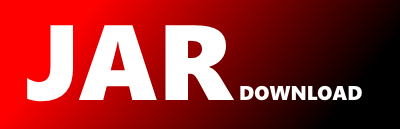
com.pulumi.awsnative.wafv2.kotlin.inputs.WebAclRequestInspectionArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.wafv2.kotlin.inputs
import com.pulumi.awsnative.wafv2.inputs.WebAclRequestInspectionArgs.builder
import com.pulumi.awsnative.wafv2.kotlin.enums.WebAclRequestInspectionPayloadType
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Configures the inspection of login requests
* @property passwordField The name of the field in the request payload that contains your customer's password.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "password": "THE_PASSWORD" } }` , the password field specification is `/form/password` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `password1` , the password field specification is `password1` .
* @property payloadType The payload type for your login endpoint, either JSON or form encoded.
* @property usernameField The name of the field in the request payload that contains your customer's username.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "username": "THE_USERNAME" } }` , the username field specification is `/form/username` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `username1` , the username field specification is `username1`
*/
public data class WebAclRequestInspectionArgs(
public val passwordField: Output,
public val payloadType: Output,
public val usernameField: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.awsnative.wafv2.inputs.WebAclRequestInspectionArgs =
com.pulumi.awsnative.wafv2.inputs.WebAclRequestInspectionArgs.builder()
.passwordField(passwordField.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.payloadType(payloadType.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.usernameField(usernameField.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [WebAclRequestInspectionArgs].
*/
@PulumiTagMarker
public class WebAclRequestInspectionArgsBuilder internal constructor() {
private var passwordField: Output? = null
private var payloadType: Output? = null
private var usernameField: Output? = null
/**
* @param value The name of the field in the request payload that contains your customer's password.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "password": "THE_PASSWORD" } }` , the password field specification is `/form/password` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `password1` , the password field specification is `password1` .
*/
@JvmName("ximvnofptrrecqct")
public suspend fun passwordField(`value`: Output) {
this.passwordField = value
}
/**
* @param value The payload type for your login endpoint, either JSON or form encoded.
*/
@JvmName("uybsamacgsxyihkj")
public suspend fun payloadType(`value`: Output) {
this.payloadType = value
}
/**
* @param value The name of the field in the request payload that contains your customer's username.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "username": "THE_USERNAME" } }` , the username field specification is `/form/username` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `username1` , the username field specification is `username1`
*/
@JvmName("jqerqxoivprudeva")
public suspend fun usernameField(`value`: Output) {
this.usernameField = value
}
/**
* @param value The name of the field in the request payload that contains your customer's password.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "password": "THE_PASSWORD" } }` , the password field specification is `/form/password` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `password1` , the password field specification is `password1` .
*/
@JvmName("qvwmuyjfowwnmyvi")
public suspend fun passwordField(`value`: WebAclFieldIdentifierArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.passwordField = mapped
}
/**
* @param argument The name of the field in the request payload that contains your customer's password.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "password": "THE_PASSWORD" } }` , the password field specification is `/form/password` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `password1` , the password field specification is `password1` .
*/
@JvmName("cnmgrysfossjgphi")
public suspend fun passwordField(argument: suspend WebAclFieldIdentifierArgsBuilder.() -> Unit) {
val toBeMapped = WebAclFieldIdentifierArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.passwordField = mapped
}
/**
* @param value The payload type for your login endpoint, either JSON or form encoded.
*/
@JvmName("ytvorwkpgojrawqd")
public suspend fun payloadType(`value`: WebAclRequestInspectionPayloadType) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.payloadType = mapped
}
/**
* @param value The name of the field in the request payload that contains your customer's username.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "username": "THE_USERNAME" } }` , the username field specification is `/form/username` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `username1` , the username field specification is `username1`
*/
@JvmName("nsdolpycxamyjalj")
public suspend fun usernameField(`value`: WebAclFieldIdentifierArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.usernameField = mapped
}
/**
* @param argument The name of the field in the request payload that contains your customer's username.
* How you specify this depends on the request inspection payload type.
* - For JSON payloads, specify the field name in JSON pointer syntax. For information about the JSON Pointer syntax, see the Internet Engineering Task Force (IETF) documentation [JavaScript Object Notation (JSON) Pointer](https://docs.aws.amazon.com/https://tools.ietf.org/html/rfc6901) .
* For example, for the JSON payload `{ "form": { "username": "THE_USERNAME" } }` , the username field specification is `/form/username` .
* - For form encoded payload types, use the HTML form names.
* For example, for an HTML form with the input element named `username1` , the username field specification is `username1`
*/
@JvmName("midqichkjagmngpl")
public suspend fun usernameField(argument: suspend WebAclFieldIdentifierArgsBuilder.() -> Unit) {
val toBeMapped = WebAclFieldIdentifierArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.usernameField = mapped
}
internal fun build(): WebAclRequestInspectionArgs = WebAclRequestInspectionArgs(
passwordField = passwordField ?: throw PulumiNullFieldException("passwordField"),
payloadType = payloadType ?: throw PulumiNullFieldException("payloadType"),
usernameField = usernameField ?: throw PulumiNullFieldException("usernameField"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy