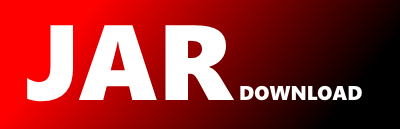
com.pulumi.azure.automation.kotlin.SoftwareUpdateConfigurationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.automation.kotlin
import com.pulumi.azure.automation.SoftwareUpdateConfigurationArgs.builder
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationLinuxArgs
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationLinuxArgsBuilder
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationPostTaskArgs
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationPostTaskArgsBuilder
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationPreTaskArgs
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationPreTaskArgsBuilder
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationScheduleArgs
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationScheduleArgsBuilder
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationTargetArgs
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationTargetArgsBuilder
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationWindowsArgs
import com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationWindowsArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Deprecated
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages an Automation Software Update Configuraion.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-rg",
* location: "East US",
* });
* const exampleAccount = new azure.automation.Account("example", {
* name: "example",
* location: example.location,
* resourceGroupName: example.name,
* skuName: "Basic",
* });
* const exampleRunBook = new azure.automation.RunBook("example", {
* name: "Get-AzureVMTutorial",
* location: example.location,
* resourceGroupName: example.name,
* automationAccountName: exampleAccount.name,
* logVerbose: true,
* logProgress: true,
* description: "This is a example runbook for terraform acceptance example",
* runbookType: "Python3",
* content: `# Some example content
* # for Terraform acceptance example
* `,
* tags: {
* ENV: "runbook_test",
* },
* });
* const exampleSoftwareUpdateConfiguration = new azure.automation.SoftwareUpdateConfiguration("example", {
* name: "example",
* automationAccountId: exampleAccount.id,
* operatingSystem: "Linux",
* linuxes: [{
* classificationIncluded: "Security",
* excludedPackages: ["apt"],
* includedPackages: ["vim"],
* reboot: "IfRequired",
* }],
* preTasks: [{
* source: exampleRunBook.name,
* parameters: {
* COMPUTER_NAME: "Foo",
* },
* }],
* duration: "PT2H2M2S",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-rg",
* location="East US")
* example_account = azure.automation.Account("example",
* name="example",
* location=example.location,
* resource_group_name=example.name,
* sku_name="Basic")
* example_run_book = azure.automation.RunBook("example",
* name="Get-AzureVMTutorial",
* location=example.location,
* resource_group_name=example.name,
* automation_account_name=example_account.name,
* log_verbose=True,
* log_progress=True,
* description="This is a example runbook for terraform acceptance example",
* runbook_type="Python3",
* content="""# Some example content
* # for Terraform acceptance example
* """,
* tags={
* "ENV": "runbook_test",
* })
* example_software_update_configuration = azure.automation.SoftwareUpdateConfiguration("example",
* name="example",
* automation_account_id=example_account.id,
* operating_system="Linux",
* linuxes=[azure.automation.SoftwareUpdateConfigurationLinuxArgs(
* classification_included="Security",
* excluded_packages=["apt"],
* included_packages=["vim"],
* reboot="IfRequired",
* )],
* pre_tasks=[azure.automation.SoftwareUpdateConfigurationPreTaskArgs(
* source=example_run_book.name,
* parameters={
* "COMPUTER_NAME": "Foo",
* },
* )],
* duration="PT2H2M2S")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-rg",
* Location = "East US",
* });
* var exampleAccount = new Azure.Automation.Account("example", new()
* {
* Name = "example",
* Location = example.Location,
* ResourceGroupName = example.Name,
* SkuName = "Basic",
* });
* var exampleRunBook = new Azure.Automation.RunBook("example", new()
* {
* Name = "Get-AzureVMTutorial",
* Location = example.Location,
* ResourceGroupName = example.Name,
* AutomationAccountName = exampleAccount.Name,
* LogVerbose = true,
* LogProgress = true,
* Description = "This is a example runbook for terraform acceptance example",
* RunbookType = "Python3",
* Content = @"# Some example content
* # for Terraform acceptance example
* ",
* Tags =
* {
* { "ENV", "runbook_test" },
* },
* });
* var exampleSoftwareUpdateConfiguration = new Azure.Automation.SoftwareUpdateConfiguration("example", new()
* {
* Name = "example",
* AutomationAccountId = exampleAccount.Id,
* OperatingSystem = "Linux",
* Linuxes = new[]
* {
* new Azure.Automation.Inputs.SoftwareUpdateConfigurationLinuxArgs
* {
* ClassificationIncluded = "Security",
* ExcludedPackages = new[]
* {
* "apt",
* },
* IncludedPackages = new[]
* {
* "vim",
* },
* Reboot = "IfRequired",
* },
* },
* PreTasks = new[]
* {
* new Azure.Automation.Inputs.SoftwareUpdateConfigurationPreTaskArgs
* {
* Source = exampleRunBook.Name,
* Parameters =
* {
* { "COMPUTER_NAME", "Foo" },
* },
* },
* },
* Duration = "PT2H2M2S",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/automation"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-rg"),
* Location: pulumi.String("East US"),
* })
* if err != nil {
* return err
* }
* exampleAccount, err := automation.NewAccount(ctx, "example", &automation.AccountArgs{
* Name: pulumi.String("example"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* SkuName: pulumi.String("Basic"),
* })
* if err != nil {
* return err
* }
* exampleRunBook, err := automation.NewRunBook(ctx, "example", &automation.RunBookArgs{
* Name: pulumi.String("Get-AzureVMTutorial"),
* Location: example.Location,
* ResourceGroupName: example.Name,
* AutomationAccountName: exampleAccount.Name,
* LogVerbose: pulumi.Bool(true),
* LogProgress: pulumi.Bool(true),
* Description: pulumi.String("This is a example runbook for terraform acceptance example"),
* RunbookType: pulumi.String("Python3"),
* Content: pulumi.String("# Some example content\n# for Terraform acceptance example\n"),
* Tags: pulumi.StringMap{
* "ENV": pulumi.String("runbook_test"),
* },
* })
* if err != nil {
* return err
* }
* _, err = automation.NewSoftwareUpdateConfiguration(ctx, "example", &automation.SoftwareUpdateConfigurationArgs{
* Name: pulumi.String("example"),
* AutomationAccountId: exampleAccount.ID(),
* OperatingSystem: pulumi.String("Linux"),
* Linuxes: automation.SoftwareUpdateConfigurationLinuxArray{
* &automation.SoftwareUpdateConfigurationLinuxArgs{
* ClassificationIncluded: pulumi.String("Security"),
* ExcludedPackages: pulumi.StringArray{
* pulumi.String("apt"),
* },
* IncludedPackages: pulumi.StringArray{
* pulumi.String("vim"),
* },
* Reboot: pulumi.String("IfRequired"),
* },
* },
* PreTasks: automation.SoftwareUpdateConfigurationPreTaskArray{
* &automation.SoftwareUpdateConfigurationPreTaskArgs{
* Source: exampleRunBook.Name,
* Parameters: pulumi.StringMap{
* "COMPUTER_NAME": pulumi.String("Foo"),
* },
* },
* },
* Duration: pulumi.String("PT2H2M2S"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.automation.Account;
* import com.pulumi.azure.automation.AccountArgs;
* import com.pulumi.azure.automation.RunBook;
* import com.pulumi.azure.automation.RunBookArgs;
* import com.pulumi.azure.automation.SoftwareUpdateConfiguration;
* import com.pulumi.azure.automation.SoftwareUpdateConfigurationArgs;
* import com.pulumi.azure.automation.inputs.SoftwareUpdateConfigurationLinuxArgs;
* import com.pulumi.azure.automation.inputs.SoftwareUpdateConfigurationPreTaskArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-rg")
* .location("East US")
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("example")
* .location(example.location())
* .resourceGroupName(example.name())
* .skuName("Basic")
* .build());
* var exampleRunBook = new RunBook("exampleRunBook", RunBookArgs.builder()
* .name("Get-AzureVMTutorial")
* .location(example.location())
* .resourceGroupName(example.name())
* .automationAccountName(exampleAccount.name())
* .logVerbose("true")
* .logProgress("true")
* .description("This is a example runbook for terraform acceptance example")
* .runbookType("Python3")
* .content("""
* # Some example content
* # for Terraform acceptance example
* """)
* .tags(Map.of("ENV", "runbook_test"))
* .build());
* var exampleSoftwareUpdateConfiguration = new SoftwareUpdateConfiguration("exampleSoftwareUpdateConfiguration", SoftwareUpdateConfigurationArgs.builder()
* .name("example")
* .automationAccountId(exampleAccount.id())
* .operatingSystem("Linux")
* .linuxes(SoftwareUpdateConfigurationLinuxArgs.builder()
* .classificationIncluded("Security")
* .excludedPackages("apt")
* .includedPackages("vim")
* .reboot("IfRequired")
* .build())
* .preTasks(SoftwareUpdateConfigurationPreTaskArgs.builder()
* .source(exampleRunBook.name())
* .parameters(Map.of("COMPUTER_NAME", "Foo"))
* .build())
* .duration("PT2H2M2S")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-rg
* location: East US
* exampleAccount:
* type: azure:automation:Account
* name: example
* properties:
* name: example
* location: ${example.location}
* resourceGroupName: ${example.name}
* skuName: Basic
* exampleRunBook:
* type: azure:automation:RunBook
* name: example
* properties:
* name: Get-AzureVMTutorial
* location: ${example.location}
* resourceGroupName: ${example.name}
* automationAccountName: ${exampleAccount.name}
* logVerbose: 'true'
* logProgress: 'true'
* description: This is a example runbook for terraform acceptance example
* runbookType: Python3
* content: |
* # Some example content
* # for Terraform acceptance example
* tags:
* ENV: runbook_test
* exampleSoftwareUpdateConfiguration:
* type: azure:automation:SoftwareUpdateConfiguration
* name: example
* properties:
* name: example
* automationAccountId: ${exampleAccount.id}
* operatingSystem: Linux
* linuxes:
* - classificationIncluded: Security
* excludedPackages:
* - apt
* includedPackages:
* - vim
* reboot: IfRequired
* preTasks:
* - source: ${exampleRunBook.name}
* parameters:
* COMPUTER_NAME: Foo
* duration: PT2H2M2S
* ```
*
* ## Import
* Automations Software Update Configuration can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:automation/softwareUpdateConfiguration:SoftwareUpdateConfiguration example /subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/group1/providers/Microsoft.Automation/automationAccounts/account1/softwareUpdateConfigurations/suc1
* ```
* @property automationAccountId The ID of Automation Account to manage this Source Control. Changing this forces a new Automation Source Control to be created.
* @property duration Maximum time allowed for the software update configuration run. using format `PT[n]H[n]M[n]S` as per ISO8601. Defaults to `PT2H`.
* @property linuxes A `linux` block as defined below.
* @property name The name which should be used for this Automation. Changing this forces a new Automation to be created.
* @property nonAzureComputerNames Specifies a list of names of non-Azure machines for the software update configuration.
* @property operatingSystem
* @property postTasks A `post_task` blocks as defined below.
* @property preTasks A `pre_task` blocks as defined below.
* @property schedules A `schedule` blocks as defined below.
* @property target A `target` blocks as defined below.
* @property virtualMachineIds Specifies a list of Azure Resource IDs of azure virtual machines.
* @property windows A `windows` block as defined below.
* > **NOTE:** One of `linux` or `windows` must be specified.
*/
public data class SoftwareUpdateConfigurationArgs(
public val automationAccountId: Output? = null,
public val duration: Output? = null,
public val linuxes: Output>? = null,
public val name: Output? = null,
public val nonAzureComputerNames: Output>? = null,
@Deprecated(
message = """
This property has been deprecated and will be removed in a future release. The use of either the
`linux` or `windows` blocks replaces setting this value directly. This value is ignored by the
provider.
""",
)
public val operatingSystem: Output? = null,
public val postTasks: Output>? = null,
public val preTasks: Output>? = null,
public val schedules: Output>? = null,
public val target: Output? = null,
public val virtualMachineIds: Output>? = null,
public val windows: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.automation.SoftwareUpdateConfigurationArgs =
com.pulumi.azure.automation.SoftwareUpdateConfigurationArgs.builder()
.automationAccountId(automationAccountId?.applyValue({ args0 -> args0 }))
.duration(duration?.applyValue({ args0 -> args0 }))
.linuxes(
linuxes?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.name(name?.applyValue({ args0 -> args0 }))
.nonAzureComputerNames(nonAzureComputerNames?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.operatingSystem(operatingSystem?.applyValue({ args0 -> args0 }))
.postTasks(
postTasks?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.preTasks(
preTasks?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.schedules(
schedules?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.target(target?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.virtualMachineIds(virtualMachineIds?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.windows(windows?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) })).build()
}
/**
* Builder for [SoftwareUpdateConfigurationArgs].
*/
@PulumiTagMarker
public class SoftwareUpdateConfigurationArgsBuilder internal constructor() {
private var automationAccountId: Output? = null
private var duration: Output? = null
private var linuxes: Output>? = null
private var name: Output? = null
private var nonAzureComputerNames: Output>? = null
private var operatingSystem: Output? = null
private var postTasks: Output>? = null
private var preTasks: Output>? = null
private var schedules: Output>? = null
private var target: Output? = null
private var virtualMachineIds: Output>? = null
private var windows: Output? = null
/**
* @param value The ID of Automation Account to manage this Source Control. Changing this forces a new Automation Source Control to be created.
*/
@JvmName("ouiqdondimlyvuxm")
public suspend fun automationAccountId(`value`: Output) {
this.automationAccountId = value
}
/**
* @param value Maximum time allowed for the software update configuration run. using format `PT[n]H[n]M[n]S` as per ISO8601. Defaults to `PT2H`.
*/
@JvmName("wpnalfyljpgledob")
public suspend fun duration(`value`: Output) {
this.duration = value
}
/**
* @param value A `linux` block as defined below.
*/
@JvmName("ygjkrfhnghmgjmrw")
public suspend fun linuxes(`value`: Output>) {
this.linuxes = value
}
@JvmName("ciicidoyoctilbwd")
public suspend fun linuxes(vararg values: Output) {
this.linuxes = Output.all(values.asList())
}
/**
* @param values A `linux` block as defined below.
*/
@JvmName("cuoswvvhmvqoqslo")
public suspend fun linuxes(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy