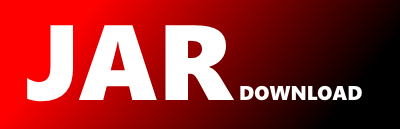
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by getLinuxFunctionApp.
* @property appSettings A map of key-value pairs for [App Settings](https://docs.microsoft.com/azure/azure-functions/functions-app-settings) and custom values.
* @property authSettings A `auth_settings` block as defined below.
* @property authSettingsV2s A `auth_settings_v2` block as defined below.
* @property availability The current availability state. Possible values are `Normal`, `Limited`, and `DisasterRecoveryMode`.
* @property backups A `backup` block as defined below.
* @property builtinLoggingEnabled Is built in logging enabled?
* @property clientCertificateEnabled Are Client Certificates enabled?
* @property clientCertificateExclusionPaths Paths to exclude when using client certificates, separated by ;
* @property clientCertificateMode The mode of the Function App's client certificates requirement for incoming requests.
* @property connectionStrings A `connection_string` blocks as defined below.
* @property contentShareForceDisabled Are the settings for linking the Function App to storage suppressed?
* @property customDomainVerificationId The identifier used by App Service to perform domain ownership verification via DNS TXT record.
* @property dailyMemoryTimeQuota The amount of memory in gigabyte-seconds that your application is allowed to consume per day.
* @property defaultHostname The default hostname of the Linux Function App.
* @property enabled Is this backup job enabled?
* @property ftpPublishBasicAuthenticationEnabled Are the default FTP Basic Authentication publishing credentials enabled.
* @property functionsExtensionVersion The runtime version associated with the Function App.
* @property hostingEnvironmentId The ID of the App Service Environment used by Function App.
* @property httpsOnly Can the Function App only be accessed via HTTPS?
* @property id The provider-assigned unique ID for this managed resource.
* @property identities A `identity` block as defined below.
* @property kind The Kind value for this Linux Function App.
* @property location The Azure Region where the Linux Function App exists.
* @property name The Site Credentials Username used for publishing.
* @property outboundIpAddressLists A list of outbound IP addresses. For example `["52.23.25.3", "52.143.43.12"]`
* @property outboundIpAddresses A comma separated list of outbound IP addresses as a string. For example `52.23.25.3,52.143.43.12`.
* @property possibleOutboundIpAddressLists A list of possible outbound IP addresses, not all of which are necessarily in use. This is a superset of `outbound_ip_address_list`. For example `["52.23.25.3", "52.143.43.12"]`.
* @property possibleOutboundIpAddresses A comma separated list of possible outbound IP addresses as a string. For example `52.23.25.3,52.143.43.12,52.143.43.17`. This is a superset of `outbound_ip_addresses`.
* @property publicNetworkAccessEnabled Is Public Network Access enabled for this Linux Function App.
* @property resourceGroupName
* @property servicePlanId The ID of the App Service Plan within which this Function App has been created.
* @property siteConfigs A `site_config` block as defined below.
* @property siteCredentials A `site_credential` block as defined below.
* @property stickySettings A `sticky_settings` block as defined below.
* @property storageAccountAccessKey The access key used to access the backend storage account for the Function App.
* @property storageAccountName The backend storage account name used by this Function App.
* @property storageKeyVaultSecretId The Key Vault Secret ID, including version, that contains the Connection String to connect to the storage account for this Function App.
* @property storageUsesManagedIdentity Does the Function App use Managed Identity to access the storage account?
* @property tags A mapping of tags which are assigned to the Linux Function App.
* @property usage The current usage state. Possible values are `Normal` and `Exceeded`.
* @property virtualNetworkSubnetId The Virtual Network Subnet ID used for this IP Restriction.
* @property webdeployPublishBasicAuthenticationEnabled Are the default WebDeploy Basic Authentication publishing credentials enabled.
*/
public data class GetLinuxFunctionAppResult(
public val appSettings: Map,
public val authSettings: List,
public val authSettingsV2s: List,
public val availability: String,
public val backups: List,
public val builtinLoggingEnabled: Boolean,
public val clientCertificateEnabled: Boolean,
public val clientCertificateExclusionPaths: String,
public val clientCertificateMode: String,
public val connectionStrings: List,
public val contentShareForceDisabled: Boolean,
public val customDomainVerificationId: String,
public val dailyMemoryTimeQuota: Int,
public val defaultHostname: String,
public val enabled: Boolean,
public val ftpPublishBasicAuthenticationEnabled: Boolean,
public val functionsExtensionVersion: String,
public val hostingEnvironmentId: String,
public val httpsOnly: Boolean,
public val id: String,
public val identities: List,
public val kind: String,
public val location: String,
public val name: String,
public val outboundIpAddressLists: List,
public val outboundIpAddresses: String,
public val possibleOutboundIpAddressLists: List,
public val possibleOutboundIpAddresses: String,
public val publicNetworkAccessEnabled: Boolean,
public val resourceGroupName: String,
public val servicePlanId: String,
public val siteConfigs: List,
public val siteCredentials: List,
public val stickySettings: List,
public val storageAccountAccessKey: String,
public val storageAccountName: String,
public val storageKeyVaultSecretId: String,
public val storageUsesManagedIdentity: Boolean,
public val tags: Map,
public val usage: String,
public val virtualNetworkSubnetId: String,
public val webdeployPublishBasicAuthenticationEnabled: Boolean,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.GetLinuxFunctionAppResult):
GetLinuxFunctionAppResult = GetLinuxFunctionAppResult(
appSettings = javaType.appSettings().map({ args0 -> args0.key.to(args0.value) }).toMap(),
authSettings = javaType.authSettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppAuthSetting.Companion.toKotlin(args0)
})
}),
authSettingsV2s = javaType.authSettingsV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppAuthSettingsV2.Companion.toKotlin(args0)
})
}),
availability = javaType.availability(),
backups = javaType.backups().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppBackup.Companion.toKotlin(args0)
})
}),
builtinLoggingEnabled = javaType.builtinLoggingEnabled(),
clientCertificateEnabled = javaType.clientCertificateEnabled(),
clientCertificateExclusionPaths = javaType.clientCertificateExclusionPaths(),
clientCertificateMode = javaType.clientCertificateMode(),
connectionStrings = javaType.connectionStrings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppConnectionString.Companion.toKotlin(args0)
})
}),
contentShareForceDisabled = javaType.contentShareForceDisabled(),
customDomainVerificationId = javaType.customDomainVerificationId(),
dailyMemoryTimeQuota = javaType.dailyMemoryTimeQuota(),
defaultHostname = javaType.defaultHostname(),
enabled = javaType.enabled(),
ftpPublishBasicAuthenticationEnabled = javaType.ftpPublishBasicAuthenticationEnabled(),
functionsExtensionVersion = javaType.functionsExtensionVersion(),
hostingEnvironmentId = javaType.hostingEnvironmentId(),
httpsOnly = javaType.httpsOnly(),
id = javaType.id(),
identities = javaType.identities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppIdentity.Companion.toKotlin(args0)
})
}),
kind = javaType.kind(),
location = javaType.location(),
name = javaType.name(),
outboundIpAddressLists = javaType.outboundIpAddressLists().map({ args0 -> args0 }),
outboundIpAddresses = javaType.outboundIpAddresses(),
possibleOutboundIpAddressLists = javaType.possibleOutboundIpAddressLists().map({ args0 -> args0 }),
possibleOutboundIpAddresses = javaType.possibleOutboundIpAddresses(),
publicNetworkAccessEnabled = javaType.publicNetworkAccessEnabled(),
resourceGroupName = javaType.resourceGroupName(),
servicePlanId = javaType.servicePlanId(),
siteConfigs = javaType.siteConfigs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppSiteConfig.Companion.toKotlin(args0)
})
}),
siteCredentials = javaType.siteCredentials().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppSiteCredential.Companion.toKotlin(args0)
})
}),
stickySettings = javaType.stickySettings().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetLinuxFunctionAppStickySetting.Companion.toKotlin(args0)
})
}),
storageAccountAccessKey = javaType.storageAccountAccessKey(),
storageAccountName = javaType.storageAccountName(),
storageKeyVaultSecretId = javaType.storageKeyVaultSecretId(),
storageUsesManagedIdentity = javaType.storageUsesManagedIdentity(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
usage = javaType.usage(),
virtualNetworkSubnetId = javaType.virtualNetworkSubnetId(),
webdeployPublishBasicAuthenticationEnabled = javaType.webdeployPublishBasicAuthenticationEnabled(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy