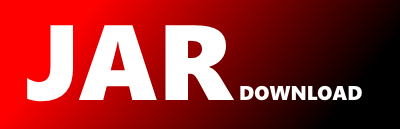
com.pulumi.awsnative.workspacesweb.kotlin.outputs.GetUserSettingsResult.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.awsnative.workspacesweb.kotlin.outputs
import com.pulumi.awsnative.kotlin.outputs.Tag
import com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property associatedPortalArns A list of web portal ARNs that this user settings resource is associated with.
* @property cookieSynchronizationConfiguration The configuration that specifies which cookies should be synchronized from the end user's local browser to the remote browser.
* @property copyAllowed Specifies whether the user can copy text from the streaming session to the local device.
* @property deepLinkAllowed Specifies whether the user can use deep links that open automatically when connecting to a session.
* @property disconnectTimeoutInMinutes The amount of time that a streaming session remains active after users disconnect.
* @property downloadAllowed Specifies whether the user can download files from the streaming session to the local device.
* @property idleDisconnectTimeoutInMinutes The amount of time that users can be idle (inactive) before they are disconnected from their streaming session and the disconnect timeout interval begins.
* @property pasteAllowed Specifies whether the user can paste text from the local device to the streaming session.
* @property printAllowed Specifies whether the user can print to the local device.
* @property tags The tags to add to the user settings resource. A tag is a key-value pair.
* @property uploadAllowed Specifies whether the user can upload files from the local device to the streaming session.
* @property userSettingsArn The ARN of the user settings.
*/
public data class GetUserSettingsResult(
public val associatedPortalArns: List? = null,
public val cookieSynchronizationConfiguration: UserSettingsCookieSynchronizationConfiguration? =
null,
public val copyAllowed: UserSettingsEnabledType? = null,
public val deepLinkAllowed: UserSettingsEnabledType? = null,
public val disconnectTimeoutInMinutes: Double? = null,
public val downloadAllowed: UserSettingsEnabledType? = null,
public val idleDisconnectTimeoutInMinutes: Double? = null,
public val pasteAllowed: UserSettingsEnabledType? = null,
public val printAllowed: UserSettingsEnabledType? = null,
public val tags: List? = null,
public val uploadAllowed: UserSettingsEnabledType? = null,
public val userSettingsArn: String? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.awsnative.workspacesweb.outputs.GetUserSettingsResult): GetUserSettingsResult = GetUserSettingsResult(
associatedPortalArns = javaType.associatedPortalArns().map({ args0 -> args0 }),
cookieSynchronizationConfiguration = javaType.cookieSynchronizationConfiguration().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.outputs.UserSettingsCookieSynchronizationConfiguration.Companion.toKotlin(args0)
})
}).orElse(null),
copyAllowed = javaType.copyAllowed().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType.Companion.toKotlin(args0)
})
}).orElse(null),
deepLinkAllowed = javaType.deepLinkAllowed().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType.Companion.toKotlin(args0)
})
}).orElse(null),
disconnectTimeoutInMinutes = javaType.disconnectTimeoutInMinutes().map({ args0 ->
args0
}).orElse(null),
downloadAllowed = javaType.downloadAllowed().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType.Companion.toKotlin(args0)
})
}).orElse(null),
idleDisconnectTimeoutInMinutes = javaType.idleDisconnectTimeoutInMinutes().map({ args0 ->
args0
}).orElse(null),
pasteAllowed = javaType.pasteAllowed().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType.Companion.toKotlin(args0)
})
}).orElse(null),
printAllowed = javaType.printAllowed().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType.Companion.toKotlin(args0)
})
}).orElse(null),
tags = javaType.tags().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.kotlin.outputs.Tag.Companion.toKotlin(args0)
})
}),
uploadAllowed = javaType.uploadAllowed().map({ args0 ->
args0.let({ args0 ->
com.pulumi.awsnative.workspacesweb.kotlin.enums.UserSettingsEnabledType.Companion.toKotlin(args0)
})
}).orElse(null),
userSettingsArn = javaType.userSettingsArn().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy