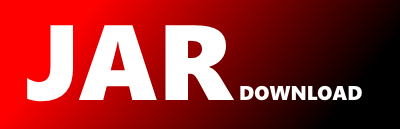
com.pulumi.azure.appservice.kotlin.inputs.LinuxFunctionAppSiteConfigApplicationStackDockerArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.LinuxFunctionAppSiteConfigApplicationStackDockerArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property imageName The name of the Docker image to use.
* @property imageTag The image tag of the image to use.
* @property registryPassword The password for the account to use to connect to the registry.
* > **NOTE:** This value is required if `container_registry_use_managed_identity` is not set to `true`.
* @property registryUrl The URL of the docker registry.
* @property registryUsername The username to use for connections to the registry.
* > **NOTE:** This value is required if `container_registry_use_managed_identity` is not set to `true`.
*/
public data class LinuxFunctionAppSiteConfigApplicationStackDockerArgs(
public val imageName: Output,
public val imageTag: Output,
public val registryPassword: Output? = null,
public val registryUrl: Output,
public val registryUsername: Output? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.appservice.inputs.LinuxFunctionAppSiteConfigApplicationStackDockerArgs =
com.pulumi.azure.appservice.inputs.LinuxFunctionAppSiteConfigApplicationStackDockerArgs.builder()
.imageName(imageName.applyValue({ args0 -> args0 }))
.imageTag(imageTag.applyValue({ args0 -> args0 }))
.registryPassword(registryPassword?.applyValue({ args0 -> args0 }))
.registryUrl(registryUrl.applyValue({ args0 -> args0 }))
.registryUsername(registryUsername?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LinuxFunctionAppSiteConfigApplicationStackDockerArgs].
*/
@PulumiTagMarker
public class LinuxFunctionAppSiteConfigApplicationStackDockerArgsBuilder internal constructor() {
private var imageName: Output? = null
private var imageTag: Output? = null
private var registryPassword: Output? = null
private var registryUrl: Output? = null
private var registryUsername: Output? = null
/**
* @param value The name of the Docker image to use.
*/
@JvmName("andipwskmavfcmva")
public suspend fun imageName(`value`: Output) {
this.imageName = value
}
/**
* @param value The image tag of the image to use.
*/
@JvmName("hvphbqeuafnuxtnk")
public suspend fun imageTag(`value`: Output) {
this.imageTag = value
}
/**
* @param value The password for the account to use to connect to the registry.
* > **NOTE:** This value is required if `container_registry_use_managed_identity` is not set to `true`.
*/
@JvmName("ivgkxphmpfwabfdc")
public suspend fun registryPassword(`value`: Output) {
this.registryPassword = value
}
/**
* @param value The URL of the docker registry.
*/
@JvmName("lnercffpdikyqwyb")
public suspend fun registryUrl(`value`: Output) {
this.registryUrl = value
}
/**
* @param value The username to use for connections to the registry.
* > **NOTE:** This value is required if `container_registry_use_managed_identity` is not set to `true`.
*/
@JvmName("vdeaeojyaatsenrg")
public suspend fun registryUsername(`value`: Output) {
this.registryUsername = value
}
/**
* @param value The name of the Docker image to use.
*/
@JvmName("iayrarfqkpobfwwg")
public suspend fun imageName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.imageName = mapped
}
/**
* @param value The image tag of the image to use.
*/
@JvmName("apjsafwbmpviqakw")
public suspend fun imageTag(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.imageTag = mapped
}
/**
* @param value The password for the account to use to connect to the registry.
* > **NOTE:** This value is required if `container_registry_use_managed_identity` is not set to `true`.
*/
@JvmName("rfmeqvposcqdegvf")
public suspend fun registryPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.registryPassword = mapped
}
/**
* @param value The URL of the docker registry.
*/
@JvmName("qkoobwdmttgtqkkw")
public suspend fun registryUrl(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.registryUrl = mapped
}
/**
* @param value The username to use for connections to the registry.
* > **NOTE:** This value is required if `container_registry_use_managed_identity` is not set to `true`.
*/
@JvmName("qqcoscafyigieqxx")
public suspend fun registryUsername(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.registryUsername = mapped
}
internal fun build(): LinuxFunctionAppSiteConfigApplicationStackDockerArgs =
LinuxFunctionAppSiteConfigApplicationStackDockerArgs(
imageName = imageName ?: throw PulumiNullFieldException("imageName"),
imageTag = imageTag ?: throw PulumiNullFieldException("imageTag"),
registryPassword = registryPassword,
registryUrl = registryUrl ?: throw PulumiNullFieldException("registryUrl"),
registryUsername = registryUsername,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy