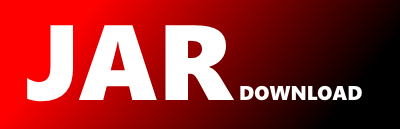
com.pulumi.azure.appservice.kotlin.outputs.LinuxWebAppSlotSiteConfig.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property alwaysOn If this Linux Web App is Always On enabled. Defaults to `true`.
* @property apiDefinitionUrl The URL to the API Definition for this Linux Web App Slot.
* @property apiManagementApiId The API Management API ID this Linux Web App Slot is associated with.
* @property appCommandLine The App command line to launch.
* @property applicationStack A `application_stack` block as defined above.
* @property autoHealEnabled Should Auto heal rules be enabled? Required with `auto_heal_setting`.
* @property autoHealSetting A `auto_heal_setting` block as defined above. Required with `auto_heal`.
* @property autoSwapSlotName The Linux Web App Slot Name to automatically swap to when deployment to that slot is successfully completed.
* > **Note:** This must be a valid slot name on the target Linux Web App.
* @property containerRegistryManagedIdentityClientId The Client ID of the Managed Service Identity to use for connections to the Azure Container Registry.
* @property containerRegistryUseManagedIdentity Should connections for Azure Container Registry use Managed Identity.
* @property cors A `cors` block as defined above.
* @property defaultDocuments Specifies a list of Default Documents for the Linux Web App.
* @property detailedErrorLoggingEnabled
* @property ftpsState
* @property healthCheckEvictionTimeInMin The amount of time in minutes that a node can be unhealthy before being removed from the load balancer. Possible values are between `2` and `10`. Only valid in conjunction with `health_check_path`.
* @property healthCheckPath The path to the Health Check.
* @property http2Enabled Should the HTTP2 be enabled?
* @property ipRestrictionDefaultAction The Default action for traffic that does not match any `ip_restriction` rule. possible values include `Allow` and `Deny`. Defaults to `Allow`.
* @property ipRestrictions One or more `ip_restriction` blocks as defined above.
* @property linuxFxVersion
* @property loadBalancingMode The Site load balancing. Possible values include: `WeightedRoundRobin`, `LeastRequests`, `LeastResponseTime`, `WeightedTotalTraffic`, `RequestHash`, `PerSiteRoundRobin`. Defaults to `LeastRequests` if omitted.
* @property localMysqlEnabled Use Local MySQL. Defaults to `false`.
* @property managedPipelineMode Managed pipeline mode. Possible values include: `Integrated`, `Classic`. Defaults to `Integrated`.
* @property minimumTlsVersion The configures the minimum version of TLS required for SSL requests. Possible values include: `1.0`, `1.1`, and `1.2`. Defaults to `1.2`.
* @property remoteDebuggingEnabled Should Remote Debugging be enabled? Defaults to `false`.
* @property remoteDebuggingVersion The Remote Debugging Version. Possible values include `VS2017` and `VS2019`
* @property scmIpRestrictionDefaultAction The Default action for traffic that does not match any `scm_ip_restriction` rule. possible values include `Allow` and `Deny`. Defaults to `Allow`.
* @property scmIpRestrictions One or more `scm_ip_restriction` blocks as defined above.
* @property scmMinimumTlsVersion The configures the minimum version of TLS required for SSL requests to the SCM site Possible values include: `1.0`, `1.1`, and `1.2`. Defaults to `1.2`.
* @property scmType
* @property scmUseMainIpRestriction Should the Linux Web App `ip_restriction` configuration be used for the SCM also.
* @property use32BitWorker Should the Linux Web App use a 32-bit worker? Defaults to `true`.
* @property vnetRouteAllEnabled Should all outbound traffic have NAT Gateways, Network Security Groups and User Defined Routes applied? Defaults to `false`.
* @property websocketsEnabled Should Web Sockets be enabled? Defaults to `false`.
* @property workerCount The number of Workers for this Linux App Service Slot.
*/
public data class LinuxWebAppSlotSiteConfig(
public val alwaysOn: Boolean? = null,
public val apiDefinitionUrl: String? = null,
public val apiManagementApiId: String? = null,
public val appCommandLine: String? = null,
public val applicationStack: LinuxWebAppSlotSiteConfigApplicationStack? = null,
public val autoHealEnabled: Boolean? = null,
public val autoHealSetting: LinuxWebAppSlotSiteConfigAutoHealSetting? = null,
public val autoSwapSlotName: String? = null,
public val containerRegistryManagedIdentityClientId: String? = null,
public val containerRegistryUseManagedIdentity: Boolean? = null,
public val cors: LinuxWebAppSlotSiteConfigCors? = null,
public val defaultDocuments: List? = null,
public val detailedErrorLoggingEnabled: Boolean? = null,
public val ftpsState: String? = null,
public val healthCheckEvictionTimeInMin: Int? = null,
public val healthCheckPath: String? = null,
public val http2Enabled: Boolean? = null,
public val ipRestrictionDefaultAction: String? = null,
public val ipRestrictions: List? = null,
public val linuxFxVersion: String? = null,
public val loadBalancingMode: String? = null,
public val localMysqlEnabled: Boolean? = null,
public val managedPipelineMode: String? = null,
public val minimumTlsVersion: String? = null,
public val remoteDebuggingEnabled: Boolean? = null,
public val remoteDebuggingVersion: String? = null,
public val scmIpRestrictionDefaultAction: String? = null,
public val scmIpRestrictions: List? = null,
public val scmMinimumTlsVersion: String? = null,
public val scmType: String? = null,
public val scmUseMainIpRestriction: Boolean? = null,
public val use32BitWorker: Boolean? = null,
public val vnetRouteAllEnabled: Boolean? = null,
public val websocketsEnabled: Boolean? = null,
public val workerCount: Int? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.LinuxWebAppSlotSiteConfig):
LinuxWebAppSlotSiteConfig = LinuxWebAppSlotSiteConfig(
alwaysOn = javaType.alwaysOn().map({ args0 -> args0 }).orElse(null),
apiDefinitionUrl = javaType.apiDefinitionUrl().map({ args0 -> args0 }).orElse(null),
apiManagementApiId = javaType.apiManagementApiId().map({ args0 -> args0 }).orElse(null),
appCommandLine = javaType.appCommandLine().map({ args0 -> args0 }).orElse(null),
applicationStack = javaType.applicationStack().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.LinuxWebAppSlotSiteConfigApplicationStack.Companion.toKotlin(args0)
})
}).orElse(null),
autoHealEnabled = javaType.autoHealEnabled().map({ args0 -> args0 }).orElse(null),
autoHealSetting = javaType.autoHealSetting().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.LinuxWebAppSlotSiteConfigAutoHealSetting.Companion.toKotlin(args0)
})
}).orElse(null),
autoSwapSlotName = javaType.autoSwapSlotName().map({ args0 -> args0 }).orElse(null),
containerRegistryManagedIdentityClientId = javaType.containerRegistryManagedIdentityClientId().map({ args0 ->
args0
}).orElse(null),
containerRegistryUseManagedIdentity = javaType.containerRegistryUseManagedIdentity().map({ args0 ->
args0
}).orElse(null),
cors = javaType.cors().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.LinuxWebAppSlotSiteConfigCors.Companion.toKotlin(args0)
})
}).orElse(null),
defaultDocuments = javaType.defaultDocuments().map({ args0 -> args0 }),
detailedErrorLoggingEnabled = javaType.detailedErrorLoggingEnabled().map({ args0 ->
args0
}).orElse(null),
ftpsState = javaType.ftpsState().map({ args0 -> args0 }).orElse(null),
healthCheckEvictionTimeInMin = javaType.healthCheckEvictionTimeInMin().map({ args0 ->
args0
}).orElse(null),
healthCheckPath = javaType.healthCheckPath().map({ args0 -> args0 }).orElse(null),
http2Enabled = javaType.http2Enabled().map({ args0 -> args0 }).orElse(null),
ipRestrictionDefaultAction = javaType.ipRestrictionDefaultAction().map({ args0 ->
args0
}).orElse(null),
ipRestrictions = javaType.ipRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.LinuxWebAppSlotSiteConfigIpRestriction.Companion.toKotlin(args0)
})
}),
linuxFxVersion = javaType.linuxFxVersion().map({ args0 -> args0 }).orElse(null),
loadBalancingMode = javaType.loadBalancingMode().map({ args0 -> args0 }).orElse(null),
localMysqlEnabled = javaType.localMysqlEnabled().map({ args0 -> args0 }).orElse(null),
managedPipelineMode = javaType.managedPipelineMode().map({ args0 -> args0 }).orElse(null),
minimumTlsVersion = javaType.minimumTlsVersion().map({ args0 -> args0 }).orElse(null),
remoteDebuggingEnabled = javaType.remoteDebuggingEnabled().map({ args0 -> args0 }).orElse(null),
remoteDebuggingVersion = javaType.remoteDebuggingVersion().map({ args0 -> args0 }).orElse(null),
scmIpRestrictionDefaultAction = javaType.scmIpRestrictionDefaultAction().map({ args0 ->
args0
}).orElse(null),
scmIpRestrictions = javaType.scmIpRestrictions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.appservice.kotlin.outputs.LinuxWebAppSlotSiteConfigScmIpRestriction.Companion.toKotlin(args0)
})
}),
scmMinimumTlsVersion = javaType.scmMinimumTlsVersion().map({ args0 -> args0 }).orElse(null),
scmType = javaType.scmType().map({ args0 -> args0 }).orElse(null),
scmUseMainIpRestriction = javaType.scmUseMainIpRestriction().map({ args0 -> args0 }).orElse(null),
use32BitWorker = javaType.use32BitWorker().map({ args0 -> args0 }).orElse(null),
vnetRouteAllEnabled = javaType.vnetRouteAllEnabled().map({ args0 -> args0 }).orElse(null),
websocketsEnabled = javaType.websocketsEnabled().map({ args0 -> args0 }).orElse(null),
workerCount = javaType.workerCount().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy