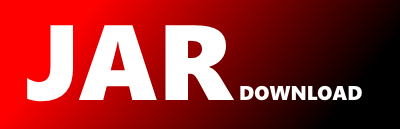
com.pulumi.azure.appservice.kotlin.inputs.FunctionAppSlotSiteConfigScmIpRestrictionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.FunctionAppSlotSiteConfigScmIpRestrictionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property action Allow or Deny access for this IP range. Defaults to `Allow`.
* @property headers The `headers` block for this specific `scm_ip_restriction` as defined below.
* @property ipAddress The IP Address used for this IP Restriction in CIDR notation.
* @property name The name for this IP Restriction.
* @property priority The priority for this IP Restriction. Restrictions are enforced in priority order. By default, priority is set to 65000 if not specified.
* @property serviceTag The Service Tag used for this IP Restriction.
* @property virtualNetworkSubnetId The Virtual Network Subnet ID used for this IP Restriction.
* > **NOTE:** One of either `ip_address`, `service_tag` or `virtual_network_subnet_id` must be specified
*/
public data class FunctionAppSlotSiteConfigScmIpRestrictionArgs(
public val action: Output? = null,
public val headers: Output? = null,
public val ipAddress: Output? = null,
public val name: Output? = null,
public val priority: Output? = null,
public val serviceTag: Output? = null,
public val virtualNetworkSubnetId: Output? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.appservice.inputs.FunctionAppSlotSiteConfigScmIpRestrictionArgs =
com.pulumi.azure.appservice.inputs.FunctionAppSlotSiteConfigScmIpRestrictionArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.headers(headers?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ipAddress(ipAddress?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.priority(priority?.applyValue({ args0 -> args0 }))
.serviceTag(serviceTag?.applyValue({ args0 -> args0 }))
.virtualNetworkSubnetId(virtualNetworkSubnetId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FunctionAppSlotSiteConfigScmIpRestrictionArgs].
*/
@PulumiTagMarker
public class FunctionAppSlotSiteConfigScmIpRestrictionArgsBuilder internal constructor() {
private var action: Output? = null
private var headers: Output? = null
private var ipAddress: Output? = null
private var name: Output? = null
private var priority: Output? = null
private var serviceTag: Output? = null
private var virtualNetworkSubnetId: Output? = null
/**
* @param value Allow or Deny access for this IP range. Defaults to `Allow`.
*/
@JvmName("grtcyanxlrkrmmtk")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value The `headers` block for this specific `scm_ip_restriction` as defined below.
*/
@JvmName("fbdcjqbmfbvpuuqf")
public suspend
fun headers(`value`: Output) {
this.headers = value
}
/**
* @param value The IP Address used for this IP Restriction in CIDR notation.
*/
@JvmName("uvsqrapcjdsgkjcf")
public suspend fun ipAddress(`value`: Output) {
this.ipAddress = value
}
/**
* @param value The name for this IP Restriction.
*/
@JvmName("bjbpnbdhjhldkray")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The priority for this IP Restriction. Restrictions are enforced in priority order. By default, priority is set to 65000 if not specified.
*/
@JvmName("qsphabmpmybxipmw")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value The Service Tag used for this IP Restriction.
*/
@JvmName("pktflliynduvqqox")
public suspend fun serviceTag(`value`: Output) {
this.serviceTag = value
}
/**
* @param value The Virtual Network Subnet ID used for this IP Restriction.
* > **NOTE:** One of either `ip_address`, `service_tag` or `virtual_network_subnet_id` must be specified
*/
@JvmName("wknknbaqusqlwwkr")
public suspend fun virtualNetworkSubnetId(`value`: Output) {
this.virtualNetworkSubnetId = value
}
/**
* @param value Allow or Deny access for this IP range. Defaults to `Allow`.
*/
@JvmName("ugtwkwiwipdinokr")
public suspend fun action(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value The `headers` block for this specific `scm_ip_restriction` as defined below.
*/
@JvmName("cbasposjduymumhk")
public suspend fun headers(`value`: FunctionAppSlotSiteConfigScmIpRestrictionHeadersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.headers = mapped
}
/**
* @param argument The `headers` block for this specific `scm_ip_restriction` as defined below.
*/
@JvmName("pqslqfabtadteddn")
public suspend
fun headers(argument: suspend FunctionAppSlotSiteConfigScmIpRestrictionHeadersArgsBuilder.() -> Unit) {
val toBeMapped = FunctionAppSlotSiteConfigScmIpRestrictionHeadersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.headers = mapped
}
/**
* @param value The IP Address used for this IP Restriction in CIDR notation.
*/
@JvmName("rnperqvmbutttvuw")
public suspend fun ipAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipAddress = mapped
}
/**
* @param value The name for this IP Restriction.
*/
@JvmName("tbhaxajsrswqdhvn")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The priority for this IP Restriction. Restrictions are enforced in priority order. By default, priority is set to 65000 if not specified.
*/
@JvmName("jjbloykbjbpewybk")
public suspend fun priority(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.priority = mapped
}
/**
* @param value The Service Tag used for this IP Restriction.
*/
@JvmName("rmotlvxfomsyaufb")
public suspend fun serviceTag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceTag = mapped
}
/**
* @param value The Virtual Network Subnet ID used for this IP Restriction.
* > **NOTE:** One of either `ip_address`, `service_tag` or `virtual_network_subnet_id` must be specified
*/
@JvmName("xamnsnhaktrobfpr")
public suspend fun virtualNetworkSubnetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.virtualNetworkSubnetId = mapped
}
internal fun build(): FunctionAppSlotSiteConfigScmIpRestrictionArgs =
FunctionAppSlotSiteConfigScmIpRestrictionArgs(
action = action,
headers = headers,
ipAddress = ipAddress,
name = name,
priority = priority,
serviceTag = serviceTag,
virtualNetworkSubnetId = virtualNetworkSubnetId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy