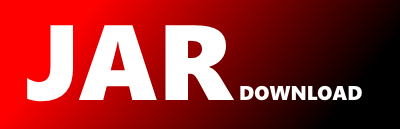
com.pulumi.azure.appconfiguration.kotlin.ConfigurationStoreArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appconfiguration.kotlin
import com.pulumi.azure.appconfiguration.ConfigurationStoreArgs.builder
import com.pulumi.azure.appconfiguration.kotlin.inputs.ConfigurationStoreEncryptionArgs
import com.pulumi.azure.appconfiguration.kotlin.inputs.ConfigurationStoreEncryptionArgsBuilder
import com.pulumi.azure.appconfiguration.kotlin.inputs.ConfigurationStoreIdentityArgs
import com.pulumi.azure.appconfiguration.kotlin.inputs.ConfigurationStoreIdentityArgsBuilder
import com.pulumi.azure.appconfiguration.kotlin.inputs.ConfigurationStoreReplicaArgs
import com.pulumi.azure.appconfiguration.kotlin.inputs.ConfigurationStoreReplicaArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const appconf = new azure.appconfiguration.ConfigurationStore("appconf", {
* name: "appConf1",
* resourceGroupName: example.name,
* location: example.location,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* appconf = azure.appconfiguration.ConfigurationStore("appconf",
* name="appConf1",
* resource_group_name=example.name,
* location=example.location)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var appconf = new Azure.AppConfiguration.ConfigurationStore("appconf", new()
* {
* Name = "appConf1",
* ResourceGroupName = example.Name,
* Location = example.Location,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/appconfiguration"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* _, err = appconfiguration.NewConfigurationStore(ctx, "appconf", &appconfiguration.ConfigurationStoreArgs{
* Name: pulumi.String("appConf1"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.appconfiguration.ConfigurationStore;
* import com.pulumi.azure.appconfiguration.ConfigurationStoreArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var appconf = new ConfigurationStore("appconf", ConfigurationStoreArgs.builder()
* .name("appConf1")
* .resourceGroupName(example.name())
* .location(example.location())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* appconf:
* type: azure:appconfiguration:ConfigurationStore
* properties:
* name: appConf1
* resourceGroupName: ${example.name}
* location: ${example.location}
* ```
*
* ### Encryption)
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleUserAssignedIdentity = new azure.authorization.UserAssignedIdentity("example", {
* name: "example-identity",
* location: example.location,
* resourceGroupName: example.name,
* });
* const current = azure.core.getClientConfig({});
* const exampleKeyVault = new azure.keyvault.KeyVault("example", {
* name: "exampleKVt123",
* location: example.location,
* resourceGroupName: example.name,
* tenantId: current.then(current => current.tenantId),
* skuName: "standard",
* softDeleteRetentionDays: 7,
* purgeProtectionEnabled: true,
* });
* const server = new azure.keyvault.AccessPolicy("server", {
* keyVaultId: exampleKeyVault.id,
* tenantId: current.then(current => current.tenantId),
* objectId: exampleUserAssignedIdentity.principalId,
* keyPermissions: [
* "Get",
* "UnwrapKey",
* "WrapKey",
* ],
* secretPermissions: ["Get"],
* });
* const client = new azure.keyvault.AccessPolicy("client", {
* keyVaultId: exampleKeyVault.id,
* tenantId: current.then(current => current.tenantId),
* objectId: current.then(current => current.objectId),
* keyPermissions: [
* "Get",
* "Create",
* "Delete",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify",
* "GetRotationPolicy",
* ],
* secretPermissions: ["Get"],
* });
* const exampleKey = new azure.keyvault.Key("example", {
* name: "exampleKVkey",
* keyVaultId: exampleKeyVault.id,
* keyType: "RSA",
* keySize: 2048,
* keyOpts: [
* "decrypt",
* "encrypt",
* "sign",
* "unwrapKey",
* "verify",
* "wrapKey",
* ],
* });
* const exampleConfigurationStore = new azure.appconfiguration.ConfigurationStore("example", {
* name: "appConf2",
* resourceGroupName: example.name,
* location: example.location,
* sku: "standard",
* localAuthEnabled: true,
* publicNetworkAccess: "Enabled",
* purgeProtectionEnabled: false,
* softDeleteRetentionDays: 1,
* identity: {
* type: "UserAssigned",
* identityIds: [exampleUserAssignedIdentity.id],
* },
* encryption: {
* keyVaultKeyIdentifier: exampleKey.id,
* identityClientId: exampleUserAssignedIdentity.clientId,
* },
* replicas: [{
* name: "replica1",
* location: "West US",
* }],
* tags: {
* environment: "development",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_user_assigned_identity = azure.authorization.UserAssignedIdentity("example",
* name="example-identity",
* location=example.location,
* resource_group_name=example.name)
* current = azure.core.get_client_config()
* example_key_vault = azure.keyvault.KeyVault("example",
* name="exampleKVt123",
* location=example.location,
* resource_group_name=example.name,
* tenant_id=current.tenant_id,
* sku_name="standard",
* soft_delete_retention_days=7,
* purge_protection_enabled=True)
* server = azure.keyvault.AccessPolicy("server",
* key_vault_id=example_key_vault.id,
* tenant_id=current.tenant_id,
* object_id=example_user_assigned_identity.principal_id,
* key_permissions=[
* "Get",
* "UnwrapKey",
* "WrapKey",
* ],
* secret_permissions=["Get"])
* client = azure.keyvault.AccessPolicy("client",
* key_vault_id=example_key_vault.id,
* tenant_id=current.tenant_id,
* object_id=current.object_id,
* key_permissions=[
* "Get",
* "Create",
* "Delete",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify",
* "GetRotationPolicy",
* ],
* secret_permissions=["Get"])
* example_key = azure.keyvault.Key("example",
* name="exampleKVkey",
* key_vault_id=example_key_vault.id,
* key_type="RSA",
* key_size=2048,
* key_opts=[
* "decrypt",
* "encrypt",
* "sign",
* "unwrapKey",
* "verify",
* "wrapKey",
* ])
* example_configuration_store = azure.appconfiguration.ConfigurationStore("example",
* name="appConf2",
* resource_group_name=example.name,
* location=example.location,
* sku="standard",
* local_auth_enabled=True,
* public_network_access="Enabled",
* purge_protection_enabled=False,
* soft_delete_retention_days=1,
* identity=azure.appconfiguration.ConfigurationStoreIdentityArgs(
* type="UserAssigned",
* identity_ids=[example_user_assigned_identity.id],
* ),
* encryption=azure.appconfiguration.ConfigurationStoreEncryptionArgs(
* key_vault_key_identifier=example_key.id,
* identity_client_id=example_user_assigned_identity.client_id,
* ),
* replicas=[azure.appconfiguration.ConfigurationStoreReplicaArgs(
* name="replica1",
* location="West US",
* )],
* tags={
* "environment": "development",
* })
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleUserAssignedIdentity = new Azure.Authorization.UserAssignedIdentity("example", new()
* {
* Name = "example-identity",
* Location = example.Location,
* ResourceGroupName = example.Name,
* });
* var current = Azure.Core.GetClientConfig.Invoke();
* var exampleKeyVault = new Azure.KeyVault.KeyVault("example", new()
* {
* Name = "exampleKVt123",
* Location = example.Location,
* ResourceGroupName = example.Name,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* SkuName = "standard",
* SoftDeleteRetentionDays = 7,
* PurgeProtectionEnabled = true,
* });
* var server = new Azure.KeyVault.AccessPolicy("server", new()
* {
* KeyVaultId = exampleKeyVault.Id,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* ObjectId = exampleUserAssignedIdentity.PrincipalId,
* KeyPermissions = new[]
* {
* "Get",
* "UnwrapKey",
* "WrapKey",
* },
* SecretPermissions = new[]
* {
* "Get",
* },
* });
* var client = new Azure.KeyVault.AccessPolicy("client", new()
* {
* KeyVaultId = exampleKeyVault.Id,
* TenantId = current.Apply(getClientConfigResult => getClientConfigResult.TenantId),
* ObjectId = current.Apply(getClientConfigResult => getClientConfigResult.ObjectId),
* KeyPermissions = new[]
* {
* "Get",
* "Create",
* "Delete",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify",
* "GetRotationPolicy",
* },
* SecretPermissions = new[]
* {
* "Get",
* },
* });
* var exampleKey = new Azure.KeyVault.Key("example", new()
* {
* Name = "exampleKVkey",
* KeyVaultId = exampleKeyVault.Id,
* KeyType = "RSA",
* KeySize = 2048,
* KeyOpts = new[]
* {
* "decrypt",
* "encrypt",
* "sign",
* "unwrapKey",
* "verify",
* "wrapKey",
* },
* });
* var exampleConfigurationStore = new Azure.AppConfiguration.ConfigurationStore("example", new()
* {
* Name = "appConf2",
* ResourceGroupName = example.Name,
* Location = example.Location,
* Sku = "standard",
* LocalAuthEnabled = true,
* PublicNetworkAccess = "Enabled",
* PurgeProtectionEnabled = false,
* SoftDeleteRetentionDays = 1,
* Identity = new Azure.AppConfiguration.Inputs.ConfigurationStoreIdentityArgs
* {
* Type = "UserAssigned",
* IdentityIds = new[]
* {
* exampleUserAssignedIdentity.Id,
* },
* },
* Encryption = new Azure.AppConfiguration.Inputs.ConfigurationStoreEncryptionArgs
* {
* KeyVaultKeyIdentifier = exampleKey.Id,
* IdentityClientId = exampleUserAssignedIdentity.ClientId,
* },
* Replicas = new[]
* {
* new Azure.AppConfiguration.Inputs.ConfigurationStoreReplicaArgs
* {
* Name = "replica1",
* Location = "West US",
* },
* },
* Tags =
* {
* { "environment", "development" },
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/appconfiguration"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/authorization"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/keyvault"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleUserAssignedIdentity, err := authorization.NewUserAssignedIdentity(ctx, "example", &authorization.UserAssignedIdentityArgs{
* Name: pulumi.String("example-identity"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* })
* if err != nil {
* return err
* }
* current, err := core.GetClientConfig(ctx, nil, nil)
* if err != nil {
* return err
* }
* exampleKeyVault, err := keyvault.NewKeyVault(ctx, "example", &keyvault.KeyVaultArgs{
* Name: pulumi.String("exampleKVt123"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* TenantId: pulumi.String(current.TenantId),
* SkuName: pulumi.String("standard"),
* SoftDeleteRetentionDays: pulumi.Int(7),
* PurgeProtectionEnabled: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* _, err = keyvault.NewAccessPolicy(ctx, "server", &keyvault.AccessPolicyArgs{
* KeyVaultId: exampleKeyVault.ID(),
* TenantId: pulumi.String(current.TenantId),
* ObjectId: exampleUserAssignedIdentity.PrincipalId,
* KeyPermissions: pulumi.StringArray{
* pulumi.String("Get"),
* pulumi.String("UnwrapKey"),
* pulumi.String("WrapKey"),
* },
* SecretPermissions: pulumi.StringArray{
* pulumi.String("Get"),
* },
* })
* if err != nil {
* return err
* }
* _, err = keyvault.NewAccessPolicy(ctx, "client", &keyvault.AccessPolicyArgs{
* KeyVaultId: exampleKeyVault.ID(),
* TenantId: pulumi.String(current.TenantId),
* ObjectId: pulumi.String(current.ObjectId),
* KeyPermissions: pulumi.StringArray{
* pulumi.String("Get"),
* pulumi.String("Create"),
* pulumi.String("Delete"),
* pulumi.String("List"),
* pulumi.String("Restore"),
* pulumi.String("Recover"),
* pulumi.String("UnwrapKey"),
* pulumi.String("WrapKey"),
* pulumi.String("Purge"),
* pulumi.String("Encrypt"),
* pulumi.String("Decrypt"),
* pulumi.String("Sign"),
* pulumi.String("Verify"),
* pulumi.String("GetRotationPolicy"),
* },
* SecretPermissions: pulumi.StringArray{
* pulumi.String("Get"),
* },
* })
* if err != nil {
* return err
* }
* exampleKey, err := keyvault.NewKey(ctx, "example", &keyvault.KeyArgs{
* Name: pulumi.String("exampleKVkey"),
* KeyVaultId: exampleKeyVault.ID(),
* KeyType: pulumi.String("RSA"),
* KeySize: pulumi.Int(2048),
* KeyOpts: pulumi.StringArray{
* pulumi.String("decrypt"),
* pulumi.String("encrypt"),
* pulumi.String("sign"),
* pulumi.String("unwrapKey"),
* pulumi.String("verify"),
* pulumi.String("wrapKey"),
* },
* })
* if err != nil {
* return err
* }
* _, err = appconfiguration.NewConfigurationStore(ctx, "example", &appconfiguration.ConfigurationStoreArgs{
* Name: pulumi.String("appConf2"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* Sku: pulumi.String("standard"),
* LocalAuthEnabled: pulumi.Bool(true),
* PublicNetworkAccess: pulumi.String("Enabled"),
* PurgeProtectionEnabled: pulumi.Bool(false),
* SoftDeleteRetentionDays: pulumi.Int(1),
* Identity: &appconfiguration.ConfigurationStoreIdentityArgs{
* Type: pulumi.String("UserAssigned"),
* IdentityIds: pulumi.StringArray{
* exampleUserAssignedIdentity.ID(),
* },
* },
* Encryption: &appconfiguration.ConfigurationStoreEncryptionArgs{
* KeyVaultKeyIdentifier: exampleKey.ID(),
* IdentityClientId: exampleUserAssignedIdentity.ClientId,
* },
* Replicas: appconfiguration.ConfigurationStoreReplicaArray{
* &appconfiguration.ConfigurationStoreReplicaArgs{
* Name: pulumi.String("replica1"),
* Location: pulumi.String("West US"),
* },
* },
* Tags: pulumi.StringMap{
* "environment": pulumi.String("development"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.authorization.UserAssignedIdentity;
* import com.pulumi.azure.authorization.UserAssignedIdentityArgs;
* import com.pulumi.azure.core.CoreFunctions;
* import com.pulumi.azure.keyvault.KeyVault;
* import com.pulumi.azure.keyvault.KeyVaultArgs;
* import com.pulumi.azure.keyvault.AccessPolicy;
* import com.pulumi.azure.keyvault.AccessPolicyArgs;
* import com.pulumi.azure.keyvault.Key;
* import com.pulumi.azure.keyvault.KeyArgs;
* import com.pulumi.azure.appconfiguration.ConfigurationStore;
* import com.pulumi.azure.appconfiguration.ConfigurationStoreArgs;
* import com.pulumi.azure.appconfiguration.inputs.ConfigurationStoreIdentityArgs;
* import com.pulumi.azure.appconfiguration.inputs.ConfigurationStoreEncryptionArgs;
* import com.pulumi.azure.appconfiguration.inputs.ConfigurationStoreReplicaArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleUserAssignedIdentity = new UserAssignedIdentity("exampleUserAssignedIdentity", UserAssignedIdentityArgs.builder()
* .name("example-identity")
* .location(example.location())
* .resourceGroupName(example.name())
* .build());
* final var current = CoreFunctions.getClientConfig();
* var exampleKeyVault = new KeyVault("exampleKeyVault", KeyVaultArgs.builder()
* .name("exampleKVt123")
* .location(example.location())
* .resourceGroupName(example.name())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .skuName("standard")
* .softDeleteRetentionDays(7)
* .purgeProtectionEnabled(true)
* .build());
* var server = new AccessPolicy("server", AccessPolicyArgs.builder()
* .keyVaultId(exampleKeyVault.id())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(exampleUserAssignedIdentity.principalId())
* .keyPermissions(
* "Get",
* "UnwrapKey",
* "WrapKey")
* .secretPermissions("Get")
* .build());
* var client = new AccessPolicy("client", AccessPolicyArgs.builder()
* .keyVaultId(exampleKeyVault.id())
* .tenantId(current.applyValue(getClientConfigResult -> getClientConfigResult.tenantId()))
* .objectId(current.applyValue(getClientConfigResult -> getClientConfigResult.objectId()))
* .keyPermissions(
* "Get",
* "Create",
* "Delete",
* "List",
* "Restore",
* "Recover",
* "UnwrapKey",
* "WrapKey",
* "Purge",
* "Encrypt",
* "Decrypt",
* "Sign",
* "Verify",
* "GetRotationPolicy")
* .secretPermissions("Get")
* .build());
* var exampleKey = new Key("exampleKey", KeyArgs.builder()
* .name("exampleKVkey")
* .keyVaultId(exampleKeyVault.id())
* .keyType("RSA")
* .keySize(2048)
* .keyOpts(
* "decrypt",
* "encrypt",
* "sign",
* "unwrapKey",
* "verify",
* "wrapKey")
* .build());
* var exampleConfigurationStore = new ConfigurationStore("exampleConfigurationStore", ConfigurationStoreArgs.builder()
* .name("appConf2")
* .resourceGroupName(example.name())
* .location(example.location())
* .sku("standard")
* .localAuthEnabled(true)
* .publicNetworkAccess("Enabled")
* .purgeProtectionEnabled(false)
* .softDeleteRetentionDays(1)
* .identity(ConfigurationStoreIdentityArgs.builder()
* .type("UserAssigned")
* .identityIds(exampleUserAssignedIdentity.id())
* .build())
* .encryption(ConfigurationStoreEncryptionArgs.builder()
* .keyVaultKeyIdentifier(exampleKey.id())
* .identityClientId(exampleUserAssignedIdentity.clientId())
* .build())
* .replicas(ConfigurationStoreReplicaArgs.builder()
* .name("replica1")
* .location("West US")
* .build())
* .tags(Map.of("environment", "development"))
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleUserAssignedIdentity:
* type: azure:authorization:UserAssignedIdentity
* name: example
* properties:
* name: example-identity
* location: ${example.location}
* resourceGroupName: ${example.name}
* exampleKeyVault:
* type: azure:keyvault:KeyVault
* name: example
* properties:
* name: exampleKVt123
* location: ${example.location}
* resourceGroupName: ${example.name}
* tenantId: ${current.tenantId}
* skuName: standard
* softDeleteRetentionDays: 7
* purgeProtectionEnabled: true
* server:
* type: azure:keyvault:AccessPolicy
* properties:
* keyVaultId: ${exampleKeyVault.id}
* tenantId: ${current.tenantId}
* objectId: ${exampleUserAssignedIdentity.principalId}
* keyPermissions:
* - Get
* - UnwrapKey
* - WrapKey
* secretPermissions:
* - Get
* client:
* type: azure:keyvault:AccessPolicy
* properties:
* keyVaultId: ${exampleKeyVault.id}
* tenantId: ${current.tenantId}
* objectId: ${current.objectId}
* keyPermissions:
* - Get
* - Create
* - Delete
* - List
* - Restore
* - Recover
* - UnwrapKey
* - WrapKey
* - Purge
* - Encrypt
* - Decrypt
* - Sign
* - Verify
* - GetRotationPolicy
* secretPermissions:
* - Get
* exampleKey:
* type: azure:keyvault:Key
* name: example
* properties:
* name: exampleKVkey
* keyVaultId: ${exampleKeyVault.id}
* keyType: RSA
* keySize: 2048
* keyOpts:
* - decrypt
* - encrypt
* - sign
* - unwrapKey
* - verify
* - wrapKey
* exampleConfigurationStore:
* type: azure:appconfiguration:ConfigurationStore
* name: example
* properties:
* name: appConf2
* resourceGroupName: ${example.name}
* location: ${example.location}
* sku: standard
* localAuthEnabled: true
* publicNetworkAccess: Enabled
* purgeProtectionEnabled: false
* softDeleteRetentionDays: 1
* identity:
* type: UserAssigned
* identityIds:
* - ${exampleUserAssignedIdentity.id}
* encryption:
* keyVaultKeyIdentifier: ${exampleKey.id}
* identityClientId: ${exampleUserAssignedIdentity.clientId}
* replicas:
* - name: replica1
* location: West US
* tags:
* environment: development
* variables:
* current:
* fn::invoke:
* Function: azure:core:getClientConfig
* Arguments: {}
* ```
*
* ## Import
* App Configurations can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:appconfiguration/configurationStore:ConfigurationStore appconf /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/resourceGroup1/providers/Microsoft.AppConfiguration/configurationStores/appConf1
* ```
* @property encryption An `encryption` block as defined below.
* @property identity An `identity` block as defined below.
* > **NOTE:** Azure does not allow a downgrade from `standard` to `free`.
* @property localAuthEnabled Whether local authentication methods is enabled. Defaults to `true`.
* @property location Specifies the supported Azure location where the resource exists. Changing this forces a new resource to be created.
* @property name Specifies the name of the App Configuration. Changing this forces a new resource to be created.
* @property publicNetworkAccess The Public Network Access setting of the App Configuration. Possible values are `Enabled` and `Disabled`.
* > **NOTE:** If `public_network_access` is not specified, the App Configuration will be created as `Automatic`. However, once a different value is defined, can not be set again as automatic.
* @property purgeProtectionEnabled Whether Purge Protection is enabled. This field only works for `standard` sku. Defaults to `false`.
* !> **Note:** Once Purge Protection has been enabled it's not possible to disable it. Deleting the App Configuration with Purge Protection enabled will schedule the App Configuration to be deleted (which will happen by Azure in the configured number of days).
* @property replicas One or more `replica` blocks as defined below.
* @property resourceGroupName The name of the resource group in which to create the App Configuration. Changing this forces a new resource to be created.
* @property sku The SKU name of the App Configuration. Possible values are `free` and `standard`. Defaults to `free`.
* @property softDeleteRetentionDays The number of days that items should be retained for once soft-deleted. This field only works for `standard` sku. This value can be between `1` and `7` days. Defaults to `7`. Changing this forces a new resource to be created.
* > **Note:** If Purge Protection is enabled, this field can only be configured one time and cannot be updated.
* @property tags A mapping of tags to assign to the resource.
*/
public data class ConfigurationStoreArgs(
public val encryption: Output? = null,
public val identity: Output? = null,
public val localAuthEnabled: Output? = null,
public val location: Output? = null,
public val name: Output? = null,
public val publicNetworkAccess: Output? = null,
public val purgeProtectionEnabled: Output? = null,
public val replicas: Output>? = null,
public val resourceGroupName: Output? = null,
public val sku: Output? = null,
public val softDeleteRetentionDays: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy