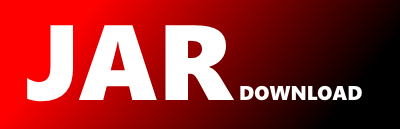
com.pulumi.azure.apimanagement.kotlin.DiagnosticArgs.kt Maven / Gradle / Ivy
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin
import com.pulumi.azure.apimanagement.DiagnosticArgs.builder
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticBackendRequestArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticBackendRequestArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticBackendResponseArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticBackendResponseArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticFrontendRequestArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticFrontendRequestArgsBuilder
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticFrontendResponseArgs
import com.pulumi.azure.apimanagement.kotlin.inputs.DiagnosticFrontendResponseArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Double
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages an API Management Service Diagnostic.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleInsights = new azure.appinsights.Insights("example", {
* name: "example-appinsights",
* location: example.location,
* resourceGroupName: example.name,
* applicationType: "web",
* });
* const exampleService = new azure.apimanagement.Service("example", {
* name: "example-apim",
* location: example.location,
* resourceGroupName: example.name,
* publisherName: "My Company",
* publisherEmail: "[email protected]",
* skuName: "Developer_1",
* });
* const exampleLogger = new azure.apimanagement.Logger("example", {
* name: "example-apimlogger",
* apiManagementName: exampleService.name,
* resourceGroupName: example.name,
* applicationInsights: {
* instrumentationKey: exampleInsights.instrumentationKey,
* },
* });
* const exampleDiagnostic = new azure.apimanagement.Diagnostic("example", {
* identifier: "applicationinsights",
* resourceGroupName: example.name,
* apiManagementName: exampleService.name,
* apiManagementLoggerId: exampleLogger.id,
* samplingPercentage: 5,
* alwaysLogErrors: true,
* logClientIp: true,
* verbosity: "verbose",
* httpCorrelationProtocol: "W3C",
* frontendRequest: {
* bodyBytes: 32,
* headersToLogs: [
* "content-type",
* "accept",
* "origin",
* ],
* },
* frontendResponse: {
* bodyBytes: 32,
* headersToLogs: [
* "content-type",
* "content-length",
* "origin",
* ],
* },
* backendRequest: {
* bodyBytes: 32,
* headersToLogs: [
* "content-type",
* "accept",
* "origin",
* ],
* },
* backendResponse: {
* bodyBytes: 32,
* headersToLogs: [
* "content-type",
* "content-length",
* "origin",
* ],
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_insights = azure.appinsights.Insights("example",
* name="example-appinsights",
* location=example.location,
* resource_group_name=example.name,
* application_type="web")
* example_service = azure.apimanagement.Service("example",
* name="example-apim",
* location=example.location,
* resource_group_name=example.name,
* publisher_name="My Company",
* publisher_email="[email protected]",
* sku_name="Developer_1")
* example_logger = azure.apimanagement.Logger("example",
* name="example-apimlogger",
* api_management_name=example_service.name,
* resource_group_name=example.name,
* application_insights=azure.apimanagement.LoggerApplicationInsightsArgs(
* instrumentation_key=example_insights.instrumentation_key,
* ))
* example_diagnostic = azure.apimanagement.Diagnostic("example",
* identifier="applicationinsights",
* resource_group_name=example.name,
* api_management_name=example_service.name,
* api_management_logger_id=example_logger.id,
* sampling_percentage=5,
* always_log_errors=True,
* log_client_ip=True,
* verbosity="verbose",
* http_correlation_protocol="W3C",
* frontend_request=azure.apimanagement.DiagnosticFrontendRequestArgs(
* body_bytes=32,
* headers_to_logs=[
* "content-type",
* "accept",
* "origin",
* ],
* ),
* frontend_response=azure.apimanagement.DiagnosticFrontendResponseArgs(
* body_bytes=32,
* headers_to_logs=[
* "content-type",
* "content-length",
* "origin",
* ],
* ),
* backend_request=azure.apimanagement.DiagnosticBackendRequestArgs(
* body_bytes=32,
* headers_to_logs=[
* "content-type",
* "accept",
* "origin",
* ],
* ),
* backend_response=azure.apimanagement.DiagnosticBackendResponseArgs(
* body_bytes=32,
* headers_to_logs=[
* "content-type",
* "content-length",
* "origin",
* ],
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleInsights = new Azure.AppInsights.Insights("example", new()
* {
* Name = "example-appinsights",
* Location = example.Location,
* ResourceGroupName = example.Name,
* ApplicationType = "web",
* });
* var exampleService = new Azure.ApiManagement.Service("example", new()
* {
* Name = "example-apim",
* Location = example.Location,
* ResourceGroupName = example.Name,
* PublisherName = "My Company",
* PublisherEmail = "[email protected]",
* SkuName = "Developer_1",
* });
* var exampleLogger = new Azure.ApiManagement.Logger("example", new()
* {
* Name = "example-apimlogger",
* ApiManagementName = exampleService.Name,
* ResourceGroupName = example.Name,
* ApplicationInsights = new Azure.ApiManagement.Inputs.LoggerApplicationInsightsArgs
* {
* InstrumentationKey = exampleInsights.InstrumentationKey,
* },
* });
* var exampleDiagnostic = new Azure.ApiManagement.Diagnostic("example", new()
* {
* Identifier = "applicationinsights",
* ResourceGroupName = example.Name,
* ApiManagementName = exampleService.Name,
* ApiManagementLoggerId = exampleLogger.Id,
* SamplingPercentage = 5,
* AlwaysLogErrors = true,
* LogClientIp = true,
* Verbosity = "verbose",
* HttpCorrelationProtocol = "W3C",
* FrontendRequest = new Azure.ApiManagement.Inputs.DiagnosticFrontendRequestArgs
* {
* BodyBytes = 32,
* HeadersToLogs = new[]
* {
* "content-type",
* "accept",
* "origin",
* },
* },
* FrontendResponse = new Azure.ApiManagement.Inputs.DiagnosticFrontendResponseArgs
* {
* BodyBytes = 32,
* HeadersToLogs = new[]
* {
* "content-type",
* "content-length",
* "origin",
* },
* },
* BackendRequest = new Azure.ApiManagement.Inputs.DiagnosticBackendRequestArgs
* {
* BodyBytes = 32,
* HeadersToLogs = new[]
* {
* "content-type",
* "accept",
* "origin",
* },
* },
* BackendResponse = new Azure.ApiManagement.Inputs.DiagnosticBackendResponseArgs
* {
* BodyBytes = 32,
* HeadersToLogs = new[]
* {
* "content-type",
* "content-length",
* "origin",
* },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/appinsights"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleInsights, err := appinsights.NewInsights(ctx, "example", &appinsights.InsightsArgs{
* Name: pulumi.String("example-appinsights"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* ApplicationType: pulumi.String("web"),
* })
* if err != nil {
* return err
* }
* exampleService, err := apimanagement.NewService(ctx, "example", &apimanagement.ServiceArgs{
* Name: pulumi.String("example-apim"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* PublisherName: pulumi.String("My Company"),
* PublisherEmail: pulumi.String("[email protected]"),
* SkuName: pulumi.String("Developer_1"),
* })
* if err != nil {
* return err
* }
* exampleLogger, err := apimanagement.NewLogger(ctx, "example", &apimanagement.LoggerArgs{
* Name: pulumi.String("example-apimlogger"),
* ApiManagementName: exampleService.Name,
* ResourceGroupName: example.Name,
* ApplicationInsights: &apimanagement.LoggerApplicationInsightsArgs{
* InstrumentationKey: exampleInsights.InstrumentationKey,
* },
* })
* if err != nil {
* return err
* }
* _, err = apimanagement.NewDiagnostic(ctx, "example", &apimanagement.DiagnosticArgs{
* Identifier: pulumi.String("applicationinsights"),
* ResourceGroupName: example.Name,
* ApiManagementName: exampleService.Name,
* ApiManagementLoggerId: exampleLogger.ID(),
* SamplingPercentage: pulumi.Float64(5),
* AlwaysLogErrors: pulumi.Bool(true),
* LogClientIp: pulumi.Bool(true),
* Verbosity: pulumi.String("verbose"),
* HttpCorrelationProtocol: pulumi.String("W3C"),
* FrontendRequest: &apimanagement.DiagnosticFrontendRequestArgs{
* BodyBytes: pulumi.Int(32),
* HeadersToLogs: pulumi.StringArray{
* pulumi.String("content-type"),
* pulumi.String("accept"),
* pulumi.String("origin"),
* },
* },
* FrontendResponse: &apimanagement.DiagnosticFrontendResponseArgs{
* BodyBytes: pulumi.Int(32),
* HeadersToLogs: pulumi.StringArray{
* pulumi.String("content-type"),
* pulumi.String("content-length"),
* pulumi.String("origin"),
* },
* },
* BackendRequest: &apimanagement.DiagnosticBackendRequestArgs{
* BodyBytes: pulumi.Int(32),
* HeadersToLogs: pulumi.StringArray{
* pulumi.String("content-type"),
* pulumi.String("accept"),
* pulumi.String("origin"),
* },
* },
* BackendResponse: &apimanagement.DiagnosticBackendResponseArgs{
* BodyBytes: pulumi.Int(32),
* HeadersToLogs: pulumi.StringArray{
* pulumi.String("content-type"),
* pulumi.String("content-length"),
* pulumi.String("origin"),
* },
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.appinsights.Insights;
* import com.pulumi.azure.appinsights.InsightsArgs;
* import com.pulumi.azure.apimanagement.Service;
* import com.pulumi.azure.apimanagement.ServiceArgs;
* import com.pulumi.azure.apimanagement.Logger;
* import com.pulumi.azure.apimanagement.LoggerArgs;
* import com.pulumi.azure.apimanagement.inputs.LoggerApplicationInsightsArgs;
* import com.pulumi.azure.apimanagement.Diagnostic;
* import com.pulumi.azure.apimanagement.DiagnosticArgs;
* import com.pulumi.azure.apimanagement.inputs.DiagnosticFrontendRequestArgs;
* import com.pulumi.azure.apimanagement.inputs.DiagnosticFrontendResponseArgs;
* import com.pulumi.azure.apimanagement.inputs.DiagnosticBackendRequestArgs;
* import com.pulumi.azure.apimanagement.inputs.DiagnosticBackendResponseArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleInsights = new Insights("exampleInsights", InsightsArgs.builder()
* .name("example-appinsights")
* .location(example.location())
* .resourceGroupName(example.name())
* .applicationType("web")
* .build());
* var exampleService = new Service("exampleService", ServiceArgs.builder()
* .name("example-apim")
* .location(example.location())
* .resourceGroupName(example.name())
* .publisherName("My Company")
* .publisherEmail("[email protected]")
* .skuName("Developer_1")
* .build());
* var exampleLogger = new Logger("exampleLogger", LoggerArgs.builder()
* .name("example-apimlogger")
* .apiManagementName(exampleService.name())
* .resourceGroupName(example.name())
* .applicationInsights(LoggerApplicationInsightsArgs.builder()
* .instrumentationKey(exampleInsights.instrumentationKey())
* .build())
* .build());
* var exampleDiagnostic = new Diagnostic("exampleDiagnostic", DiagnosticArgs.builder()
* .identifier("applicationinsights")
* .resourceGroupName(example.name())
* .apiManagementName(exampleService.name())
* .apiManagementLoggerId(exampleLogger.id())
* .samplingPercentage(5)
* .alwaysLogErrors(true)
* .logClientIp(true)
* .verbosity("verbose")
* .httpCorrelationProtocol("W3C")
* .frontendRequest(DiagnosticFrontendRequestArgs.builder()
* .bodyBytes(32)
* .headersToLogs(
* "content-type",
* "accept",
* "origin")
* .build())
* .frontendResponse(DiagnosticFrontendResponseArgs.builder()
* .bodyBytes(32)
* .headersToLogs(
* "content-type",
* "content-length",
* "origin")
* .build())
* .backendRequest(DiagnosticBackendRequestArgs.builder()
* .bodyBytes(32)
* .headersToLogs(
* "content-type",
* "accept",
* "origin")
* .build())
* .backendResponse(DiagnosticBackendResponseArgs.builder()
* .bodyBytes(32)
* .headersToLogs(
* "content-type",
* "content-length",
* "origin")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleInsights:
* type: azure:appinsights:Insights
* name: example
* properties:
* name: example-appinsights
* location: ${example.location}
* resourceGroupName: ${example.name}
* applicationType: web
* exampleService:
* type: azure:apimanagement:Service
* name: example
* properties:
* name: example-apim
* location: ${example.location}
* resourceGroupName: ${example.name}
* publisherName: My Company
* publisherEmail: [email protected]
* skuName: Developer_1
* exampleLogger:
* type: azure:apimanagement:Logger
* name: example
* properties:
* name: example-apimlogger
* apiManagementName: ${exampleService.name}
* resourceGroupName: ${example.name}
* applicationInsights:
* instrumentationKey: ${exampleInsights.instrumentationKey}
* exampleDiagnostic:
* type: azure:apimanagement:Diagnostic
* name: example
* properties:
* identifier: applicationinsights
* resourceGroupName: ${example.name}
* apiManagementName: ${exampleService.name}
* apiManagementLoggerId: ${exampleLogger.id}
* samplingPercentage: 5
* alwaysLogErrors: true
* logClientIp: true
* verbosity: verbose
* httpCorrelationProtocol: W3C
* frontendRequest:
* bodyBytes: 32
* headersToLogs:
* - content-type
* - accept
* - origin
* frontendResponse:
* bodyBytes: 32
* headersToLogs:
* - content-type
* - content-length
* - origin
* backendRequest:
* bodyBytes: 32
* headersToLogs:
* - content-type
* - accept
* - origin
* backendResponse:
* bodyBytes: 32
* headersToLogs:
* - content-type
* - content-length
* - origin
* ```
*
* ## Import
* API Management Diagnostics can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:apimanagement/diagnostic:Diagnostic example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/mygroup1/providers/Microsoft.ApiManagement/service/instance1/diagnostics/applicationinsights
* ```
* @property alwaysLogErrors Always log errors. Send telemetry if there is an erroneous condition, regardless of sampling settings.
* @property apiManagementLoggerId The id of the target API Management Logger where the API Management Diagnostic should be saved.
* @property apiManagementName The Name of the API Management Service where this Diagnostic should be created. Changing this forces a new resource to be created.
* @property backendRequest A `backend_request` block as defined below.
* @property backendResponse A `backend_response` block as defined below.
* @property frontendRequest A `frontend_request` block as defined below.
* @property frontendResponse A `frontend_response` block as defined below.
* @property httpCorrelationProtocol The HTTP Correlation Protocol to use. Possible values are `None`, `Legacy` or `W3C`.
* @property identifier The diagnostic identifier for the API Management Service. At this time the supported values are `applicationinsights` and `azuremonitor`. Changing this forces a new resource to be created.
* @property logClientIp Log client IP address.
* @property operationNameFormat The format of the Operation Name for Application Insights telemetries. Possible values are `Name`, and `Url`. Defaults to `Name`.
* @property resourceGroupName The Name of the Resource Group where the API Management Service exists. Changing this forces a new resource to be created.
* @property samplingPercentage Sampling (%). For high traffic APIs, please read this [documentation](https://docs.microsoft.com/azure/api-management/api-management-howto-app-insights#performance-implications-and-log-sampling) to understand performance implications and log sampling. Valid values are between `0.0` and `100.0`.
* @property verbosity Logging verbosity. Possible values are `verbose`, `information` or `error`.
*/
public data class DiagnosticArgs(
public val alwaysLogErrors: Output? = null,
public val apiManagementLoggerId: Output? = null,
public val apiManagementName: Output? = null,
public val backendRequest: Output? = null,
public val backendResponse: Output? = null,
public val frontendRequest: Output? = null,
public val frontendResponse: Output? = null,
public val httpCorrelationProtocol: Output? = null,
public val identifier: Output? = null,
public val logClientIp: Output? = null,
public val operationNameFormat: Output? = null,
public val resourceGroupName: Output? = null,
public val samplingPercentage: Output? = null,
public val verbosity: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.apimanagement.DiagnosticArgs =
com.pulumi.azure.apimanagement.DiagnosticArgs.builder()
.alwaysLogErrors(alwaysLogErrors?.applyValue({ args0 -> args0 }))
.apiManagementLoggerId(apiManagementLoggerId?.applyValue({ args0 -> args0 }))
.apiManagementName(apiManagementName?.applyValue({ args0 -> args0 }))
.backendRequest(backendRequest?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.backendResponse(backendResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.frontendRequest(frontendRequest?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.frontendResponse(frontendResponse?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.httpCorrelationProtocol(httpCorrelationProtocol?.applyValue({ args0 -> args0 }))
.identifier(identifier?.applyValue({ args0 -> args0 }))
.logClientIp(logClientIp?.applyValue({ args0 -> args0 }))
.operationNameFormat(operationNameFormat?.applyValue({ args0 -> args0 }))
.resourceGroupName(resourceGroupName?.applyValue({ args0 -> args0 }))
.samplingPercentage(samplingPercentage?.applyValue({ args0 -> args0 }))
.verbosity(verbosity?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [DiagnosticArgs].
*/
@PulumiTagMarker
public class DiagnosticArgsBuilder internal constructor() {
private var alwaysLogErrors: Output? = null
private var apiManagementLoggerId: Output? = null
private var apiManagementName: Output? = null
private var backendRequest: Output? = null
private var backendResponse: Output? = null
private var frontendRequest: Output? = null
private var frontendResponse: Output? = null
private var httpCorrelationProtocol: Output? = null
private var identifier: Output? = null
private var logClientIp: Output? = null
private var operationNameFormat: Output? = null
private var resourceGroupName: Output? = null
private var samplingPercentage: Output? = null
private var verbosity: Output? = null
/**
* @param value Always log errors. Send telemetry if there is an erroneous condition, regardless of sampling settings.
*/
@JvmName("tvquilragjhafrnq")
public suspend fun alwaysLogErrors(`value`: Output) {
this.alwaysLogErrors = value
}
/**
* @param value The id of the target API Management Logger where the API Management Diagnostic should be saved.
*/
@JvmName("nrirdvfdvwpkokov")
public suspend fun apiManagementLoggerId(`value`: Output) {
this.apiManagementLoggerId = value
}
/**
* @param value The Name of the API Management Service where this Diagnostic should be created. Changing this forces a new resource to be created.
*/
@JvmName("bxtclcgdviibghwr")
public suspend fun apiManagementName(`value`: Output) {
this.apiManagementName = value
}
/**
* @param value A `backend_request` block as defined below.
*/
@JvmName("tbmksbrjhdeliahs")
public suspend fun backendRequest(`value`: Output) {
this.backendRequest = value
}
/**
* @param value A `backend_response` block as defined below.
*/
@JvmName("aauiwpagwnwyfkgb")
public suspend fun backendResponse(`value`: Output) {
this.backendResponse = value
}
/**
* @param value A `frontend_request` block as defined below.
*/
@JvmName("ildjkoanbkvxmiuf")
public suspend fun frontendRequest(`value`: Output) {
this.frontendRequest = value
}
/**
* @param value A `frontend_response` block as defined below.
*/
@JvmName("rlytmbvknbnjmkhr")
public suspend fun frontendResponse(`value`: Output) {
this.frontendResponse = value
}
/**
* @param value The HTTP Correlation Protocol to use. Possible values are `None`, `Legacy` or `W3C`.
*/
@JvmName("rymqnnwceccoigvx")
public suspend fun httpCorrelationProtocol(`value`: Output) {
this.httpCorrelationProtocol = value
}
/**
* @param value The diagnostic identifier for the API Management Service. At this time the supported values are `applicationinsights` and `azuremonitor`. Changing this forces a new resource to be created.
*/
@JvmName("xowumwypxbxiigmj")
public suspend fun identifier(`value`: Output) {
this.identifier = value
}
/**
* @param value Log client IP address.
*/
@JvmName("maxecsjucjcqocwt")
public suspend fun logClientIp(`value`: Output) {
this.logClientIp = value
}
/**
* @param value The format of the Operation Name for Application Insights telemetries. Possible values are `Name`, and `Url`. Defaults to `Name`.
*/
@JvmName("jkftvdkhsgoysugw")
public suspend fun operationNameFormat(`value`: Output) {
this.operationNameFormat = value
}
/**
* @param value The Name of the Resource Group where the API Management Service exists. Changing this forces a new resource to be created.
*/
@JvmName("mdajmxrotcyvdxre")
public suspend fun resourceGroupName(`value`: Output) {
this.resourceGroupName = value
}
/**
* @param value Sampling (%). For high traffic APIs, please read this [documentation](https://docs.microsoft.com/azure/api-management/api-management-howto-app-insights#performance-implications-and-log-sampling) to understand performance implications and log sampling. Valid values are between `0.0` and `100.0`.
*/
@JvmName("edrltraveqcshvus")
public suspend fun samplingPercentage(`value`: Output) {
this.samplingPercentage = value
}
/**
* @param value Logging verbosity. Possible values are `verbose`, `information` or `error`.
*/
@JvmName("emtumlljqxpynrki")
public suspend fun verbosity(`value`: Output) {
this.verbosity = value
}
/**
* @param value Always log errors. Send telemetry if there is an erroneous condition, regardless of sampling settings.
*/
@JvmName("kiqchgkcebrcfuaj")
public suspend fun alwaysLogErrors(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.alwaysLogErrors = mapped
}
/**
* @param value The id of the target API Management Logger where the API Management Diagnostic should be saved.
*/
@JvmName("rjtqxgyveypqbsxo")
public suspend fun apiManagementLoggerId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiManagementLoggerId = mapped
}
/**
* @param value The Name of the API Management Service where this Diagnostic should be created. Changing this forces a new resource to be created.
*/
@JvmName("wawueyxiddmimjyt")
public suspend fun apiManagementName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.apiManagementName = mapped
}
/**
* @param value A `backend_request` block as defined below.
*/
@JvmName("kxqkadpnjekyasao")
public suspend fun backendRequest(`value`: DiagnosticBackendRequestArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backendRequest = mapped
}
/**
* @param argument A `backend_request` block as defined below.
*/
@JvmName("hmjvikyqkrsovobh")
public suspend
fun backendRequest(argument: suspend DiagnosticBackendRequestArgsBuilder.() -> Unit) {
val toBeMapped = DiagnosticBackendRequestArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.backendRequest = mapped
}
/**
* @param value A `backend_response` block as defined below.
*/
@JvmName("ibqnlorytrpcuqbd")
public suspend fun backendResponse(`value`: DiagnosticBackendResponseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.backendResponse = mapped
}
/**
* @param argument A `backend_response` block as defined below.
*/
@JvmName("rkwieeswanqplayu")
public suspend
fun backendResponse(argument: suspend DiagnosticBackendResponseArgsBuilder.() -> Unit) {
val toBeMapped = DiagnosticBackendResponseArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.backendResponse = mapped
}
/**
* @param value A `frontend_request` block as defined below.
*/
@JvmName("htmvloeqsyeecjrt")
public suspend fun frontendRequest(`value`: DiagnosticFrontendRequestArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.frontendRequest = mapped
}
/**
* @param argument A `frontend_request` block as defined below.
*/
@JvmName("pylgukxfddforxes")
public suspend
fun frontendRequest(argument: suspend DiagnosticFrontendRequestArgsBuilder.() -> Unit) {
val toBeMapped = DiagnosticFrontendRequestArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.frontendRequest = mapped
}
/**
* @param value A `frontend_response` block as defined below.
*/
@JvmName("nsiimwcugsyqdokw")
public suspend fun frontendResponse(`value`: DiagnosticFrontendResponseArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.frontendResponse = mapped
}
/**
* @param argument A `frontend_response` block as defined below.
*/
@JvmName("ewefdeocyvamcxcr")
public suspend
fun frontendResponse(argument: suspend DiagnosticFrontendResponseArgsBuilder.() -> Unit) {
val toBeMapped = DiagnosticFrontendResponseArgsBuilder().applySuspend { argument() }.build()
val mapped = of(toBeMapped)
this.frontendResponse = mapped
}
/**
* @param value The HTTP Correlation Protocol to use. Possible values are `None`, `Legacy` or `W3C`.
*/
@JvmName("cywqjavpxuionrih")
public suspend fun httpCorrelationProtocol(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.httpCorrelationProtocol = mapped
}
/**
* @param value The diagnostic identifier for the API Management Service. At this time the supported values are `applicationinsights` and `azuremonitor`. Changing this forces a new resource to be created.
*/
@JvmName("nhntsqopugbsvakc")
public suspend fun identifier(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.identifier = mapped
}
/**
* @param value Log client IP address.
*/
@JvmName("uwcwxeshyulyucoo")
public suspend fun logClientIp(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.logClientIp = mapped
}
/**
* @param value The format of the Operation Name for Application Insights telemetries. Possible values are `Name`, and `Url`. Defaults to `Name`.
*/
@JvmName("qfjorqsjeumhrqqh")
public suspend fun operationNameFormat(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.operationNameFormat = mapped
}
/**
* @param value The Name of the Resource Group where the API Management Service exists. Changing this forces a new resource to be created.
*/
@JvmName("yqidnhjtpshmjfsk")
public suspend fun resourceGroupName(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.resourceGroupName = mapped
}
/**
* @param value Sampling (%). For high traffic APIs, please read this [documentation](https://docs.microsoft.com/azure/api-management/api-management-howto-app-insights#performance-implications-and-log-sampling) to understand performance implications and log sampling. Valid values are between `0.0` and `100.0`.
*/
@JvmName("rpmfnqpywhkjglpc")
public suspend fun samplingPercentage(`value`: Double?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.samplingPercentage = mapped
}
/**
* @param value Logging verbosity. Possible values are `verbose`, `information` or `error`.
*/
@JvmName("gifkdiojfmyboofj")
public suspend fun verbosity(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.verbosity = mapped
}
internal fun build(): DiagnosticArgs = DiagnosticArgs(
alwaysLogErrors = alwaysLogErrors,
apiManagementLoggerId = apiManagementLoggerId,
apiManagementName = apiManagementName,
backendRequest = backendRequest,
backendResponse = backendResponse,
frontendRequest = frontendRequest,
frontendResponse = frontendResponse,
httpCorrelationProtocol = httpCorrelationProtocol,
identifier = identifier,
logClientIp = logClientIp,
operationNameFormat = operationNameFormat,
resourceGroupName = resourceGroupName,
samplingPercentage = samplingPercentage,
verbosity = verbosity,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy