationArn = mapped
}
internal fun build(): CanaryCodeArgs = CanaryCodeArgs(
handler = handler ?: throw PulumiNullFieldException("handler"),
s3Bucket = s3Bucket,
s3Key = s3Key,
s3ObjectVersion = s3ObjectVersion,
script = script,
sourceLocationArn = sourceLocationArn,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy