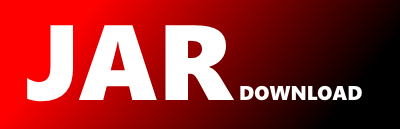
com.pulumi.azure.apimanagement.kotlin.GatewayCertificateAuthority.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
/**
* Builder for [GatewayCertificateAuthority].
*/
@PulumiTagMarker
public class GatewayCertificateAuthorityResourceBuilder internal constructor() {
public var name: String? = null
public var args: GatewayCertificateAuthorityArgs = GatewayCertificateAuthorityArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend GatewayCertificateAuthorityArgsBuilder.() -> Unit) {
val builder = GatewayCertificateAuthorityArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): GatewayCertificateAuthority {
val builtJavaResource =
com.pulumi.azure.apimanagement.GatewayCertificateAuthority(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return GatewayCertificateAuthority(builtJavaResource)
}
}
/**
* Manages an API Management Gateway Certificate Authority.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* import * as std from "@pulumi/std";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleService = new azure.apimanagement.Service("example", {
* name: "example-apim",
* location: example.location,
* resourceGroupName: example.name,
* publisherName: "pub1",
* publisherEmail: "[email protected]",
* skuName: "Consumption_0",
* });
* const exampleGateway = new azure.apimanagement.Gateway("example", {
* name: "example-gateway",
* apiManagementId: exampleService.id,
* description: "Example API Management gateway",
* locationData: {
* name: "example name",
* city: "example city",
* district: "example district",
* region: "example region",
* },
* });
* const exampleCertificate = new azure.apimanagement.Certificate("example", {
* name: "example-cert",
* apiManagementName: exampleService.name,
* resourceGroupName: example.name,
* data: std.filebase64({
* input: "example.pfx",
* }).then(invoke => invoke.result),
* });
* const exampleGatewayCertificateAuthority = new azure.apimanagement.GatewayCertificateAuthority("example", {
* apiManagementId: exampleService.id,
* certificateName: exampleCertificate.name,
* gatewayName: exampleGateway.name,
* isTrusted: true,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* import pulumi_std as std
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_service = azure.apimanagement.Service("example",
* name="example-apim",
* location=example.location,
* resource_group_name=example.name,
* publisher_name="pub1",
* publisher_email="[email protected]",
* sku_name="Consumption_0")
* example_gateway = azure.apimanagement.Gateway("example",
* name="example-gateway",
* api_management_id=example_service.id,
* description="Example API Management gateway",
* location_data=azure.apimanagement.GatewayLocationDataArgs(
* name="example name",
* city="example city",
* district="example district",
* region="example region",
* ))
* example_certificate = azure.apimanagement.Certificate("example",
* name="example-cert",
* api_management_name=example_service.name,
* resource_group_name=example.name,
* data=std.filebase64(input="example.pfx").result)
* example_gateway_certificate_authority = azure.apimanagement.GatewayCertificateAuthority("example",
* api_management_id=example_service.id,
* certificate_name=example_certificate.name,
* gateway_name=example_gateway.name,
* is_trusted=True)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* using Std = Pulumi.Std;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleService = new Azure.ApiManagement.Service("example", new()
* {
* Name = "example-apim",
* Location = example.Location,
* ResourceGroupName = example.Name,
* PublisherName = "pub1",
* PublisherEmail = "[email protected]",
* SkuName = "Consumption_0",
* });
* var exampleGateway = new Azure.ApiManagement.Gateway("example", new()
* {
* Name = "example-gateway",
* ApiManagementId = exampleService.Id,
* Description = "Example API Management gateway",
* LocationData = new Azure.ApiManagement.Inputs.GatewayLocationDataArgs
* {
* Name = "example name",
* City = "example city",
* District = "example district",
* Region = "example region",
* },
* });
* var exampleCertificate = new Azure.ApiManagement.Certificate("example", new()
* {
* Name = "example-cert",
* ApiManagementName = exampleService.Name,
* ResourceGroupName = example.Name,
* Data = Std.Filebase64.Invoke(new()
* {
* Input = "example.pfx",
* }).Apply(invoke => invoke.Result),
* });
* var exampleGatewayCertificateAuthority = new Azure.ApiManagement.GatewayCertificateAuthority("example", new()
* {
* ApiManagementId = exampleService.Id,
* CertificateName = exampleCertificate.Name,
* GatewayName = exampleGateway.Name,
* IsTrusted = true,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/apimanagement"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-std/sdk/go/std"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleService, err := apimanagement.NewService(ctx, "example", &apimanagement.ServiceArgs{
* Name: pulumi.String("example-apim"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* PublisherName: pulumi.String("pub1"),
* PublisherEmail: pulumi.String("[email protected]"),
* SkuName: pulumi.String("Consumption_0"),
* })
* if err != nil {
* return err
* }
* exampleGateway, err := apimanagement.NewGateway(ctx, "example", &apimanagement.GatewayArgs{
* Name: pulumi.String("example-gateway"),
* ApiManagementId: exampleService.ID(),
* Description: pulumi.String("Example API Management gateway"),
* LocationData: &apimanagement.GatewayLocationDataArgs{
* Name: pulumi.String("example name"),
* City: pulumi.String("example city"),
* District: pulumi.String("example district"),
* Region: pulumi.String("example region"),
* },
* })
* if err != nil {
* return err
* }
* invokeFilebase64, err := std.Filebase64(ctx, &std.Filebase64Args{
* Input: "example.pfx",
* }, nil)
* if err != nil {
* return err
* }
* exampleCertificate, err := apimanagement.NewCertificate(ctx, "example", &apimanagement.CertificateArgs{
* Name: pulumi.String("example-cert"),
* ApiManagementName: exampleService.Name,
* ResourceGroupName: example.Name,
* Data: invokeFilebase64.Result,
* })
* if err != nil {
* return err
* }
* _, err = apimanagement.NewGatewayCertificateAuthority(ctx, "example", &apimanagement.GatewayCertificateAuthorityArgs{
* ApiManagementId: exampleService.ID(),
* CertificateName: exampleCertificate.Name,
* GatewayName: exampleGateway.Name,
* IsTrusted: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.apimanagement.Service;
* import com.pulumi.azure.apimanagement.ServiceArgs;
* import com.pulumi.azure.apimanagement.Gateway;
* import com.pulumi.azure.apimanagement.GatewayArgs;
* import com.pulumi.azure.apimanagement.inputs.GatewayLocationDataArgs;
* import com.pulumi.azure.apimanagement.Certificate;
* import com.pulumi.azure.apimanagement.CertificateArgs;
* import com.pulumi.azure.apimanagement.GatewayCertificateAuthority;
* import com.pulumi.azure.apimanagement.GatewayCertificateAuthorityArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleService = new Service("exampleService", ServiceArgs.builder()
* .name("example-apim")
* .location(example.location())
* .resourceGroupName(example.name())
* .publisherName("pub1")
* .publisherEmail("[email protected]")
* .skuName("Consumption_0")
* .build());
* var exampleGateway = new Gateway("exampleGateway", GatewayArgs.builder()
* .name("example-gateway")
* .apiManagementId(exampleService.id())
* .description("Example API Management gateway")
* .locationData(GatewayLocationDataArgs.builder()
* .name("example name")
* .city("example city")
* .district("example district")
* .region("example region")
* .build())
* .build());
* var exampleCertificate = new Certificate("exampleCertificate", CertificateArgs.builder()
* .name("example-cert")
* .apiManagementName(exampleService.name())
* .resourceGroupName(example.name())
* .data(StdFunctions.filebase64(Filebase64Args.builder()
* .input("example.pfx")
* .build()).result())
* .build());
* var exampleGatewayCertificateAuthority = new GatewayCertificateAuthority("exampleGatewayCertificateAuthority", GatewayCertificateAuthorityArgs.builder()
* .apiManagementId(exampleService.id())
* .certificateName(exampleCertificate.name())
* .gatewayName(exampleGateway.name())
* .isTrusted(true)
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleService:
* type: azure:apimanagement:Service
* name: example
* properties:
* name: example-apim
* location: ${example.location}
* resourceGroupName: ${example.name}
* publisherName: pub1
* publisherEmail: [email protected]
* skuName: Consumption_0
* exampleGateway:
* type: azure:apimanagement:Gateway
* name: example
* properties:
* name: example-gateway
* apiManagementId: ${exampleService.id}
* description: Example API Management gateway
* locationData:
* name: example name
* city: example city
* district: example district
* region: example region
* exampleCertificate:
* type: azure:apimanagement:Certificate
* name: example
* properties:
* name: example-cert
* apiManagementName: ${exampleService.name}
* resourceGroupName: ${example.name}
* data:
* fn::invoke:
* Function: std:filebase64
* Arguments:
* input: example.pfx
* Return: result
* exampleGatewayCertificateAuthority:
* type: azure:apimanagement:GatewayCertificateAuthority
* name: example
* properties:
* apiManagementId: ${exampleService.id}
* certificateName: ${exampleCertificate.name}
* gatewayName: ${exampleGateway.name}
* isTrusted: true
* ```
*
* ## Import
* API Management Gateway Certificate Authority can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:apimanagement/gatewayCertificateAuthority:GatewayCertificateAuthority example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.ApiManagement/service/service1/gateways/gateway1/certificateAuthorities/cert1
* ```
*/
public class GatewayCertificateAuthority internal constructor(
override val javaResource: com.pulumi.azure.apimanagement.GatewayCertificateAuthority,
) : KotlinCustomResource(javaResource, GatewayCertificateAuthorityMapper) {
/**
* The ID of the API Management Service. Changing this forces a new resource to be created.
*/
public val apiManagementId: Output
get() = javaResource.apiManagementId().applyValue({ args0 -> args0 })
/**
* The name of the API Management Certificate. Changing this forces a new resource to be created.
*/
public val certificateName: Output
get() = javaResource.certificateName().applyValue({ args0 -> args0 })
/**
* The name of the API Management Gateway. Changing this forces a new resource to be created.
*/
public val gatewayName: Output
get() = javaResource.gatewayName().applyValue({ args0 -> args0 })
/**
* Whether the API Management Gateway Certificate Authority is trusted.
*/
public val isTrusted: Output?
get() = javaResource.isTrusted().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
}
public object GatewayCertificateAuthorityMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.apimanagement.GatewayCertificateAuthority::class == javaResource::class
override fun map(javaResource: Resource): GatewayCertificateAuthority =
GatewayCertificateAuthority(
javaResource as
com.pulumi.azure.apimanagement.GatewayCertificateAuthority,
)
}
/**
* @see [GatewayCertificateAuthority].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [GatewayCertificateAuthority].
*/
public suspend fun gatewayCertificateAuthority(
name: String,
block: suspend GatewayCertificateAuthorityResourceBuilder.() -> Unit,
):
GatewayCertificateAuthority {
val builder = GatewayCertificateAuthorityResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [GatewayCertificateAuthority].
* @param name The _unique_ name of the resulting resource.
*/
public fun gatewayCertificateAuthority(name: String): GatewayCertificateAuthority {
val builder = GatewayCertificateAuthorityResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy