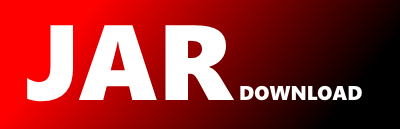
com.pulumi.awsnative.timestream.kotlin.InfluxDbInstance.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.timestream.kotlin
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceDbInstanceType
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceDbStorageType
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceDeploymentType
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceStatus
import com.pulumi.awsnative.timestream.kotlin.outputs.LogDeliveryConfigurationProperties
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin as tagToKotlin
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceDbInstanceType.Companion.toKotlin as influxDbInstanceDbInstanceTypeToKotlin
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceDbStorageType.Companion.toKotlin as influxDbInstanceDbStorageTypeToKotlin
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceDeploymentType.Companion.toKotlin as influxDbInstanceDeploymentTypeToKotlin
import com.pulumi.awsnative.timestream.kotlin.enums.InfluxDbInstanceStatus.Companion.toKotlin as influxDbInstanceStatusToKotlin
import com.pulumi.awsnative.timestream.kotlin.outputs.LogDeliveryConfigurationProperties.Companion.toKotlin as logDeliveryConfigurationPropertiesToKotlin
/**
* Builder for [InfluxDbInstance].
*/
@PulumiTagMarker
public class InfluxDbInstanceResourceBuilder internal constructor() {
public var name: String? = null
public var args: InfluxDbInstanceArgs = InfluxDbInstanceArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend InfluxDbInstanceArgsBuilder.() -> Unit) {
val builder = InfluxDbInstanceArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): InfluxDbInstance {
val builtJavaResource =
com.pulumi.awsnative.timestream.InfluxDbInstance(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return InfluxDbInstance(builtJavaResource)
}
}
/**
* The AWS::Timestream::InfluxDBInstance resource creates an InfluxDB instance.
*/
public class InfluxDbInstance internal constructor(
override val javaResource: com.pulumi.awsnative.timestream.InfluxDbInstance,
) : KotlinCustomResource(javaResource, InfluxDbInstanceMapper) {
/**
* The allocated storage for the InfluxDB instance.
*/
public val allocatedStorage: Output?
get() = javaResource.allocatedStorage().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Amazon Resource Name (ARN) that is associated with the InfluxDB instance.
*/
public val arn: Output
get() = javaResource.arn().applyValue({ args0 -> args0 })
/**
* The Availability Zone (AZ) where the InfluxDB instance is created.
*/
public val availabilityZone: Output
get() = javaResource.availabilityZone().applyValue({ args0 -> args0 })
/**
* The service generated unique identifier for InfluxDB instance.
*/
public val awsId: Output
get() = javaResource.awsId().applyValue({ args0 -> args0 })
/**
* The bucket for the InfluxDB instance.
*/
public val bucket: Output?
get() = javaResource.bucket().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The compute instance of the InfluxDB instance.
*/
public val dbInstanceType: Output?
get() = javaResource.dbInstanceType().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> influxDbInstanceDbInstanceTypeToKotlin(args0) })
}).orElse(null)
})
/**
* The name of an existing InfluxDB parameter group.
*/
public val dbParameterGroupIdentifier: Output?
get() = javaResource.dbParameterGroupIdentifier().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The storage type of the InfluxDB instance.
*/
public val dbStorageType: Output?
get() = javaResource.dbStorageType().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> influxDbInstanceDbStorageTypeToKotlin(args0) })
}).orElse(null)
})
/**
* Deployment type of the InfluxDB Instance.
*/
public val deploymentType: Output?
get() = javaResource.deploymentType().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> influxDbInstanceDeploymentTypeToKotlin(args0) })
}).orElse(null)
})
/**
* The connection endpoint for the InfluxDB instance.
*/
public val endpoint: Output
get() = javaResource.endpoint().applyValue({ args0 -> args0 })
/**
* The Auth parameters secret Amazon Resource name (ARN) that is associated with the InfluxDB instance.
*/
public val influxAuthParametersSecretArn: Output
get() = javaResource.influxAuthParametersSecretArn().applyValue({ args0 -> args0 })
/**
* Configuration for sending logs to customer account from the InfluxDB instance.
*/
public val logDeliveryConfiguration: Output?
get() = javaResource.logDeliveryConfiguration().applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> logDeliveryConfigurationPropertiesToKotlin(args0) })
}).orElse(null)
})
/**
* The unique name that is associated with the InfluxDB instance.
*/
public val name: Output?
get() = javaResource.name().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The organization for the InfluxDB instance.
*/
public val organization: Output?
get() = javaResource.organization().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The password for the InfluxDB instance.
*/
public val password: Output?
get() = javaResource.password().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* Attach a public IP to the customer ENI.
*/
public val publiclyAccessible: Output?
get() = javaResource.publiclyAccessible().applyValue({ args0 ->
args0.map({ args0 ->
args0
}).orElse(null)
})
/**
* The Secondary Availability Zone (AZ) where the InfluxDB instance is created, if DeploymentType is set as WITH_MULTIAZ_STANDBY.
*/
public val secondaryAvailabilityZone: Output
get() = javaResource.secondaryAvailabilityZone().applyValue({ args0 -> args0 })
/**
* Status of the InfluxDB Instance.
*/
public val status: Output
get() = javaResource.status().applyValue({ args0 ->
args0.let({ args0 ->
influxDbInstanceStatusToKotlin(args0)
})
})
/**
* An arbitrary set of tags (key-value pairs) for this DB instance.
*/
public val tags: Output>?
get() = javaResource.tags().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0.let({ args0 -> tagToKotlin(args0) })
})
}).orElse(null)
})
/**
* The username for the InfluxDB instance.
*/
public val username: Output?
get() = javaResource.username().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* A list of Amazon EC2 VPC security groups to associate with this InfluxDB instance.
*/
public val vpcSecurityGroupIds: Output>?
get() = javaResource.vpcSecurityGroupIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 -> args0 })
}).orElse(null)
})
/**
* A list of EC2 subnet IDs for this InfluxDB instance.
*/
public val vpcSubnetIds: Output>?
get() = javaResource.vpcSubnetIds().applyValue({ args0 ->
args0.map({ args0 ->
args0.map({ args0 ->
args0
})
}).orElse(null)
})
}
public object InfluxDbInstanceMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.awsnative.timestream.InfluxDbInstance::class == javaResource::class
override fun map(javaResource: Resource): InfluxDbInstance = InfluxDbInstance(
javaResource as
com.pulumi.awsnative.timestream.InfluxDbInstance,
)
}
/**
* @see [InfluxDbInstance].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [InfluxDbInstance].
*/
public suspend fun influxDbInstance(
name: String,
block: suspend InfluxDbInstanceResourceBuilder.() -> Unit,
): InfluxDbInstance {
val builder = InfluxDbInstanceResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [InfluxDbInstance].
* @param name The _unique_ name of the resulting resource.
*/
public fun influxDbInstance(name: String): InfluxDbInstance {
val builder = InfluxDbInstanceResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy