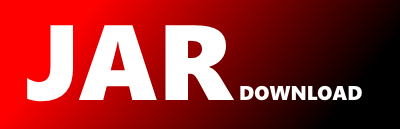
com.pulumi.azure.appservice.kotlin.outputs.GetAppServiceSiteConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property acrUseManagedIdentityCredentials Are Managed Identity Credentials used for Azure Container Registry pull.
* @property acrUserManagedIdentityClientId The User Managed Identity Client Id.
* @property alwaysOn Is the app loaded at all times?
* @property appCommandLine App command line to launch.
* @property cors A `cors` block as defined above.
* @property defaultDocuments The ordering of default documents to load, if an address isn't specified.
* @property dotnetFrameworkVersion The version of the .NET framework's CLR used in this App Service.
* @property ftpsState State of FTP / FTPS service for this AppService.
* @property healthCheckPath The health check path to be pinged by App Service.
* @property http2Enabled Is HTTP2 Enabled on this App Service?
* @property ipRestrictions One or more `ip_restriction` blocks as defined above.
* @property javaContainer The Java Container in use.
* @property javaContainerVersion The version of the Java Container in use.
* @property javaVersion The version of Java in use.
* @property linuxFxVersion Linux App Framework and version for the AppService.
* @property localMysqlEnabled Is "MySQL In App" Enabled? This runs a local MySQL instance with your app and shares resources from the App Service plan.
* @property managedPipelineMode The Managed Pipeline Mode used in this App Service.
* @property minTlsVersion The minimum supported TLS version for this App Service.
* @property numberOfWorkers The scaled number of workers (for per site scaling) of this App Service.
* @property phpVersion The version of PHP used in this App Service.
* @property pythonVersion The version of Python used in this App Service.
* @property remoteDebuggingEnabled Is Remote Debugging Enabled in this App Service?
* @property remoteDebuggingVersion Which version of Visual Studio is the Remote Debugger compatible with?
* @property scmIpRestrictions One or more `scm_ip_restriction` blocks as defined above.
* @property scmType The type of Source Control enabled for this App Service.
* @property scmUseMainIpRestriction IP security restrictions for scm to use main.
* @property use32BitWorkerProcess Does the App Service run in 32 bit mode, rather than 64 bit mode?
* @property vnetRouteAllEnabled (Optional) Should all outbound traffic to have Virtual Network Security Groups and User Defined Routes applied?
* @property websocketsEnabled Are WebSockets enabled for this App Service?
* @property windowsFxVersion Windows Container Docker Image for the AppService.
*/
public data class GetAppServiceSiteConfig(
public val acrUseManagedIdentityCredentials: Boolean,
public val acrUserManagedIdentityClientId: String,
public val alwaysOn: Boolean,
public val appCommandLine: String,
public val cors: List,
public val defaultDocuments: List,
public val dotnetFrameworkVersion: String,
public val ftpsState: String,
public val healthCheckPath: String,
public val http2Enabled: Boolean,
public val ipRestrictions: List,
public val javaContainer: String,
public val javaContainerVersion: String,
public val javaVersion: String,
public val linuxFxVersion: String,
public val localMysqlEnabled: Boolean,
public val managedPipelineMode: String,
public val minTlsVersion: String,
public val numberOfWorkers: Int,
public val phpVersion: String,
public val pythonVersion: String,
public val remoteDebuggingEnabled: Boolean,
public val remoteDebuggingVersion: String,
public val scmIpRestrictions: List,
public val scmType: String,
public val scmUseMainIpRestriction: Boolean,
public val use32BitWorkerProcess: Boolean,
public val vnetRouteAllEnabled: Boolean,
public val websocketsEnabled: Boolean,
public val windowsFxVersion: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.GetAppServiceSiteConfig):
GetAppServiceSiteConfig = GetAppServiceSiteConfig(
acrUseManagedIdentityCredentials = javaType.acrUseManagedIdentityCredentials(),
acrUserManagedIdentityClientId = javaType.acrUserManagedIdentityClientId(),
alwaysOn = javaType.alwaysOn(),
appCommandLine = javaType.appCommandLine(),
cors = javaType.cors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetAppServiceSiteConfigCor.Companion.toKotlin(args0)
})
}),
defaultDocuments = javaType.defaultDocuments().map({ args0 -> args0 }),
dotnetFrameworkVersion = javaType.dotnetFrameworkVersion(),
ftpsState = javaType.ftpsState(),
healthCheckPath = javaType.healthCheckPath(),
http2Enabled = javaType.http2Enabled(),
ipRestrictions = javaType.ipRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetAppServiceSiteConfigIpRestriction.Companion.toKotlin(args0)
})
}),
javaContainer = javaType.javaContainer(),
javaContainerVersion = javaType.javaContainerVersion(),
javaVersion = javaType.javaVersion(),
linuxFxVersion = javaType.linuxFxVersion(),
localMysqlEnabled = javaType.localMysqlEnabled(),
managedPipelineMode = javaType.managedPipelineMode(),
minTlsVersion = javaType.minTlsVersion(),
numberOfWorkers = javaType.numberOfWorkers(),
phpVersion = javaType.phpVersion(),
pythonVersion = javaType.pythonVersion(),
remoteDebuggingEnabled = javaType.remoteDebuggingEnabled(),
remoteDebuggingVersion = javaType.remoteDebuggingVersion(),
scmIpRestrictions = javaType.scmIpRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetAppServiceSiteConfigScmIpRestriction.Companion.toKotlin(args0)
})
}),
scmType = javaType.scmType(),
scmUseMainIpRestriction = javaType.scmUseMainIpRestriction(),
use32BitWorkerProcess = javaType.use32BitWorkerProcess(),
vnetRouteAllEnabled = javaType.vnetRouteAllEnabled(),
websocketsEnabled = javaType.websocketsEnabled(),
windowsFxVersion = javaType.windowsFxVersion(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy