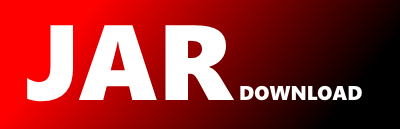
com.pulumi.azure.avs.kotlin.PrivateCloudArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.avs.kotlin
import com.pulumi.azure.avs.PrivateCloudArgs.builder
import com.pulumi.azure.avs.kotlin.inputs.PrivateCloudManagementClusterArgs
import com.pulumi.azure.avs.kotlin.inputs.PrivateCloudManagementClusterArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Pair
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.Map
import kotlin.jvm.JvmName
/**
* Manages an Azure VMware Solution Private Cloud.
* ## Example Usage
* > **NOTE :** Normal `pulumi up` could ignore this note. Please disable correlation request id for continuous operations in one build (like acctest). The continuous operations like `update` or `delete` could not be triggered when it shares the same `correlation-id` with its previous operation.
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const examplePrivateCloud = new azure.avs.PrivateCloud("example", {
* name: "example-vmware-private-cloud",
* resourceGroupName: example.name,
* location: example.location,
* skuName: "av36",
* managementCluster: {
* size: 3,
* },
* networkSubnetCidr: "192.168.48.0/22",
* internetConnectionEnabled: false,
* nsxtPassword: "QazWsx13$Edc",
* vcenterPassword: "WsxEdc23$Rfv",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_private_cloud = azure.avs.PrivateCloud("example",
* name="example-vmware-private-cloud",
* resource_group_name=example.name,
* location=example.location,
* sku_name="av36",
* management_cluster=azure.avs.PrivateCloudManagementClusterArgs(
* size=3,
* ),
* network_subnet_cidr="192.168.48.0/22",
* internet_connection_enabled=False,
* nsxt_password="QazWsx13$Edc",
* vcenter_password="WsxEdc23$Rfv")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var examplePrivateCloud = new Azure.Avs.PrivateCloud("example", new()
* {
* Name = "example-vmware-private-cloud",
* ResourceGroupName = example.Name,
* Location = example.Location,
* SkuName = "av36",
* ManagementCluster = new Azure.Avs.Inputs.PrivateCloudManagementClusterArgs
* {
* Size = 3,
* },
* NetworkSubnetCidr = "192.168.48.0/22",
* InternetConnectionEnabled = false,
* NsxtPassword = "QazWsx13$Edc",
* VcenterPassword = "WsxEdc23$Rfv",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/avs"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* _, err = avs.NewPrivateCloud(ctx, "example", &avs.PrivateCloudArgs{
* Name: pulumi.String("example-vmware-private-cloud"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* SkuName: pulumi.String("av36"),
* ManagementCluster: &avs.PrivateCloudManagementClusterArgs{
* Size: pulumi.Int(3),
* },
* NetworkSubnetCidr: pulumi.String("192.168.48.0/22"),
* InternetConnectionEnabled: pulumi.Bool(false),
* NsxtPassword: pulumi.String("QazWsx13$Edc"),
* VcenterPassword: pulumi.String("WsxEdc23$Rfv"),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.avs.PrivateCloud;
* import com.pulumi.azure.avs.PrivateCloudArgs;
* import com.pulumi.azure.avs.inputs.PrivateCloudManagementClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var examplePrivateCloud = new PrivateCloud("examplePrivateCloud", PrivateCloudArgs.builder()
* .name("example-vmware-private-cloud")
* .resourceGroupName(example.name())
* .location(example.location())
* .skuName("av36")
* .managementCluster(PrivateCloudManagementClusterArgs.builder()
* .size(3)
* .build())
* .networkSubnetCidr("192.168.48.0/22")
* .internetConnectionEnabled(false)
* .nsxtPassword("QazWsx13$Edc")
* .vcenterPassword("WsxEdc23$Rfv")
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* examplePrivateCloud:
* type: azure:avs:PrivateCloud
* name: example
* properties:
* name: example-vmware-private-cloud
* resourceGroupName: ${example.name}
* location: ${example.location}
* skuName: av36
* managementCluster:
* size: 3
* networkSubnetCidr: 192.168.48.0/22
* internetConnectionEnabled: false
* nsxtPassword: QazWsx13$Edc
* vcenterPassword: WsxEdc23$Rfv
* ```
*
* ## Import
* Azure VMware Solution Private Clouds can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:avs/privateCloud:PrivateCloud example /subscriptions/00000000-0000-0000-0000-000000000000/resourceGroups/group1/providers/Microsoft.AVS/privateClouds/privateCloud1
* ```
* @property internetConnectionEnabled Is the Azure VMware Solution Private Cloud connected to the internet? This field can not be updated with `management_cluster[0].size` together.
* > **NOTE :** `internet_connection_enabled` and `management_cluster[0].size` cannot be updated at the same time.
* @property location The Azure Region where the Azure VMware Solution Private Cloud should exist. Changing this forces a new Azure VMware Solution Private Cloud to be created.
* @property managementCluster A `management_cluster` block as defined below.
* > **NOTE :** `internet_connection_enabled` and `management_cluster[0].size` cannot be updated at the same time.
* @property name The name which should be used for this Azure VMware Solution Private Cloud. Changing this forces a new Azure VMware Solution Private Cloud to be created.
* @property networkSubnetCidr The subnet which should be unique across virtual network in your subscription as well as on-premise. Changing this forces a new Azure VMware Solution Private Cloud to be created.
* @property nsxtPassword The password of the VMware NSX Manager cloudadmin. Changing this forces a new Azure VMware Solution Private Cloud to be created.
* @property resourceGroupName The name of the Resource Group where the Azure VMware Solution Private Cloud should exist. Changing this forces a new Azure VMware Solution Private Cloud to be created.
* @property skuName The Name of the SKU used for this Azure VMware Solution Private Cloud. Possible values are `av20`, `av36`, `av36t`, `av36p`, `av36pt`, `av52`, `av52t`, and `av64`. Changing this forces a new Azure VMware Solution Private Cloud to be created.
* @property tags A mapping of tags which should be assigned to the Azure VMware Solution Private Cloud.
* @property vcenterPassword The password of the VMware vCenter Server cloudadmin. Changing this forces a new Azure VMware Solution Private Cloud to be created.
*/
public data class PrivateCloudArgs(
public val internetConnectionEnabled: Output? = null,
public val location: Output? = null,
public val managementCluster: Output? = null,
public val name: Output? = null,
public val networkSubnetCidr: Output? = null,
public val nsxtPassword: Output? = null,
public val resourceGroupName: Output? = null,
public val skuName: Output? = null,
public val tags: Output
© 2015 - 2025 Weber Informatics LLC | Privacy Policy