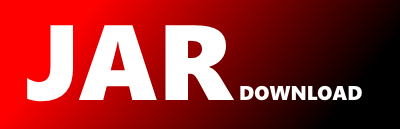
com.pulumi.azure.appservice.kotlin.outputs.WindowsWebAppSiteConfigApplicationStack.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.appservice.kotlin.outputs
import kotlin.Boolean
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
/**
*
* @property currentStack The Application Stack for the Windows Web App. Possible values include `dotnet`, `dotnetcore`, `node`, `python`, `php`, and `java`.
* > **NOTE:** Whilst this property is Optional omitting it can cause unexpected behaviour, in particular for display of settings in the Azure Portal.
* > **NOTE:** Windows Web apps can configure multiple `app_stack` properties, it is recommended to always configure this `Optional` value and set it to the primary application stack of your app to ensure correct operation of this resource and display the correct metadata in the Azure Portal.
* @property dockerContainerName The name of the container to be used. This value is required with `docker_container_tag`.
* @property dockerContainerRegistry
* @property dockerContainerTag The tag of the container to be used. This value is required with `docker_container_name`.
* @property dockerImageName The docker image, including tag, to be used. e.g. `azure-app-service/windows/parkingpage:latest`.
* @property dockerRegistryPassword The User Name to use for authentication against the registry to pull the image.
* > **NOTE:** `docker_registry_url`, `docker_registry_username`, and `docker_registry_password` replace the use of the `app_settings` values of `DOCKER_REGISTRY_SERVER_URL`, `DOCKER_REGISTRY_SERVER_USERNAME` and `DOCKER_REGISTRY_SERVER_PASSWORD` respectively, these values will be managed by the provider and should not be specified in the `app_settings` map.
* @property dockerRegistryUrl The URL of the container registry where the `docker_image_name` is located. e.g. `https://index.docker.io` or `https://mcr.microsoft.com`. This value is required with `docker_image_name`.
* @property dockerRegistryUsername The User Name to use for authentication against the registry to pull the image.
* @property dotnetCoreVersion The version of .NET to use when `current_stack` is set to `dotnetcore`. Possible values include `v4.0`.
* @property dotnetVersion The version of .NET to use when `current_stack` is set to `dotnet`. Possible values include `v2.0`,`v3.0`, `v4.0`, `v5.0`, `v6.0`, `v7.0` and `v8.0`.
* > **NOTE:** The Portal displayed values and the actual underlying API values differ for this setting, as follows:
* Portal Value | API value
* :--|--:
* ASP.NET V3.5 | v2.0
* ASP.NET V4.8 | v4.0
* .NET 6 (LTS) | v6.0
* .NET 7 (STS) | v7.0
* .NET 8 (LTS) | v8.0
* @property javaContainer
* @property javaContainerVersion
* @property javaEmbeddedServerEnabled Should the Java Embedded Server (Java SE) be used to run the app.
* @property javaVersion The version of Java to use when `current_stack` is set to `java`.
* > **NOTE:** For currently supported versions, please see the official documentation. Some example values include: `1.8`, `1.8.0_322`, `11`, `11.0.14`, `17` and `17.0.2`
* @property nodeVersion The version of node to use when `current_stack` is set to `node`. Possible values are `~12`, `~14`, `~16`, `~18` and `~20`.
* > **NOTE:** This property conflicts with `java_version`.
* @property phpVersion The version of PHP to use when `current_stack` is set to `php`. Possible values are `7.1`, `7.4` and `Off`.
* > **NOTE:** The value `Off` is used to signify latest supported by the service.
* @property python Specifies whether this is a Python app. Defaults to `false`.
* @property pythonVersion
* @property tomcatVersion The version of Tomcat the Java App should use. Conflicts with `java_embedded_server_enabled`
* > **NOTE:** See the official documentation for current supported versions. Some example valuess include: `10.0`, `10.0.20`.
*/
public data class WindowsWebAppSiteConfigApplicationStack(
public val currentStack: String? = null,
public val dockerContainerName: String? = null,
@Deprecated(
message = """
This property has been deprecated and will be removed in a future release of the provider.
""",
)
public val dockerContainerRegistry: String? = null,
public val dockerContainerTag: String? = null,
public val dockerImageName: String? = null,
public val dockerRegistryPassword: String? = null,
public val dockerRegistryUrl: String? = null,
public val dockerRegistryUsername: String? = null,
public val dotnetCoreVersion: String? = null,
public val dotnetVersion: String? = null,
@Deprecated(
message = """
this property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
public val javaContainer: String? = null,
@Deprecated(
message = """
This property has been deprecated in favour of `tomcat_version` and `java_embedded_server_enabled`
""",
)
public val javaContainerVersion: String? = null,
public val javaEmbeddedServerEnabled: Boolean? = null,
public val javaVersion: String? = null,
public val nodeVersion: String? = null,
public val phpVersion: String? = null,
public val python: Boolean? = null,
@Deprecated(
message = """
This property is deprecated. Values set are not used by the service.
""",
)
public val pythonVersion: String? = null,
public val tomcatVersion: String? = null,
) {
public companion object {
public
fun toKotlin(javaType: com.pulumi.azure.appservice.outputs.WindowsWebAppSiteConfigApplicationStack):
WindowsWebAppSiteConfigApplicationStack = WindowsWebAppSiteConfigApplicationStack(
currentStack = javaType.currentStack().map({ args0 -> args0 }).orElse(null),
dockerContainerName = javaType.dockerContainerName().map({ args0 -> args0 }).orElse(null),
dockerContainerRegistry = javaType.dockerContainerRegistry().map({ args0 -> args0 }).orElse(null),
dockerContainerTag = javaType.dockerContainerTag().map({ args0 -> args0 }).orElse(null),
dockerImageName = javaType.dockerImageName().map({ args0 -> args0 }).orElse(null),
dockerRegistryPassword = javaType.dockerRegistryPassword().map({ args0 -> args0 }).orElse(null),
dockerRegistryUrl = javaType.dockerRegistryUrl().map({ args0 -> args0 }).orElse(null),
dockerRegistryUsername = javaType.dockerRegistryUsername().map({ args0 -> args0 }).orElse(null),
dotnetCoreVersion = javaType.dotnetCoreVersion().map({ args0 -> args0 }).orElse(null),
dotnetVersion = javaType.dotnetVersion().map({ args0 -> args0 }).orElse(null),
javaContainer = javaType.javaContainer().map({ args0 -> args0 }).orElse(null),
javaContainerVersion = javaType.javaContainerVersion().map({ args0 -> args0 }).orElse(null),
javaEmbeddedServerEnabled = javaType.javaEmbeddedServerEnabled().map({ args0 ->
args0
}).orElse(null),
javaVersion = javaType.javaVersion().map({ args0 -> args0 }).orElse(null),
nodeVersion = javaType.nodeVersion().map({ args0 -> args0 }).orElse(null),
phpVersion = javaType.phpVersion().map({ args0 -> args0 }).orElse(null),
python = javaType.python().map({ args0 -> args0 }).orElse(null),
pythonVersion = javaType.pythonVersion().map({ args0 -> args0 }).orElse(null),
tomcatVersion = javaType.tomcatVersion().map({ args0 -> args0 }).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy