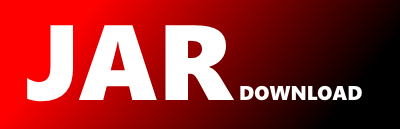
com.pulumi.azure.backup.kotlin.inputs.PolicyVMWorkloadProtectionPolicyBackupArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.backup.kotlin.inputs
import com.pulumi.azure.backup.inputs.PolicyVMWorkloadProtectionPolicyBackupArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property frequency The backup frequency for the VM Workload Backup Policy. Possible values are `Daily` and `Weekly`.
* @property frequencyInMinutes The backup frequency in minutes for the VM Workload Backup Policy. Possible values are `15`, `30`, `60`, `120`, `240`, `480`, `720` and `1440`.
* @property time The time of day to perform the backup in 24hour format.
* @property weekdays The days of the week to perform backups on. Possible values are `Sunday`, `Monday`, `Tuesday`, `Wednesday`, `Thursday`, `Friday` or `Saturday`. This is used when `frequency` is `Weekly`.
*/
public data class PolicyVMWorkloadProtectionPolicyBackupArgs(
public val frequency: Output? = null,
public val frequencyInMinutes: Output? = null,
public val time: Output? = null,
public val weekdays: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.backup.inputs.PolicyVMWorkloadProtectionPolicyBackupArgs =
com.pulumi.azure.backup.inputs.PolicyVMWorkloadProtectionPolicyBackupArgs.builder()
.frequency(frequency?.applyValue({ args0 -> args0 }))
.frequencyInMinutes(frequencyInMinutes?.applyValue({ args0 -> args0 }))
.time(time?.applyValue({ args0 -> args0 }))
.weekdays(weekdays?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [PolicyVMWorkloadProtectionPolicyBackupArgs].
*/
@PulumiTagMarker
public class PolicyVMWorkloadProtectionPolicyBackupArgsBuilder internal constructor() {
private var frequency: Output? = null
private var frequencyInMinutes: Output? = null
private var time: Output? = null
private var weekdays: Output>? = null
/**
* @param value The backup frequency for the VM Workload Backup Policy. Possible values are `Daily` and `Weekly`.
*/
@JvmName("tedmbbbnexijnhuj")
public suspend fun frequency(`value`: Output) {
this.frequency = value
}
/**
* @param value The backup frequency in minutes for the VM Workload Backup Policy. Possible values are `15`, `30`, `60`, `120`, `240`, `480`, `720` and `1440`.
*/
@JvmName("jbekbgwkludiykfw")
public suspend fun frequencyInMinutes(`value`: Output) {
this.frequencyInMinutes = value
}
/**
* @param value The time of day to perform the backup in 24hour format.
*/
@JvmName("cwtgjyvbfeimmasy")
public suspend fun time(`value`: Output) {
this.time = value
}
/**
* @param value The days of the week to perform backups on. Possible values are `Sunday`, `Monday`, `Tuesday`, `Wednesday`, `Thursday`, `Friday` or `Saturday`. This is used when `frequency` is `Weekly`.
*/
@JvmName("kgjwxsmpglhfecpj")
public suspend fun weekdays(`value`: Output>) {
this.weekdays = value
}
@JvmName("yrcwyhlxopwoabqs")
public suspend fun weekdays(vararg values: Output) {
this.weekdays = Output.all(values.asList())
}
/**
* @param values The days of the week to perform backups on. Possible values are `Sunday`, `Monday`, `Tuesday`, `Wednesday`, `Thursday`, `Friday` or `Saturday`. This is used when `frequency` is `Weekly`.
*/
@JvmName("tenhxcjjqnudtgxi")
public suspend fun weekdays(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy