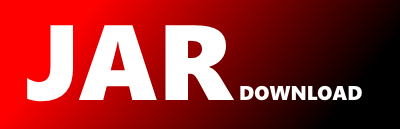
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property activeDirectoryV2s An `active_directory_v2` block as defined below.
* @property appleV2s An `apple_v2` block as defined below.
* @property authEnabled Are the AuthV2 Settings enabled.
* @property azureStaticWebAppV2s An `azure_static_web_app_v2` block as defined below.
* @property configFilePath The path to the App Auth settings.
* @property customOidcV2s Zero or more `custom_oidc_v2` blocks as defined below.
* @property defaultProvider The Default Authentication Provider used when more than one Authentication Provider is configured and the `unauthenticated_action` is set to `RedirectToLoginPage`.
* @property excludedPaths The paths which should be excluded from the `unauthenticated_action` when it is set to `RedirectToLoginPage`.
* @property facebookV2s A `facebook_v2` block as defined below.
* @property forwardProxyConvention The convention used to determine the url of the request made.
* @property forwardProxyCustomHostHeaderName The name of the custom header containing the host of the request.
* @property forwardProxyCustomSchemeHeaderName The name of the custom header containing the scheme of the request.
* @property githubV2s A `github_v2` block as defined below.
* @property googleV2s A `google_v2` block as defined below.
* @property httpRouteApiPrefix The prefix that should precede all the authentication and authorisation paths.
* @property logins A `login` block as defined below.
* @property microsoftV2s A `microsoft_v2` block as defined below.
* @property requireAuthentication Is the authentication flow used for all requests.
* @property requireHttps Is HTTPS required on connections?
* @property runtimeVersion The Runtime Version of the Authentication and Authorisation feature of this App.
* @property twitterV2s A `twitter_v2` block as defined below.
* @property unauthenticatedAction The action to take for requests made without authentication.
*/
public data class GetWindowsWebAppAuthSettingsV2(
public val activeDirectoryV2s: List,
public val appleV2s: List,
public val authEnabled: Boolean,
public val azureStaticWebAppV2s: List,
public val configFilePath: String,
public val customOidcV2s: List,
public val defaultProvider: String,
public val excludedPaths: List,
public val facebookV2s: List,
public val forwardProxyConvention: String,
public val forwardProxyCustomHostHeaderName: String,
public val forwardProxyCustomSchemeHeaderName: String,
public val githubV2s: List,
public val googleV2s: List,
public val httpRouteApiPrefix: String,
public val logins: List,
public val microsoftV2s: List,
public val requireAuthentication: Boolean,
public val requireHttps: Boolean,
public val runtimeVersion: String,
public val twitterV2s: List,
public val unauthenticatedAction: String,
) {
public companion object {
public
fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.GetWindowsWebAppAuthSettingsV2):
GetWindowsWebAppAuthSettingsV2 = GetWindowsWebAppAuthSettingsV2(
activeDirectoryV2s = javaType.activeDirectoryV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2ActiveDirectoryV2.Companion.toKotlin(args0)
})
}),
appleV2s = javaType.appleV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2AppleV2.Companion.toKotlin(args0)
})
}),
authEnabled = javaType.authEnabled(),
azureStaticWebAppV2s = javaType.azureStaticWebAppV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2AzureStaticWebAppV2.Companion.toKotlin(args0)
})
}),
configFilePath = javaType.configFilePath(),
customOidcV2s = javaType.customOidcV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2CustomOidcV2.Companion.toKotlin(args0)
})
}),
defaultProvider = javaType.defaultProvider(),
excludedPaths = javaType.excludedPaths().map({ args0 -> args0 }),
facebookV2s = javaType.facebookV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2FacebookV2.Companion.toKotlin(args0)
})
}),
forwardProxyConvention = javaType.forwardProxyConvention(),
forwardProxyCustomHostHeaderName = javaType.forwardProxyCustomHostHeaderName(),
forwardProxyCustomSchemeHeaderName = javaType.forwardProxyCustomSchemeHeaderName(),
githubV2s = javaType.githubV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2GithubV2.Companion.toKotlin(args0)
})
}),
googleV2s = javaType.googleV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2GoogleV2.Companion.toKotlin(args0)
})
}),
httpRouteApiPrefix = javaType.httpRouteApiPrefix(),
logins = javaType.logins().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2Login.Companion.toKotlin(args0)
})
}),
microsoftV2s = javaType.microsoftV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2MicrosoftV2.Companion.toKotlin(args0)
})
}),
requireAuthentication = javaType.requireAuthentication(),
requireHttps = javaType.requireHttps(),
runtimeVersion = javaType.runtimeVersion(),
twitterV2s = javaType.twitterV2s().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.GetWindowsWebAppAuthSettingsV2TwitterV2.Companion.toKotlin(args0)
})
}),
unauthenticatedAction = javaType.unauthenticatedAction(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy