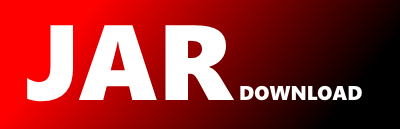
com.pulumi.azure.arcmachine.kotlin.outputs.GetResult.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.arcmachine.kotlin.outputs
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.collections.Map
/**
* A collection of values returned by get.
* @property activeDirectoryFqdn Specifies the AD fully qualified display name.
* @property agentVersion The Azure Arc machine agent full version.
* @property agents A `agent` block as defined below.
* @property clientPublicKey Public Key that the client provides to be used during initial resource onboarding.
* @property cloudMetadatas A `cloud_metadata` block as defined below.
* @property detectedProperties A `detected_properties` block as defined below.
* @property displayName Specifies the Azure Arc machine display name.
* @property dnsFqdn Specifies the DNS fully qualified display name.
* @property domainName Specifies the Windows domain name.
* @property id The provider-assigned unique ID for this managed resource.
* @property identities A `identity` block as defined below.
* @property lastStatusChangeTime The time of the last status change.
* @property location The Azure Region where the Azure Arc machine exists.
* @property locationDatas A `location_data` block as defined below.
* @property machineFqdn Specifies the Azure Arc machine fully qualified display name.
* @property mssqlDiscovered Specifies whether any MS SQL instance is discovered on the machine.
* @property name A canonical name for the geographic or physical location.
* @property osName The Operating System running on the Azure Arc machine.
* @property osProfiles A `os_profile` block as defined below.
* @property osSku Specifies the Operating System product SKU.
* @property osType The type of Operating System. Possible values are `windows` and `linux`.
* @property osVersion The version of Operating System running on the Azure Arc machine.
* @property parentClusterResourceId The resource id of the parent cluster (Azure HCI) this machine is assigned to, if any.
* @property privateLinkScopeResourceId The resource id of the parent cluster (Azure HCI) this machine is assigned to, if any.
* @property resourceGroupName
* @property serviceStatuses A `service_status` block as defined below.
* @property status The current status of the service.
* @property tags A mapping of tags assigned to the Hybrid Compute.
* @property vmId Specifies the Azure Arc machine unique ID.
* @property vmUuid Specifies the Arc Machine's unique SMBIOS ID.
*/
public data class GetResult(
public val activeDirectoryFqdn: String,
public val agentVersion: String,
public val agents: List,
public val clientPublicKey: String,
public val cloudMetadatas: List,
public val detectedProperties: Map,
public val displayName: String,
public val dnsFqdn: String,
public val domainName: String,
public val id: String,
public val identities: List,
public val lastStatusChangeTime: String,
public val location: String,
public val locationDatas: List,
public val machineFqdn: String,
public val mssqlDiscovered: Boolean,
public val name: String,
public val osName: String,
public val osProfiles: List,
public val osSku: String,
public val osType: String,
public val osVersion: String,
public val parentClusterResourceId: String,
public val privateLinkScopeResourceId: String,
public val resourceGroupName: String,
public val serviceStatuses: List,
public val status: String,
public val tags: Map,
public val vmId: String,
public val vmUuid: String,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.arcmachine.outputs.GetResult): GetResult =
GetResult(
activeDirectoryFqdn = javaType.activeDirectoryFqdn(),
agentVersion = javaType.agentVersion(),
agents = javaType.agents().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.arcmachine.kotlin.outputs.GetAgent.Companion.toKotlin(args0)
})
}),
clientPublicKey = javaType.clientPublicKey(),
cloudMetadatas = javaType.cloudMetadatas().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.arcmachine.kotlin.outputs.GetCloudMetadata.Companion.toKotlin(args0)
})
}),
detectedProperties = javaType.detectedProperties().map({ args0 ->
args0.key.to(args0.value)
}).toMap(),
displayName = javaType.displayName(),
dnsFqdn = javaType.dnsFqdn(),
domainName = javaType.domainName(),
id = javaType.id(),
identities = javaType.identities().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.arcmachine.kotlin.outputs.GetIdentity.Companion.toKotlin(args0)
})
}),
lastStatusChangeTime = javaType.lastStatusChangeTime(),
location = javaType.location(),
locationDatas = javaType.locationDatas().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.arcmachine.kotlin.outputs.GetLocationData.Companion.toKotlin(args0)
})
}),
machineFqdn = javaType.machineFqdn(),
mssqlDiscovered = javaType.mssqlDiscovered(),
name = javaType.name(),
osName = javaType.osName(),
osProfiles = javaType.osProfiles().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.arcmachine.kotlin.outputs.GetOsProfile.Companion.toKotlin(args0)
})
}),
osSku = javaType.osSku(),
osType = javaType.osType(),
osVersion = javaType.osVersion(),
parentClusterResourceId = javaType.parentClusterResourceId(),
privateLinkScopeResourceId = javaType.privateLinkScopeResourceId(),
resourceGroupName = javaType.resourceGroupName(),
serviceStatuses = javaType.serviceStatuses().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.arcmachine.kotlin.outputs.GetServiceStatus.Companion.toKotlin(args0)
})
}),
status = javaType.status(),
tags = javaType.tags().map({ args0 -> args0.key.to(args0.value) }).toMap(),
vmId = javaType.vmId(),
vmUuid = javaType.vmUuid(),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy