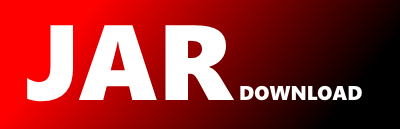
com.pulumi.azure.automation.kotlin.inputs.SoftwareUpdateConfigurationScheduleArgs.kt Maven / Gradle / Ivy
Go to download
The Apache Commons Codec package contains simple encoder and decoders for
various formats such as Base64 and Hexadecimal. In addition to these
widely used encoders and decoders, the codec package also maintains a
collection of phonetic encoding utilities.
The newest version!
Please wait ...