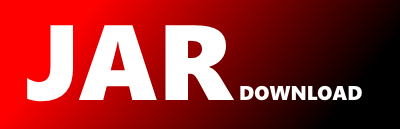
com.pulumi.azure.backup.kotlin.inputs.PolicyVMWorkloadProtectionPolicyArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.backup.kotlin.inputs
import com.pulumi.azure.backup.inputs.PolicyVMWorkloadProtectionPolicyArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property backup A `backup` block as defined below.
* @property policyType The type of the VM Workload Backup Policy. Possible values are `Differential`, `Full`, `Incremental` and `Log`.
* @property retentionDaily A `retention_daily` block as defined below.
* @property retentionMonthly A `retention_monthly` block as defined below.
* @property retentionWeekly A `retention_weekly` block as defined below.
* @property retentionYearly A `retention_yearly` block as defined below.
* @property simpleRetention A `simple_retention` block as defined below.
*/
public data class PolicyVMWorkloadProtectionPolicyArgs(
public val backup: Output,
public val policyType: Output,
public val retentionDaily: Output? = null,
public val retentionMonthly: Output? = null,
public val retentionWeekly: Output? = null,
public val retentionYearly: Output? = null,
public val simpleRetention: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.backup.inputs.PolicyVMWorkloadProtectionPolicyArgs =
com.pulumi.azure.backup.inputs.PolicyVMWorkloadProtectionPolicyArgs.builder()
.backup(backup.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.policyType(policyType.applyValue({ args0 -> args0 }))
.retentionDaily(retentionDaily?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retentionMonthly(retentionMonthly?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retentionWeekly(retentionWeekly?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.retentionYearly(retentionYearly?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.simpleRetention(
simpleRetention?.applyValue({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
}),
).build()
}
/**
* Builder for [PolicyVMWorkloadProtectionPolicyArgs].
*/
@PulumiTagMarker
public class PolicyVMWorkloadProtectionPolicyArgsBuilder internal constructor() {
private var backup: Output? = null
private var policyType: Output? = null
private var retentionDaily: Output? = null
private var retentionMonthly: Output? = null
private var retentionWeekly: Output? = null
private var retentionYearly: Output? = null
private var simpleRetention: Output? = null
/**
* @param value A `backup` block as defined below.
*/
@JvmName("xinmlqguloulvngp")
public suspend fun backup(`value`: Output) {
this.backup = value
}
/**
* @param value The type of the VM Workload Backup Policy. Possible values are `Differential`, `Full`, `Incremental` and `Log`.
*/
@JvmName("funwkyqeeenvvnhs")
public suspend fun policyType(`value`: Output) {
this.policyType = value
}
/**
* @param value A `retention_daily` block as defined below.
*/
@JvmName("thtkotqeqkghxrbu")
public suspend
fun retentionDaily(`value`: Output) {
this.retentionDaily = value
}
/**
* @param value A `retention_monthly` block as defined below.
*/
@JvmName("rugfhautqeyafdwv")
public suspend
fun retentionMonthly(`value`: Output) {
this.retentionMonthly = value
}
/**
* @param value A `retention_weekly` block as defined below.
*/
@JvmName("xatbybpxsslyiawm")
public suspend
fun retentionWeekly(`value`: Output) {
this.retentionWeekly = value
}
/**
* @param value A `retention_yearly` block as defined below.
*/
@JvmName("nxamkfqypjavjppt")
public suspend
fun retentionYearly(`value`: Output) {
this.retentionYearly = value
}
/**
* @param value A `simple_retention` block as defined below.
*/
@JvmName("jklvalccoqnswdht")
public suspend
fun simpleRetention(`value`: Output) {
this.simpleRetention = value
}
/**
* @param value A `backup` block as defined below.
*/
@JvmName("kvpqdlwjkkbtmmni")
public suspend fun backup(`value`: PolicyVMWorkloadProtectionPolicyBackupArgs) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.backup = mapped
}
/**
* @param argument A `backup` block as defined below.
*/
@JvmName("dfiouwijhsgbyjwe")
public suspend
fun backup(argument: suspend PolicyVMWorkloadProtectionPolicyBackupArgsBuilder.() -> Unit) {
val toBeMapped = PolicyVMWorkloadProtectionPolicyBackupArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.backup = mapped
}
/**
* @param value The type of the VM Workload Backup Policy. Possible values are `Differential`, `Full`, `Incremental` and `Log`.
*/
@JvmName("pkivxtsmpbtefcmi")
public suspend fun policyType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.policyType = mapped
}
/**
* @param value A `retention_daily` block as defined below.
*/
@JvmName("vtkssdymhnugiymm")
public suspend fun retentionDaily(`value`: PolicyVMWorkloadProtectionPolicyRetentionDailyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retentionDaily = mapped
}
/**
* @param argument A `retention_daily` block as defined below.
*/
@JvmName("isdwskdsqabfbdic")
public suspend
fun retentionDaily(argument: suspend PolicyVMWorkloadProtectionPolicyRetentionDailyArgsBuilder.() -> Unit) {
val toBeMapped = PolicyVMWorkloadProtectionPolicyRetentionDailyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.retentionDaily = mapped
}
/**
* @param value A `retention_monthly` block as defined below.
*/
@JvmName("vcehfgenuqrbadvx")
public suspend
fun retentionMonthly(`value`: PolicyVMWorkloadProtectionPolicyRetentionMonthlyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retentionMonthly = mapped
}
/**
* @param argument A `retention_monthly` block as defined below.
*/
@JvmName("pwdgrxxrujcumhvv")
public suspend
fun retentionMonthly(argument: suspend PolicyVMWorkloadProtectionPolicyRetentionMonthlyArgsBuilder.() -> Unit) {
val toBeMapped = PolicyVMWorkloadProtectionPolicyRetentionMonthlyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.retentionMonthly = mapped
}
/**
* @param value A `retention_weekly` block as defined below.
*/
@JvmName("dkcmehrpiegyksux")
public suspend
fun retentionWeekly(`value`: PolicyVMWorkloadProtectionPolicyRetentionWeeklyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retentionWeekly = mapped
}
/**
* @param argument A `retention_weekly` block as defined below.
*/
@JvmName("lqwkcbstcijmytom")
public suspend
fun retentionWeekly(argument: suspend PolicyVMWorkloadProtectionPolicyRetentionWeeklyArgsBuilder.() -> Unit) {
val toBeMapped = PolicyVMWorkloadProtectionPolicyRetentionWeeklyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.retentionWeekly = mapped
}
/**
* @param value A `retention_yearly` block as defined below.
*/
@JvmName("wahskvcnnejkmdbt")
public suspend
fun retentionYearly(`value`: PolicyVMWorkloadProtectionPolicyRetentionYearlyArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.retentionYearly = mapped
}
/**
* @param argument A `retention_yearly` block as defined below.
*/
@JvmName("qubymkjfjekcwqil")
public suspend
fun retentionYearly(argument: suspend PolicyVMWorkloadProtectionPolicyRetentionYearlyArgsBuilder.() -> Unit) {
val toBeMapped = PolicyVMWorkloadProtectionPolicyRetentionYearlyArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.retentionYearly = mapped
}
/**
* @param value A `simple_retention` block as defined below.
*/
@JvmName("tseoqooolciyyyun")
public suspend
fun simpleRetention(`value`: PolicyVMWorkloadProtectionPolicySimpleRetentionArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.simpleRetention = mapped
}
/**
* @param argument A `simple_retention` block as defined below.
*/
@JvmName("okhnmxqwgcujqnip")
public suspend
fun simpleRetention(argument: suspend PolicyVMWorkloadProtectionPolicySimpleRetentionArgsBuilder.() -> Unit) {
val toBeMapped = PolicyVMWorkloadProtectionPolicySimpleRetentionArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.simpleRetention = mapped
}
internal fun build(): PolicyVMWorkloadProtectionPolicyArgs = PolicyVMWorkloadProtectionPolicyArgs(
backup = backup ?: throw PulumiNullFieldException("backup"),
policyType = policyType ?: throw PulumiNullFieldException("policyType"),
retentionDaily = retentionDaily,
retentionMonthly = retentionMonthly,
retentionWeekly = retentionWeekly,
retentionYearly = retentionYearly,
simpleRetention = simpleRetention,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy