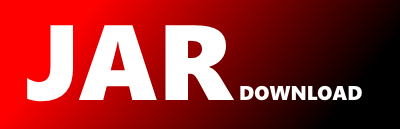
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsFunctionAppSiteConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property alwaysOn Is this Windows Function App Always On?.
* @property apiDefinitionUrl The URL of the API definition that describes this Windows Function App.
* @property apiManagementApiId The ID of the API Management API for this Windows Function App.
* @property appCommandLine The App command line to launch.
* @property appScaleLimit The number of workers this function app can scale out to.
* @property appServiceLogs A `app_service_logs` block as defined above.
* @property applicationInsightsConnectionString The Connection String for linking the Windows Function App to Application Insights.
* @property applicationInsightsKey The Instrumentation Key for connecting the Windows Function App to Application Insights.
* @property applicationStacks A `application_stack` block as defined above.
* @property cors A `cors` block as defined above.
* @property defaultDocuments A list of Default Documents for the Windows Web App.
* @property detailedErrorLoggingEnabled Is detailed error logging enabled?
* @property elasticInstanceMinimum The number of minimum instances for this Windows Function App.
* @property ftpsState State of FTP / FTPS service for this Windows Function App.
* @property healthCheckEvictionTimeInMin The amount of time in minutes that a node can be unhealthy before being removed from the load balancer.
* @property healthCheckPath The path to be checked for this Windows Function App health.
* @property http2Enabled Is the HTTP2 protocol enabled?
* @property ipRestrictionDefaultAction The Default action for traffic that does not match any `ip_restriction` rule.
* @property ipRestrictions One or more `ip_restriction` blocks as defined above.
* @property loadBalancingMode The Site load balancing mode.
* @property managedPipelineMode The Managed pipeline mode.
* @property minimumTlsVersion The minimum version of TLS required for SSL requests.
* @property preWarmedInstanceCount The number of pre-warmed instances for this Windows Function App.
* @property remoteDebuggingEnabled Is Remote Debugging enabled?
* @property remoteDebuggingVersion The Remote Debugging Version.
* @property runtimeScaleMonitoringEnabled Is Scale Monitoring of the Functions Runtime enabled?
* @property scmIpRestrictionDefaultAction The Default action for traffic that does not match any `scm_ip_restriction` rule.
* @property scmIpRestrictions One or more `scm_ip_restriction` blocks as defined above.
* @property scmMinimumTlsVersion The minimum version of TLS required for SSL requests to the SCM site.
* @property scmType The SCM type.
* @property scmUseMainIpRestriction Is the `ip_restriction` configuration used for the SCM?.
* @property use32BitWorker Is the Windows Function App using a 32-bit worker process?
* @property vnetRouteAllEnabled Are all outbound traffic to NAT Gateways, Network Security Groups and User Defined Routes applied?
* @property websocketsEnabled Are Web Sockets enabled?
* @property windowsFxVersion The Windows FX version.
* @property workerCount The number of Workers for this Windows Function App.
*/
public data class GetWindowsFunctionAppSiteConfig(
public val alwaysOn: Boolean,
public val apiDefinitionUrl: String,
public val apiManagementApiId: String,
public val appCommandLine: String,
public val appScaleLimit: Int,
public val appServiceLogs: List,
public val applicationInsightsConnectionString: String,
public val applicationInsightsKey: String,
public val applicationStacks: List,
public val cors: List,
public val defaultDocuments: List,
public val detailedErrorLoggingEnabled: Boolean,
public val elasticInstanceMinimum: Int,
public val ftpsState: String,
public val healthCheckEvictionTimeInMin: Int,
public val healthCheckPath: String,
public val http2Enabled: Boolean,
public val ipRestrictionDefaultAction: String,
public val ipRestrictions: List,
public val loadBalancingMode: String,
public val managedPipelineMode: String,
public val minimumTlsVersion: String,
public val preWarmedInstanceCount: Int,
public val remoteDebuggingEnabled: Boolean,
public val remoteDebuggingVersion: String,
public val runtimeScaleMonitoringEnabled: Boolean,
public val scmIpRestrictionDefaultAction: String,
public val scmIpRestrictions: List,
public val scmMinimumTlsVersion: String,
public val scmType: String,
public val scmUseMainIpRestriction: Boolean,
public val use32BitWorker: Boolean,
public val vnetRouteAllEnabled: Boolean,
public val websocketsEnabled: Boolean,
public val windowsFxVersion: String,
public val workerCount: Int,
) {
public companion object {
public
fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.GetWindowsFunctionAppSiteConfig):
GetWindowsFunctionAppSiteConfig = GetWindowsFunctionAppSiteConfig(
alwaysOn = javaType.alwaysOn(),
apiDefinitionUrl = javaType.apiDefinitionUrl(),
apiManagementApiId = javaType.apiManagementApiId(),
appCommandLine = javaType.appCommandLine(),
appScaleLimit = javaType.appScaleLimit(),
appServiceLogs = javaType.appServiceLogs().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsFunctionAppSiteConfigAppServiceLog.Companion.toKotlin(args0)
})
}),
applicationInsightsConnectionString = javaType.applicationInsightsConnectionString(),
applicationInsightsKey = javaType.applicationInsightsKey(),
applicationStacks = javaType.applicationStacks().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsFunctionAppSiteConfigApplicationStack.Companion.toKotlin(args0)
})
}),
cors = javaType.cors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsFunctionAppSiteConfigCor.Companion.toKotlin(args0)
})
}),
defaultDocuments = javaType.defaultDocuments().map({ args0 -> args0 }),
detailedErrorLoggingEnabled = javaType.detailedErrorLoggingEnabled(),
elasticInstanceMinimum = javaType.elasticInstanceMinimum(),
ftpsState = javaType.ftpsState(),
healthCheckEvictionTimeInMin = javaType.healthCheckEvictionTimeInMin(),
healthCheckPath = javaType.healthCheckPath(),
http2Enabled = javaType.http2Enabled(),
ipRestrictionDefaultAction = javaType.ipRestrictionDefaultAction(),
ipRestrictions = javaType.ipRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsFunctionAppSiteConfigIpRestriction.Companion.toKotlin(args0)
})
}),
loadBalancingMode = javaType.loadBalancingMode(),
managedPipelineMode = javaType.managedPipelineMode(),
minimumTlsVersion = javaType.minimumTlsVersion(),
preWarmedInstanceCount = javaType.preWarmedInstanceCount(),
remoteDebuggingEnabled = javaType.remoteDebuggingEnabled(),
remoteDebuggingVersion = javaType.remoteDebuggingVersion(),
runtimeScaleMonitoringEnabled = javaType.runtimeScaleMonitoringEnabled(),
scmIpRestrictionDefaultAction = javaType.scmIpRestrictionDefaultAction(),
scmIpRestrictions = javaType.scmIpRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsFunctionAppSiteConfigScmIpRestriction.Companion.toKotlin(args0)
})
}),
scmMinimumTlsVersion = javaType.scmMinimumTlsVersion(),
scmType = javaType.scmType(),
scmUseMainIpRestriction = javaType.scmUseMainIpRestriction(),
use32BitWorker = javaType.use32BitWorker(),
vnetRouteAllEnabled = javaType.vnetRouteAllEnabled(),
websocketsEnabled = javaType.websocketsEnabled(),
windowsFxVersion = javaType.windowsFxVersion(),
workerCount = javaType.workerCount(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy