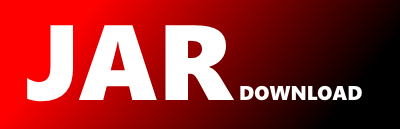
com.pulumi.azure.apimanagement.kotlin.inputs.BackendServiceFabricClusterArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin.inputs
import com.pulumi.azure.apimanagement.inputs.BackendServiceFabricClusterArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property clientCertificateId The client certificate resource id for the management endpoint.
* > **Note:** At least one of `client_certificate_thumbprint`, and `client_certificate_id` must be set.
* >
* @property clientCertificateThumbprint The client certificate thumbprint for the management endpoint.
* @property managementEndpoints A list of cluster management endpoints.
* @property maxPartitionResolutionRetries The maximum number of retries when attempting resolve the partition.
* @property serverCertificateThumbprints A list of thumbprints of the server certificates of the Service Fabric cluster.
* @property serverX509Names One or more `server_x509_name` blocks as documented below.
*/
public data class BackendServiceFabricClusterArgs(
public val clientCertificateId: Output? = null,
public val clientCertificateThumbprint: Output? = null,
public val managementEndpoints: Output>,
public val maxPartitionResolutionRetries: Output,
public val serverCertificateThumbprints: Output>? = null,
public val serverX509Names: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.apimanagement.inputs.BackendServiceFabricClusterArgs =
com.pulumi.azure.apimanagement.inputs.BackendServiceFabricClusterArgs.builder()
.clientCertificateId(clientCertificateId?.applyValue({ args0 -> args0 }))
.clientCertificateThumbprint(clientCertificateThumbprint?.applyValue({ args0 -> args0 }))
.managementEndpoints(managementEndpoints.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.maxPartitionResolutionRetries(maxPartitionResolutionRetries.applyValue({ args0 -> args0 }))
.serverCertificateThumbprints(
serverCertificateThumbprints?.applyValue({ args0 ->
args0.map({ args0 -> args0 })
}),
)
.serverX509Names(
serverX509Names?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
).build()
}
/**
* Builder for [BackendServiceFabricClusterArgs].
*/
@PulumiTagMarker
public class BackendServiceFabricClusterArgsBuilder internal constructor() {
private var clientCertificateId: Output? = null
private var clientCertificateThumbprint: Output? = null
private var managementEndpoints: Output>? = null
private var maxPartitionResolutionRetries: Output? = null
private var serverCertificateThumbprints: Output>? = null
private var serverX509Names: Output>? = null
/**
* @param value The client certificate resource id for the management endpoint.
* > **Note:** At least one of `client_certificate_thumbprint`, and `client_certificate_id` must be set.
* >
*/
@JvmName("qtkvupnyqiuvqwqx")
public suspend fun clientCertificateId(`value`: Output) {
this.clientCertificateId = value
}
/**
* @param value The client certificate thumbprint for the management endpoint.
*/
@JvmName("wirgscsynrxujxud")
public suspend fun clientCertificateThumbprint(`value`: Output) {
this.clientCertificateThumbprint = value
}
/**
* @param value A list of cluster management endpoints.
*/
@JvmName("tfxxvldpojctwgik")
public suspend fun managementEndpoints(`value`: Output>) {
this.managementEndpoints = value
}
@JvmName("ghdwnkrjcnduswks")
public suspend fun managementEndpoints(vararg values: Output) {
this.managementEndpoints = Output.all(values.asList())
}
/**
* @param values A list of cluster management endpoints.
*/
@JvmName("cfhskevgicqpubqp")
public suspend fun managementEndpoints(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy