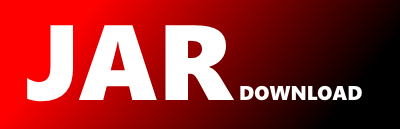
com.pulumi.azure.appservice.kotlin.inputs.WindowsWebAppSlotSiteConfigScmIpRestrictionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.inputs
import com.pulumi.azure.appservice.inputs.WindowsWebAppSlotSiteConfigScmIpRestrictionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property action The action to take. Possible values are `Allow` or `Deny`. Defaults to `Allow`.
* @property description The Description of this IP Restriction.
* @property headers A `headers` block as defined above.
* @property ipAddress The CIDR notation of the IP or IP Range to match. For example: `10.0.0.0/24` or `192.168.10.1/32`
* @property name The name which should be used for this `ip_restriction`.
* @property priority The priority value of this `ip_restriction`. Defaults to `65000`.
* @property serviceTag The Service Tag used for this IP Restriction.
* @property virtualNetworkSubnetId The Virtual Network Subnet ID used for this IP Restriction.
* > **NOTE:** One and only one of `ip_address`, `service_tag` or `virtual_network_subnet_id` must be specified.
*/
public data class WindowsWebAppSlotSiteConfigScmIpRestrictionArgs(
public val action: Output? = null,
public val description: Output? = null,
public val headers: Output? = null,
public val ipAddress: Output? = null,
public val name: Output? = null,
public val priority: Output? = null,
public val serviceTag: Output? = null,
public val virtualNetworkSubnetId: Output? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.appservice.inputs.WindowsWebAppSlotSiteConfigScmIpRestrictionArgs =
com.pulumi.azure.appservice.inputs.WindowsWebAppSlotSiteConfigScmIpRestrictionArgs.builder()
.action(action?.applyValue({ args0 -> args0 }))
.description(description?.applyValue({ args0 -> args0 }))
.headers(headers?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.ipAddress(ipAddress?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.priority(priority?.applyValue({ args0 -> args0 }))
.serviceTag(serviceTag?.applyValue({ args0 -> args0 }))
.virtualNetworkSubnetId(virtualNetworkSubnetId?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [WindowsWebAppSlotSiteConfigScmIpRestrictionArgs].
*/
@PulumiTagMarker
public class WindowsWebAppSlotSiteConfigScmIpRestrictionArgsBuilder internal constructor() {
private var action: Output? = null
private var description: Output? = null
private var headers: Output? = null
private var ipAddress: Output? = null
private var name: Output? = null
private var priority: Output? = null
private var serviceTag: Output? = null
private var virtualNetworkSubnetId: Output? = null
/**
* @param value The action to take. Possible values are `Allow` or `Deny`. Defaults to `Allow`.
*/
@JvmName("hwqqnjnshekyegto")
public suspend fun action(`value`: Output) {
this.action = value
}
/**
* @param value The Description of this IP Restriction.
*/
@JvmName("pggxxgydjygbopax")
public suspend fun description(`value`: Output) {
this.description = value
}
/**
* @param value A `headers` block as defined above.
*/
@JvmName("jylkvrnahexoghaw")
public suspend
fun headers(`value`: Output) {
this.headers = value
}
/**
* @param value The CIDR notation of the IP or IP Range to match. For example: `10.0.0.0/24` or `192.168.10.1/32`
*/
@JvmName("opjpsrkhnuyvbpyj")
public suspend fun ipAddress(`value`: Output) {
this.ipAddress = value
}
/**
* @param value The name which should be used for this `ip_restriction`.
*/
@JvmName("ippoykqtffvjmoca")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value The priority value of this `ip_restriction`. Defaults to `65000`.
*/
@JvmName("suxcflomyatkhejb")
public suspend fun priority(`value`: Output) {
this.priority = value
}
/**
* @param value The Service Tag used for this IP Restriction.
*/
@JvmName("bbttqtedeoyivdoy")
public suspend fun serviceTag(`value`: Output) {
this.serviceTag = value
}
/**
* @param value The Virtual Network Subnet ID used for this IP Restriction.
* > **NOTE:** One and only one of `ip_address`, `service_tag` or `virtual_network_subnet_id` must be specified.
*/
@JvmName("vokwckivpooxrfpy")
public suspend fun virtualNetworkSubnetId(`value`: Output) {
this.virtualNetworkSubnetId = value
}
/**
* @param value The action to take. Possible values are `Allow` or `Deny`. Defaults to `Allow`.
*/
@JvmName("tbtknrsdjighsqyh")
public suspend fun action(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.action = mapped
}
/**
* @param value The Description of this IP Restriction.
*/
@JvmName("hedojntboncrwhhn")
public suspend fun description(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.description = mapped
}
/**
* @param value A `headers` block as defined above.
*/
@JvmName("hbbmcvulakgcrsnn")
public suspend fun headers(`value`: WindowsWebAppSlotSiteConfigScmIpRestrictionHeadersArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.headers = mapped
}
/**
* @param argument A `headers` block as defined above.
*/
@JvmName("ixvfuoyctrebfosd")
public suspend
fun headers(argument: suspend WindowsWebAppSlotSiteConfigScmIpRestrictionHeadersArgsBuilder.() -> Unit) {
val toBeMapped = WindowsWebAppSlotSiteConfigScmIpRestrictionHeadersArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.headers = mapped
}
/**
* @param value The CIDR notation of the IP or IP Range to match. For example: `10.0.0.0/24` or `192.168.10.1/32`
*/
@JvmName("wdbagefxitcrsqqk")
public suspend fun ipAddress(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.ipAddress = mapped
}
/**
* @param value The name which should be used for this `ip_restriction`.
*/
@JvmName("vqufjlpygnrecuqh")
public suspend fun name(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.name = mapped
}
/**
* @param value The priority value of this `ip_restriction`. Defaults to `65000`.
*/
@JvmName("ksrdkqtwywditupx")
public suspend fun priority(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.priority = mapped
}
/**
* @param value The Service Tag used for this IP Restriction.
*/
@JvmName("ydgreiyhhtlehsdk")
public suspend fun serviceTag(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.serviceTag = mapped
}
/**
* @param value The Virtual Network Subnet ID used for this IP Restriction.
* > **NOTE:** One and only one of `ip_address`, `service_tag` or `virtual_network_subnet_id` must be specified.
*/
@JvmName("qiyycvpuxbapcwyk")
public suspend fun virtualNetworkSubnetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.virtualNetworkSubnetId = mapped
}
internal fun build(): WindowsWebAppSlotSiteConfigScmIpRestrictionArgs =
WindowsWebAppSlotSiteConfigScmIpRestrictionArgs(
action = action,
description = description,
headers = headers,
ipAddress = ipAddress,
name = name,
priority = priority,
serviceTag = serviceTag,
virtualNetworkSubnetId = virtualNetworkSubnetId,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy