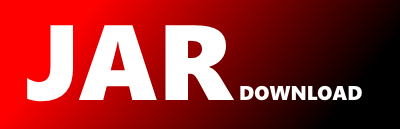
com.pulumi.azure.arckubernetes.kotlin.inputs.FluxConfigurationBucketArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.arckubernetes.kotlin.inputs
import com.pulumi.azure.arckubernetes.inputs.FluxConfigurationBucketArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property accessKey Specifies the plaintext access key used to securely access the S3 bucket.
* @property bucketName Specifies the bucket name to sync from the url endpoint for the flux configuration.
* @property localAuthReference Specifies the name of a local secret on the Kubernetes cluster to use as the authentication secret rather than the managed or user-provided configuration secrets.
* @property secretKeyBase64 Specifies the Base64-encoded secret key used to authenticate with the bucket source.
* @property syncIntervalInSeconds Specifies the interval at which to re-reconcile the cluster git repository source with the remote. Defaults to `600`.
* @property timeoutInSeconds Specifies the maximum time to attempt to reconcile the cluster git repository source with the remote. Defaults to `600`.
* @property tlsEnabled Specify whether to communicate with a bucket using TLS is enabled. Defaults to `true`.
* @property url Specifies the URL to sync for the flux configuration S3 bucket. It must start with `http://` or `https://`.
*/
public data class FluxConfigurationBucketArgs(
public val accessKey: Output? = null,
public val bucketName: Output,
public val localAuthReference: Output? = null,
public val secretKeyBase64: Output? = null,
public val syncIntervalInSeconds: Output? = null,
public val timeoutInSeconds: Output? = null,
public val tlsEnabled: Output? = null,
public val url: Output,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.arckubernetes.inputs.FluxConfigurationBucketArgs =
com.pulumi.azure.arckubernetes.inputs.FluxConfigurationBucketArgs.builder()
.accessKey(accessKey?.applyValue({ args0 -> args0 }))
.bucketName(bucketName.applyValue({ args0 -> args0 }))
.localAuthReference(localAuthReference?.applyValue({ args0 -> args0 }))
.secretKeyBase64(secretKeyBase64?.applyValue({ args0 -> args0 }))
.syncIntervalInSeconds(syncIntervalInSeconds?.applyValue({ args0 -> args0 }))
.timeoutInSeconds(timeoutInSeconds?.applyValue({ args0 -> args0 }))
.tlsEnabled(tlsEnabled?.applyValue({ args0 -> args0 }))
.url(url.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FluxConfigurationBucketArgs].
*/
@PulumiTagMarker
public class FluxConfigurationBucketArgsBuilder internal constructor() {
private var accessKey: Output? = null
private var bucketName: Output? = null
private var localAuthReference: Output? = null
private var secretKeyBase64: Output? = null
private var syncIntervalInSeconds: Output? = null
private var timeoutInSeconds: Output? = null
private var tlsEnabled: Output? = null
private var url: Output? = null
/**
* @param value Specifies the plaintext access key used to securely access the S3 bucket.
*/
@JvmName("ungasaecghndwgqa")
public suspend fun accessKey(`value`: Output) {
this.accessKey = value
}
/**
* @param value Specifies the bucket name to sync from the url endpoint for the flux configuration.
*/
@JvmName("bdcytcdnbwddoeot")
public suspend fun bucketName(`value`: Output) {
this.bucketName = value
}
/**
* @param value Specifies the name of a local secret on the Kubernetes cluster to use as the authentication secret rather than the managed or user-provided configuration secrets.
*/
@JvmName("pmirbvjpbksmpscm")
public suspend fun localAuthReference(`value`: Output) {
this.localAuthReference = value
}
/**
* @param value Specifies the Base64-encoded secret key used to authenticate with the bucket source.
*/
@JvmName("yadjpmuyxxydkhah")
public suspend fun secretKeyBase64(`value`: Output) {
this.secretKeyBase64 = value
}
/**
* @param value Specifies the interval at which to re-reconcile the cluster git repository source with the remote. Defaults to `600`.
*/
@JvmName("vjftqtcrbymkyayo")
public suspend fun syncIntervalInSeconds(`value`: Output) {
this.syncIntervalInSeconds = value
}
/**
* @param value Specifies the maximum time to attempt to reconcile the cluster git repository source with the remote. Defaults to `600`.
*/
@JvmName("flssxnmsasrsctej")
public suspend fun timeoutInSeconds(`value`: Output) {
this.timeoutInSeconds = value
}
/**
* @param value Specify whether to communicate with a bucket using TLS is enabled. Defaults to `true`.
*/
@JvmName("plffxlvimsoloxsa")
public suspend fun tlsEnabled(`value`: Output) {
this.tlsEnabled = value
}
/**
* @param value Specifies the URL to sync for the flux configuration S3 bucket. It must start with `http://` or `https://`.
*/
@JvmName("ragohxoelagevxhx")
public suspend fun url(`value`: Output) {
this.url = value
}
/**
* @param value Specifies the plaintext access key used to securely access the S3 bucket.
*/
@JvmName("mhivjplifgyxioat")
public suspend fun accessKey(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.accessKey = mapped
}
/**
* @param value Specifies the bucket name to sync from the url endpoint for the flux configuration.
*/
@JvmName("tfqfrkyyoniogbsk")
public suspend fun bucketName(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.bucketName = mapped
}
/**
* @param value Specifies the name of a local secret on the Kubernetes cluster to use as the authentication secret rather than the managed or user-provided configuration secrets.
*/
@JvmName("ahyysqvxwhefjchv")
public suspend fun localAuthReference(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.localAuthReference = mapped
}
/**
* @param value Specifies the Base64-encoded secret key used to authenticate with the bucket source.
*/
@JvmName("intqdvhjitbusaph")
public suspend fun secretKeyBase64(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secretKeyBase64 = mapped
}
/**
* @param value Specifies the interval at which to re-reconcile the cluster git repository source with the remote. Defaults to `600`.
*/
@JvmName("fhjfnlynodfvikht")
public suspend fun syncIntervalInSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.syncIntervalInSeconds = mapped
}
/**
* @param value Specifies the maximum time to attempt to reconcile the cluster git repository source with the remote. Defaults to `600`.
*/
@JvmName("amfleryaplyfkmit")
public suspend fun timeoutInSeconds(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.timeoutInSeconds = mapped
}
/**
* @param value Specify whether to communicate with a bucket using TLS is enabled. Defaults to `true`.
*/
@JvmName("gvlfplxhvfrtrext")
public suspend fun tlsEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.tlsEnabled = mapped
}
/**
* @param value Specifies the URL to sync for the flux configuration S3 bucket. It must start with `http://` or `https://`.
*/
@JvmName("gpgmjavrwyklfhcp")
public suspend fun url(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.url = mapped
}
internal fun build(): FluxConfigurationBucketArgs = FluxConfigurationBucketArgs(
accessKey = accessKey,
bucketName = bucketName ?: throw PulumiNullFieldException("bucketName"),
localAuthReference = localAuthReference,
secretKeyBase64 = secretKeyBase64,
syncIntervalInSeconds = syncIntervalInSeconds,
timeoutInSeconds = timeoutInSeconds,
tlsEnabled = tlsEnabled,
url = url ?: throw PulumiNullFieldException("url"),
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy