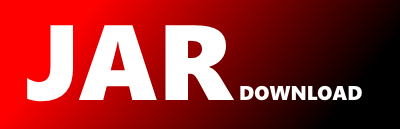
com.pulumi.azure.appservice.kotlin.SourceControlArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin
import com.pulumi.azure.appservice.SourceControlArgs.builder
import com.pulumi.azure.appservice.kotlin.inputs.SourceControlGithubActionConfigurationArgs
import com.pulumi.azure.appservice.kotlin.inputs.SourceControlGithubActionConfigurationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
* Manages an App Service Web App or Function App Source Control Configuration.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleServicePlan = new azure.appservice.ServicePlan("example", {
* name: "example",
* resourceGroupName: example.name,
* location: example.location,
* osType: "Linux",
* skuName: "P1v2",
* });
* const exampleLinuxWebApp = new azure.appservice.LinuxWebApp("example", {
* name: "example",
* resourceGroupName: example.name,
* location: exampleServicePlan.location,
* servicePlanId: exampleServicePlan.id,
* siteConfig: {},
* });
* const exampleSourceControl = new azure.appservice.SourceControl("example", {
* appId: exampleLinuxWebApp.id,
* repoUrl: "https://github.com/Azure-Samples/python-docs-hello-world",
* branch: "master",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_service_plan = azure.appservice.ServicePlan("example",
* name="example",
* resource_group_name=example.name,
* location=example.location,
* os_type="Linux",
* sku_name="P1v2")
* example_linux_web_app = azure.appservice.LinuxWebApp("example",
* name="example",
* resource_group_name=example.name,
* location=example_service_plan.location,
* service_plan_id=example_service_plan.id,
* site_config=azure.appservice.LinuxWebAppSiteConfigArgs())
* example_source_control = azure.appservice.SourceControl("example",
* app_id=example_linux_web_app.id,
* repo_url="https://github.com/Azure-Samples/python-docs-hello-world",
* branch="master")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleServicePlan = new Azure.AppService.ServicePlan("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* Location = example.Location,
* OsType = "Linux",
* SkuName = "P1v2",
* });
* var exampleLinuxWebApp = new Azure.AppService.LinuxWebApp("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* Location = exampleServicePlan.Location,
* ServicePlanId = exampleServicePlan.Id,
* SiteConfig = null,
* });
* var exampleSourceControl = new Azure.AppService.SourceControl("example", new()
* {
* AppId = exampleLinuxWebApp.Id,
* RepoUrl = "https://github.com/Azure-Samples/python-docs-hello-world",
* Branch = "master",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/appservice"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleServicePlan, err := appservice.NewServicePlan(ctx, "example", &appservice.ServicePlanArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* OsType: pulumi.String("Linux"),
* SkuName: pulumi.String("P1v2"),
* })
* if err != nil {
* return err
* }
* exampleLinuxWebApp, err := appservice.NewLinuxWebApp(ctx, "example", &appservice.LinuxWebAppArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* Location: exampleServicePlan.Location,
* ServicePlanId: exampleServicePlan.ID(),
* SiteConfig: nil,
* })
* if err != nil {
* return err
* }
* _, err = appservice.NewSourceControl(ctx, "example", &appservice.SourceControlArgs{
* AppId: exampleLinuxWebApp.ID(),
* RepoUrl: pulumi.String("https://github.com/Azure-Samples/python-docs-hello-world"),
* Branch: pulumi.String("master"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.appservice.ServicePlan;
* import com.pulumi.azure.appservice.ServicePlanArgs;
* import com.pulumi.azure.appservice.LinuxWebApp;
* import com.pulumi.azure.appservice.LinuxWebAppArgs;
* import com.pulumi.azure.appservice.inputs.LinuxWebAppSiteConfigArgs;
* import com.pulumi.azure.appservice.SourceControl;
* import com.pulumi.azure.appservice.SourceControlArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleServicePlan = new ServicePlan("exampleServicePlan", ServicePlanArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .location(example.location())
* .osType("Linux")
* .skuName("P1v2")
* .build());
* var exampleLinuxWebApp = new LinuxWebApp("exampleLinuxWebApp", LinuxWebAppArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .location(exampleServicePlan.location())
* .servicePlanId(exampleServicePlan.id())
* .siteConfig()
* .build());
* var exampleSourceControl = new SourceControl("exampleSourceControl", SourceControlArgs.builder()
* .appId(exampleLinuxWebApp.id())
* .repoUrl("https://github.com/Azure-Samples/python-docs-hello-world")
* .branch("master")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleServicePlan:
* type: azure:appservice:ServicePlan
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* location: ${example.location}
* osType: Linux
* skuName: P1v2
* exampleLinuxWebApp:
* type: azure:appservice:LinuxWebApp
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* location: ${exampleServicePlan.location}
* servicePlanId: ${exampleServicePlan.id}
* siteConfig: {}
* exampleSourceControl:
* type: azure:appservice:SourceControl
* name: example
* properties:
* appId: ${exampleLinuxWebApp.id}
* repoUrl: https://github.com/Azure-Samples/python-docs-hello-world
* branch: master
* ```
*
* ## Import
* App Service Source Controls can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:appservice/sourceControl:SourceControl example /subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/resGroup1/providers/Microsoft.Web/sites/site1
* ```
* @property appId The ID of the Windows or Linux Web App. Changing this forces a new resource to be created.
* > **NOTE:** Function apps are not supported at this time.
* @property branch The branch name to use for deployments. Changing this forces a new resource to be created.
* @property githubActionConfiguration A `github_action_configuration` block as defined below. Changing this forces a new resource to be created.
* @property repoUrl The URL for the repository. Changing this forces a new resource to be created.
* @property rollbackEnabled Should the Deployment Rollback be enabled? Defaults to `false`. Changing this forces a new resource to be created.
* > **NOTE:** Azure can typically set this value automatically based on the `repo_url` value.
* @property useLocalGit Should the App use local Git configuration. Changing this forces a new resource to be created.
* @property useManualIntegration Should code be deployed manually. Set to `false` to enable continuous integration, such as webhooks into online repos such as GitHub. Defaults to `false`. Changing this forces a new resource to be created.
* @property useMercurial The repository specified is Mercurial. Defaults to `false`. Changing this forces a new resource to be created.
*/
public data class SourceControlArgs(
public val appId: Output? = null,
public val branch: Output? = null,
public val githubActionConfiguration: Output? = null,
public val repoUrl: Output? = null,
public val rollbackEnabled: Output? = null,
public val useLocalGit: Output? = null,
public val useManualIntegration: Output? = null,
public val useMercurial: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appservice.SourceControlArgs =
com.pulumi.azure.appservice.SourceControlArgs.builder()
.appId(appId?.applyValue({ args0 -> args0 }))
.branch(branch?.applyValue({ args0 -> args0 }))
.githubActionConfiguration(
githubActionConfiguration?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
)
.repoUrl(repoUrl?.applyValue({ args0 -> args0 }))
.rollbackEnabled(rollbackEnabled?.applyValue({ args0 -> args0 }))
.useLocalGit(useLocalGit?.applyValue({ args0 -> args0 }))
.useManualIntegration(useManualIntegration?.applyValue({ args0 -> args0 }))
.useMercurial(useMercurial?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [SourceControlArgs].
*/
@PulumiTagMarker
public class SourceControlArgsBuilder internal constructor() {
private var appId: Output? = null
private var branch: Output? = null
private var githubActionConfiguration: Output? = null
private var repoUrl: Output? = null
private var rollbackEnabled: Output? = null
private var useLocalGit: Output? = null
private var useManualIntegration: Output? = null
private var useMercurial: Output? = null
/**
* @param value The ID of the Windows or Linux Web App. Changing this forces a new resource to be created.
* > **NOTE:** Function apps are not supported at this time.
*/
@JvmName("smwtwhdedlyrodht")
public suspend fun appId(`value`: Output) {
this.appId = value
}
/**
* @param value The branch name to use for deployments. Changing this forces a new resource to be created.
*/
@JvmName("upelfdfbvtgtikuj")
public suspend fun branch(`value`: Output) {
this.branch = value
}
/**
* @param value A `github_action_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("ytptpcdgmcjwgorx")
public suspend
fun githubActionConfiguration(`value`: Output) {
this.githubActionConfiguration = value
}
/**
* @param value The URL for the repository. Changing this forces a new resource to be created.
*/
@JvmName("boeepqgetgfwntif")
public suspend fun repoUrl(`value`: Output) {
this.repoUrl = value
}
/**
* @param value Should the Deployment Rollback be enabled? Defaults to `false`. Changing this forces a new resource to be created.
* > **NOTE:** Azure can typically set this value automatically based on the `repo_url` value.
*/
@JvmName("rdgvmxjfafikuqvu")
public suspend fun rollbackEnabled(`value`: Output) {
this.rollbackEnabled = value
}
/**
* @param value Should the App use local Git configuration. Changing this forces a new resource to be created.
*/
@JvmName("qexmnpfqeivmtofy")
public suspend fun useLocalGit(`value`: Output) {
this.useLocalGit = value
}
/**
* @param value Should code be deployed manually. Set to `false` to enable continuous integration, such as webhooks into online repos such as GitHub. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("gessperlfupxnebw")
public suspend fun useManualIntegration(`value`: Output) {
this.useManualIntegration = value
}
/**
* @param value The repository specified is Mercurial. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("mxmvgdvuyomnbiru")
public suspend fun useMercurial(`value`: Output) {
this.useMercurial = value
}
/**
* @param value The ID of the Windows or Linux Web App. Changing this forces a new resource to be created.
* > **NOTE:** Function apps are not supported at this time.
*/
@JvmName("dfnixbrtvapvvxwm")
public suspend fun appId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.appId = mapped
}
/**
* @param value The branch name to use for deployments. Changing this forces a new resource to be created.
*/
@JvmName("fkkcdcdfoignuwmg")
public suspend fun branch(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.branch = mapped
}
/**
* @param value A `github_action_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("wrihxekmvuhaojgp")
public suspend
fun githubActionConfiguration(`value`: SourceControlGithubActionConfigurationArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.githubActionConfiguration = mapped
}
/**
* @param argument A `github_action_configuration` block as defined below. Changing this forces a new resource to be created.
*/
@JvmName("taerjvfqikjdsuey")
public suspend
fun githubActionConfiguration(argument: suspend SourceControlGithubActionConfigurationArgsBuilder.() -> Unit) {
val toBeMapped = SourceControlGithubActionConfigurationArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.githubActionConfiguration = mapped
}
/**
* @param value The URL for the repository. Changing this forces a new resource to be created.
*/
@JvmName("tnximvpstnpuilsl")
public suspend fun repoUrl(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.repoUrl = mapped
}
/**
* @param value Should the Deployment Rollback be enabled? Defaults to `false`. Changing this forces a new resource to be created.
* > **NOTE:** Azure can typically set this value automatically based on the `repo_url` value.
*/
@JvmName("bewkgairpatltqtx")
public suspend fun rollbackEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.rollbackEnabled = mapped
}
/**
* @param value Should the App use local Git configuration. Changing this forces a new resource to be created.
*/
@JvmName("asegtakstjfkbofo")
public suspend fun useLocalGit(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useLocalGit = mapped
}
/**
* @param value Should code be deployed manually. Set to `false` to enable continuous integration, such as webhooks into online repos such as GitHub. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("tqxsborblpihiced")
public suspend fun useManualIntegration(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useManualIntegration = mapped
}
/**
* @param value The repository specified is Mercurial. Defaults to `false`. Changing this forces a new resource to be created.
*/
@JvmName("jruxcpgqlkgxowef")
public suspend fun useMercurial(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.useMercurial = mapped
}
internal fun build(): SourceControlArgs = SourceControlArgs(
appId = appId,
branch = branch,
githubActionConfiguration = githubActionConfiguration,
repoUrl = repoUrl,
rollbackEnabled = rollbackEnabled,
useLocalGit = useLocalGit,
useManualIntegration = useManualIntegration,
useMercurial = useMercurial,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy