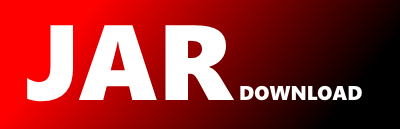
com.pulumi.azure.apimanagement.kotlin.outputs.ServiceSecurity.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.apimanagement.kotlin.outputs
import kotlin.Boolean
import kotlin.Suppress
/**
*
* @property enableBackendSsl30 Should SSL 3.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Ssl30` field
* @property enableBackendTls10 Should TLS 1.0 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls10` field
* @property enableBackendTls11 Should TLS 1.1 be enabled on the backend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Backend.Protocols.Tls11` field
* @property enableFrontendSsl30 Should SSL 3.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Ssl30` field
* @property enableFrontendTls10 Should TLS 1.0 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls10` field
* @property enableFrontendTls11 Should TLS 1.1 be enabled on the frontend of the gateway? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Protocols.Tls11` field
* @property tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled Should the `TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_128_CBC_SHA` field
* @property tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled Should the `TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_ECDSA_WITH_AES_256_CBC_SHA` field
* @property tlsEcdheRsaWithAes128CbcShaCiphersEnabled Should the `TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_128_CBC_SHA` field
* @property tlsEcdheRsaWithAes256CbcShaCiphersEnabled Should the `TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_ECDHE_RSA_WITH_AES_256_CBC_SHA` field
* @property tlsRsaWithAes128CbcSha256CiphersEnabled Should the `TLS_RSA_WITH_AES_128_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA256` field
* @property tlsRsaWithAes128CbcShaCiphersEnabled Should the `TLS_RSA_WITH_AES_128_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_CBC_SHA` field
* @property tlsRsaWithAes128GcmSha256CiphersEnabled Should the `TLS_RSA_WITH_AES_128_GCM_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_128_GCM_SHA256` field
* @property tlsRsaWithAes256CbcSha256CiphersEnabled Should the `TLS_RSA_WITH_AES_256_CBC_SHA256` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA256` field
* @property tlsRsaWithAes256CbcShaCiphersEnabled Should the `TLS_RSA_WITH_AES_256_CBC_SHA` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_CBC_SHA` field
* @property tlsRsaWithAes256GcmSha384CiphersEnabled Should the `TLS_RSA_WITH_AES_256_GCM_SHA384` cipher be enabled? Defaults to `false`.
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TLS_RSA_WITH_AES_256_GCM_SHA384` field
* @property tripleDesCiphersEnabled Should the `TLS_RSA_WITH_3DES_EDE_CBC_SHA` cipher be enabled for alL TLS versions (1.0, 1.1 and 1.2)?
* > **info:** This maps to the `Microsoft.WindowsAzure.ApiManagement.Gateway.Security.Ciphers.TripleDes168` field
*/
public data class ServiceSecurity(
public val enableBackendSsl30: Boolean? = null,
public val enableBackendTls10: Boolean? = null,
public val enableBackendTls11: Boolean? = null,
public val enableFrontendSsl30: Boolean? = null,
public val enableFrontendTls10: Boolean? = null,
public val enableFrontendTls11: Boolean? = null,
public val tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled: Boolean? = null,
public val tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled: Boolean? = null,
public val tlsEcdheRsaWithAes128CbcShaCiphersEnabled: Boolean? = null,
public val tlsEcdheRsaWithAes256CbcShaCiphersEnabled: Boolean? = null,
public val tlsRsaWithAes128CbcSha256CiphersEnabled: Boolean? = null,
public val tlsRsaWithAes128CbcShaCiphersEnabled: Boolean? = null,
public val tlsRsaWithAes128GcmSha256CiphersEnabled: Boolean? = null,
public val tlsRsaWithAes256CbcSha256CiphersEnabled: Boolean? = null,
public val tlsRsaWithAes256CbcShaCiphersEnabled: Boolean? = null,
public val tlsRsaWithAes256GcmSha384CiphersEnabled: Boolean? = null,
public val tripleDesCiphersEnabled: Boolean? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.apimanagement.outputs.ServiceSecurity):
ServiceSecurity = ServiceSecurity(
enableBackendSsl30 = javaType.enableBackendSsl30().map({ args0 -> args0 }).orElse(null),
enableBackendTls10 = javaType.enableBackendTls10().map({ args0 -> args0 }).orElse(null),
enableBackendTls11 = javaType.enableBackendTls11().map({ args0 -> args0 }).orElse(null),
enableFrontendSsl30 = javaType.enableFrontendSsl30().map({ args0 -> args0 }).orElse(null),
enableFrontendTls10 = javaType.enableFrontendTls10().map({ args0 -> args0 }).orElse(null),
enableFrontendTls11 = javaType.enableFrontendTls11().map({ args0 -> args0 }).orElse(null),
tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled = javaType.tlsEcdheEcdsaWithAes128CbcShaCiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled = javaType.tlsEcdheEcdsaWithAes256CbcShaCiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsEcdheRsaWithAes128CbcShaCiphersEnabled = javaType.tlsEcdheRsaWithAes128CbcShaCiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsEcdheRsaWithAes256CbcShaCiphersEnabled = javaType.tlsEcdheRsaWithAes256CbcShaCiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsRsaWithAes128CbcSha256CiphersEnabled = javaType.tlsRsaWithAes128CbcSha256CiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsRsaWithAes128CbcShaCiphersEnabled = javaType.tlsRsaWithAes128CbcShaCiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsRsaWithAes128GcmSha256CiphersEnabled = javaType.tlsRsaWithAes128GcmSha256CiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsRsaWithAes256CbcSha256CiphersEnabled = javaType.tlsRsaWithAes256CbcSha256CiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsRsaWithAes256CbcShaCiphersEnabled = javaType.tlsRsaWithAes256CbcShaCiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tlsRsaWithAes256GcmSha384CiphersEnabled = javaType.tlsRsaWithAes256GcmSha384CiphersEnabled().map({ args0 ->
args0
}).orElse(null),
tripleDesCiphersEnabled = javaType.tripleDesCiphersEnabled().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy