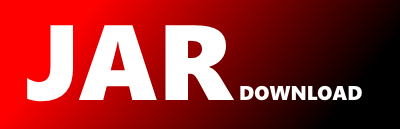
com.pulumi.azure.appservice.kotlin.inputs.LinuxFunctionAppAuthSettingsV2CustomOidcV2Args.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.LinuxFunctionAppAuthSettingsV2CustomOidcV2Args.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property authorisationEndpoint The endpoint to make the Authorisation Request as supplied by `openid_configuration_endpoint` response.
* @property certificationUri The endpoint that provides the keys necessary to validate the token as supplied by `openid_configuration_endpoint` response.
* @property clientCredentialMethod The Client Credential Method used.
* @property clientId The ID of the Client to use to authenticate with the Custom OIDC.
* @property clientSecretSettingName The App Setting name that contains the secret for this Custom OIDC Client. This is generated from `name` above and suffixed with `_PROVIDER_AUTHENTICATION_SECRET`.
* @property issuerEndpoint The endpoint that issued the Token as supplied by `openid_configuration_endpoint` response.
* @property name The name of the Custom OIDC Authentication Provider.
* > **NOTE:** An `app_setting` matching this value in upper case with the suffix of `_PROVIDER_AUTHENTICATION_SECRET` is required. e.g. `MYOIDC_PROVIDER_AUTHENTICATION_SECRET` for a value of `myoidc`.
* @property nameClaimType The name of the claim that contains the users name.
* @property openidConfigurationEndpoint The app setting name that contains the `client_secret` value used for the Custom OIDC Login.
* @property scopes The list of the scopes that should be requested while authenticating.
* @property tokenEndpoint The endpoint used to request a Token as supplied by `openid_configuration_endpoint` response.
*/
public data class LinuxFunctionAppAuthSettingsV2CustomOidcV2Args(
public val authorisationEndpoint: Output? = null,
public val certificationUri: Output? = null,
public val clientCredentialMethod: Output? = null,
public val clientId: Output,
public val clientSecretSettingName: Output? = null,
public val issuerEndpoint: Output? = null,
public val name: Output,
public val nameClaimType: Output? = null,
public val openidConfigurationEndpoint: Output,
public val scopes: Output>? = null,
public val tokenEndpoint: Output? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.appservice.inputs.LinuxFunctionAppAuthSettingsV2CustomOidcV2Args =
com.pulumi.azure.appservice.inputs.LinuxFunctionAppAuthSettingsV2CustomOidcV2Args.builder()
.authorisationEndpoint(authorisationEndpoint?.applyValue({ args0 -> args0 }))
.certificationUri(certificationUri?.applyValue({ args0 -> args0 }))
.clientCredentialMethod(clientCredentialMethod?.applyValue({ args0 -> args0 }))
.clientId(clientId.applyValue({ args0 -> args0 }))
.clientSecretSettingName(clientSecretSettingName?.applyValue({ args0 -> args0 }))
.issuerEndpoint(issuerEndpoint?.applyValue({ args0 -> args0 }))
.name(name.applyValue({ args0 -> args0 }))
.nameClaimType(nameClaimType?.applyValue({ args0 -> args0 }))
.openidConfigurationEndpoint(openidConfigurationEndpoint.applyValue({ args0 -> args0 }))
.scopes(scopes?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.tokenEndpoint(tokenEndpoint?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LinuxFunctionAppAuthSettingsV2CustomOidcV2Args].
*/
@PulumiTagMarker
public class LinuxFunctionAppAuthSettingsV2CustomOidcV2ArgsBuilder internal constructor() {
private var authorisationEndpoint: Output? = null
private var certificationUri: Output? = null
private var clientCredentialMethod: Output? = null
private var clientId: Output? = null
private var clientSecretSettingName: Output? = null
private var issuerEndpoint: Output? = null
private var name: Output? = null
private var nameClaimType: Output? = null
private var openidConfigurationEndpoint: Output? = null
private var scopes: Output>? = null
private var tokenEndpoint: Output? = null
/**
* @param value The endpoint to make the Authorisation Request as supplied by `openid_configuration_endpoint` response.
*/
@JvmName("viykvanwjdcowfua")
public suspend fun authorisationEndpoint(`value`: Output) {
this.authorisationEndpoint = value
}
/**
* @param value The endpoint that provides the keys necessary to validate the token as supplied by `openid_configuration_endpoint` response.
*/
@JvmName("yhfcsliorrccgkse")
public suspend fun certificationUri(`value`: Output) {
this.certificationUri = value
}
/**
* @param value The Client Credential Method used.
*/
@JvmName("qqduaosrkrbdddkd")
public suspend fun clientCredentialMethod(`value`: Output) {
this.clientCredentialMethod = value
}
/**
* @param value The ID of the Client to use to authenticate with the Custom OIDC.
*/
@JvmName("verohutqkwrxrfmh")
public suspend fun clientId(`value`: Output) {
this.clientId = value
}
/**
* @param value The App Setting name that contains the secret for this Custom OIDC Client. This is generated from `name` above and suffixed with `_PROVIDER_AUTHENTICATION_SECRET`.
*/
@JvmName("fhwebhvhevvcjljj")
public suspend fun clientSecretSettingName(`value`: Output) {
this.clientSecretSettingName = value
}
/**
* @param value The endpoint that issued the Token as supplied by `openid_configuration_endpoint` response.
*/
@JvmName("usmxsulyvhitqjdx")
public suspend fun issuerEndpoint(`value`: Output) {
this.issuerEndpoint = value
}
/**
* @param value The name of the Custom OIDC Authentication Provider.
* > **NOTE:** An `app_setting` matching this value in upper case with the suffix of `_PROVIDER_AUTHENTICATION_SECRET` is required. e.g. `MYOIDC_PROVIDER_AUTHENTICATION_SECRET` for a value of `myoidc`.
*/
@JvmName("euxkysktecnkeamd")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The name of the claim that contains the users name.
*/
@JvmName("pkooeyumehbldrqg")
public suspend fun nameClaimType(`value`: Output) {
this.nameClaimType = value
}
/**
* @param value The app setting name that contains the `client_secret` value used for the Custom OIDC Login.
*/
@JvmName("pgchdkhclpieghjq")
public suspend fun openidConfigurationEndpoint(`value`: Output) {
this.openidConfigurationEndpoint = value
}
/**
* @param value The list of the scopes that should be requested while authenticating.
*/
@JvmName("deesqtsabidncpqc")
public suspend fun scopes(`value`: Output>) {
this.scopes = value
}
@JvmName("ybamnxchfxsywdyv")
public suspend fun scopes(vararg values: Output) {
this.scopes = Output.all(values.asList())
}
/**
* @param values The list of the scopes that should be requested while authenticating.
*/
@JvmName("dsfmnolugkkcxpvi")
public suspend fun scopes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy