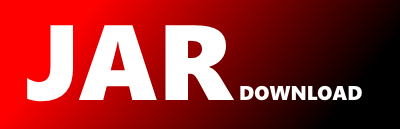
com.pulumi.awsnative.transfer.kotlin.inputs.WorkflowStepDecryptStepDetailsPropertiesArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.transfer.kotlin.inputs
import com.pulumi.awsnative.transfer.inputs.WorkflowStepDecryptStepDetailsPropertiesArgs.builder
import com.pulumi.awsnative.transfer.kotlin.enums.WorkflowStepDecryptStepDetailsPropertiesOverwriteExisting
import com.pulumi.awsnative.transfer.kotlin.enums.WorkflowStepDecryptStepDetailsPropertiesType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Details for a step that performs a file decryption.
* @property destinationFileLocation
* @property name The name of the step, used as an identifier.
* @property overwriteExisting A flag that indicates whether or not to overwrite an existing file of the same name. The default is FALSE.
* @property sourceFileLocation Specifies which file to use as input to the workflow step.
* @property type Specifies which encryption method to use.
*/
public data class WorkflowStepDecryptStepDetailsPropertiesArgs(
public val destinationFileLocation: Output,
public val name: Output? = null,
public val overwriteExisting: Output? =
null,
public val sourceFileLocation: Output? = null,
public val type: Output,
) :
ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.transfer.inputs.WorkflowStepDecryptStepDetailsPropertiesArgs =
com.pulumi.awsnative.transfer.inputs.WorkflowStepDecryptStepDetailsPropertiesArgs.builder()
.destinationFileLocation(
destinationFileLocation.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.overwriteExisting(overwriteExisting?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.sourceFileLocation(sourceFileLocation?.applyValue({ args0 -> args0 }))
.type(type.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [WorkflowStepDecryptStepDetailsPropertiesArgs].
*/
@PulumiTagMarker
public class WorkflowStepDecryptStepDetailsPropertiesArgsBuilder internal constructor() {
private var destinationFileLocation: Output? = null
private var name: Output? = null
private var overwriteExisting: Output? =
null
private var sourceFileLocation: Output? = null
private var type: Output? = null
/**
* @param value
*/
@JvmName("jdtjugxojttujmto")
public suspend fun destinationFileLocation(`value`: Output) {
this.destinationFileLocation = value
}
/**
* @param value The name of the step, used as an identifier.
*/
@JvmName("gyoyirasibymkmee")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value A flag that indicates whether or not to overwrite an existing file of the same name. The default is FALSE.
*/
@JvmName("jiguqelhqbmrswey")
public suspend fun overwriteExisting(`value`: Output) {
this.overwriteExisting = value
}
/**
* @param value Specifies which file to use as input to the workflow step.
*/
@JvmName("yjwlmwffxrocvpco")
public suspend fun sourceFileLocation(`value`: Output) {
this.sourceFileLocation = value
}
/**
* @param value Specifies which encryption method to use.
*/
@JvmName("msdvsdgvurxodajc")
public suspend fun type(`value`: Output) {
this.type = value
}
/**
* @param value
*/
@JvmName("hlaulonfgxfhqrxg")
public suspend fun destinationFileLocation(`value`: WorkflowInputFileLocationArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.destinationFileLocation = mapped
}
/**
* @param argument
*/
@JvmName("gembvabcnvbqioki")
public suspend fun destinationFileLocation(argument: suspend WorkflowInputFileLocationArgsBuilder.() -> Unit) {
val toBeMapped = WorkflowInputFileLocationArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.destinationFileLocation = mapped
}
/**
* @param value The name of the step, used as an identifier.
*/
@JvmName("ekyyobfdgkmbxdia")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value A flag that indicates whether or not to overwrite an existing file of the same name. The default is FALSE.
*/
@JvmName("ixdvwtccfltksnel")
public suspend fun overwriteExisting(`value`: WorkflowStepDecryptStepDetailsPropertiesOverwriteExisting?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.overwriteExisting = mapped
}
/**
* @param value Specifies which file to use as input to the workflow step.
*/
@JvmName("bqhsbtdupykymtnt")
public suspend fun sourceFileLocation(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sourceFileLocation = mapped
}
/**
* @param value Specifies which encryption method to use.
*/
@JvmName("pacrjcsmhesmfigu")
public suspend fun type(`value`: WorkflowStepDecryptStepDetailsPropertiesType) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.type = mapped
}
internal fun build(): WorkflowStepDecryptStepDetailsPropertiesArgs =
WorkflowStepDecryptStepDetailsPropertiesArgs(
destinationFileLocation = destinationFileLocation ?: throw
PulumiNullFieldException("destinationFileLocation"),
name = name,
overwriteExisting = overwriteExisting,
sourceFileLocation = sourceFileLocation,
type = type ?: throw PulumiNullFieldException("type"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy