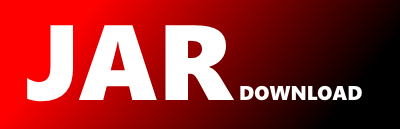
com.pulumi.azure.appservice.kotlin.inputs.LinuxFunctionAppBackupScheduleArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.LinuxFunctionAppBackupScheduleArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property frequencyInterval How often the backup should be executed (e.g. for weekly backup, this should be set to `7` and `frequency_unit` should be set to `Day`).
* > **NOTE:** Not all intervals are supported on all Linux Function App SKUs. Please refer to the official documentation for appropriate values.
* @property frequencyUnit The unit of time for how often the backup should take place. Possible values include: `Day` and `Hour`.
* @property keepAtLeastOneBackup Should the service keep at least one backup, regardless of age of backup. Defaults to `false`.
* @property lastExecutionTime The time the backup was last attempted.
* @property retentionPeriodDays After how many days backups should be deleted. Defaults to `30`.
* @property startTime When the schedule should start working in RFC-3339 format.
*/
public data class LinuxFunctionAppBackupScheduleArgs(
public val frequencyInterval: Output,
public val frequencyUnit: Output,
public val keepAtLeastOneBackup: Output? = null,
public val lastExecutionTime: Output? = null,
public val retentionPeriodDays: Output? = null,
public val startTime: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.appservice.inputs.LinuxFunctionAppBackupScheduleArgs =
com.pulumi.azure.appservice.inputs.LinuxFunctionAppBackupScheduleArgs.builder()
.frequencyInterval(frequencyInterval.applyValue({ args0 -> args0 }))
.frequencyUnit(frequencyUnit.applyValue({ args0 -> args0 }))
.keepAtLeastOneBackup(keepAtLeastOneBackup?.applyValue({ args0 -> args0 }))
.lastExecutionTime(lastExecutionTime?.applyValue({ args0 -> args0 }))
.retentionPeriodDays(retentionPeriodDays?.applyValue({ args0 -> args0 }))
.startTime(startTime?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LinuxFunctionAppBackupScheduleArgs].
*/
@PulumiTagMarker
public class LinuxFunctionAppBackupScheduleArgsBuilder internal constructor() {
private var frequencyInterval: Output? = null
private var frequencyUnit: Output? = null
private var keepAtLeastOneBackup: Output? = null
private var lastExecutionTime: Output? = null
private var retentionPeriodDays: Output? = null
private var startTime: Output? = null
/**
* @param value How often the backup should be executed (e.g. for weekly backup, this should be set to `7` and `frequency_unit` should be set to `Day`).
* > **NOTE:** Not all intervals are supported on all Linux Function App SKUs. Please refer to the official documentation for appropriate values.
*/
@JvmName("hbhucerjjhgscsoe")
public suspend fun frequencyInterval(`value`: Output) {
this.frequencyInterval = value
}
/**
* @param value The unit of time for how often the backup should take place. Possible values include: `Day` and `Hour`.
*/
@JvmName("ngebvqoeopyalqnq")
public suspend fun frequencyUnit(`value`: Output) {
this.frequencyUnit = value
}
/**
* @param value Should the service keep at least one backup, regardless of age of backup. Defaults to `false`.
*/
@JvmName("ialpxviqrgucsioc")
public suspend fun keepAtLeastOneBackup(`value`: Output) {
this.keepAtLeastOneBackup = value
}
/**
* @param value The time the backup was last attempted.
*/
@JvmName("nsqplecbkxinyqpo")
public suspend fun lastExecutionTime(`value`: Output) {
this.lastExecutionTime = value
}
/**
* @param value After how many days backups should be deleted. Defaults to `30`.
*/
@JvmName("yddadiexsiimxktq")
public suspend fun retentionPeriodDays(`value`: Output) {
this.retentionPeriodDays = value
}
/**
* @param value When the schedule should start working in RFC-3339 format.
*/
@JvmName("bgskaomyauywnphf")
public suspend fun startTime(`value`: Output) {
this.startTime = value
}
/**
* @param value How often the backup should be executed (e.g. for weekly backup, this should be set to `7` and `frequency_unit` should be set to `Day`).
* > **NOTE:** Not all intervals are supported on all Linux Function App SKUs. Please refer to the official documentation for appropriate values.
*/
@JvmName("eyksqagfslmccfmk")
public suspend fun frequencyInterval(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.frequencyInterval = mapped
}
/**
* @param value The unit of time for how often the backup should take place. Possible values include: `Day` and `Hour`.
*/
@JvmName("qheithsfvcouquga")
public suspend fun frequencyUnit(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.frequencyUnit = mapped
}
/**
* @param value Should the service keep at least one backup, regardless of age of backup. Defaults to `false`.
*/
@JvmName("fdimppjapjnakviu")
public suspend fun keepAtLeastOneBackup(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.keepAtLeastOneBackup = mapped
}
/**
* @param value The time the backup was last attempted.
*/
@JvmName("ihdtapieicwvxhbs")
public suspend fun lastExecutionTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.lastExecutionTime = mapped
}
/**
* @param value After how many days backups should be deleted. Defaults to `30`.
*/
@JvmName("buvdjhohgdhubkxa")
public suspend fun retentionPeriodDays(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retentionPeriodDays = mapped
}
/**
* @param value When the schedule should start working in RFC-3339 format.
*/
@JvmName("kgonuhruibnktftx")
public suspend fun startTime(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.startTime = mapped
}
internal fun build(): LinuxFunctionAppBackupScheduleArgs = LinuxFunctionAppBackupScheduleArgs(
frequencyInterval = frequencyInterval ?: throw PulumiNullFieldException("frequencyInterval"),
frequencyUnit = frequencyUnit ?: throw PulumiNullFieldException("frequencyUnit"),
keepAtLeastOneBackup = keepAtLeastOneBackup,
lastExecutionTime = lastExecutionTime,
retentionPeriodDays = retentionPeriodDays,
startTime = startTime,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy