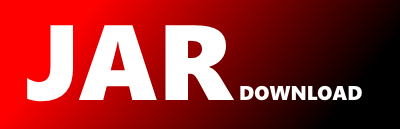
com.pulumi.azure.billing.kotlin.AccountCostManagementExport.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.billing.kotlin
import com.pulumi.azure.billing.kotlin.outputs.AccountCostManagementExportExportDataOptions
import com.pulumi.azure.billing.kotlin.outputs.AccountCostManagementExportExportDataStorageLocation
import com.pulumi.core.Output
import com.pulumi.kotlin.KotlinCustomResource
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.ResourceMapper
import com.pulumi.kotlin.options.CustomResourceOptions
import com.pulumi.kotlin.options.CustomResourceOptionsBuilder
import com.pulumi.resources.Resource
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import com.pulumi.azure.billing.kotlin.outputs.AccountCostManagementExportExportDataOptions.Companion.toKotlin as accountCostManagementExportExportDataOptionsToKotlin
import com.pulumi.azure.billing.kotlin.outputs.AccountCostManagementExportExportDataStorageLocation.Companion.toKotlin as accountCostManagementExportExportDataStorageLocationToKotlin
/**
* Builder for [AccountCostManagementExport].
*/
@PulumiTagMarker
public class AccountCostManagementExportResourceBuilder internal constructor() {
public var name: String? = null
public var args: AccountCostManagementExportArgs = AccountCostManagementExportArgs()
public var opts: CustomResourceOptions = CustomResourceOptions()
/**
* @param name The _unique_ name of the resulting resource.
*/
public fun name(`value`: String) {
this.name = value
}
/**
* @param block The arguments to use to populate this resource's properties.
*/
public suspend fun args(block: suspend AccountCostManagementExportArgsBuilder.() -> Unit) {
val builder = AccountCostManagementExportArgsBuilder()
block(builder)
this.args = builder.build()
}
/**
* @param block A bag of options that control this resource's behavior.
*/
public suspend fun opts(block: suspend CustomResourceOptionsBuilder.() -> Unit) {
this.opts = com.pulumi.kotlin.options.CustomResourceOptions.opts(block)
}
internal fun build(): AccountCostManagementExport {
val builtJavaResource =
com.pulumi.azure.billing.AccountCostManagementExport(
this.name,
this.args.toJava(),
this.opts.toJava(),
)
return AccountCostManagementExport(builtJavaResource)
}
}
/**
* Manages a Cost Management Export for a Billing Account.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-resources",
* location: "West Europe",
* });
* const exampleAccount = new azure.storage.Account("example", {
* name: "example",
* resourceGroupName: example.name,
* location: example.location,
* accountTier: "Standard",
* accountReplicationType: "LRS",
* });
* const exampleContainer = new azure.storage.Container("example", {
* name: "examplecontainer",
* storageAccountName: exampleAccount.name,
* });
* const exampleAccountCostManagementExport = new azure.billing.AccountCostManagementExport("example", {
* name: "example",
* billingAccountId: "example",
* recurrenceType: "Monthly",
* recurrencePeriodStartDate: "2020-08-18T00:00:00Z",
* recurrencePeriodEndDate: "2020-09-18T00:00:00Z",
* exportDataStorageLocation: {
* containerId: exampleContainer.resourceManagerId,
* rootFolderPath: "/root/updated",
* },
* exportDataOptions: {
* type: "Usage",
* timeFrame: "WeekToDate",
* },
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-resources",
* location="West Europe")
* example_account = azure.storage.Account("example",
* name="example",
* resource_group_name=example.name,
* location=example.location,
* account_tier="Standard",
* account_replication_type="LRS")
* example_container = azure.storage.Container("example",
* name="examplecontainer",
* storage_account_name=example_account.name)
* example_account_cost_management_export = azure.billing.AccountCostManagementExport("example",
* name="example",
* billing_account_id="example",
* recurrence_type="Monthly",
* recurrence_period_start_date="2020-08-18T00:00:00Z",
* recurrence_period_end_date="2020-09-18T00:00:00Z",
* export_data_storage_location=azure.billing.AccountCostManagementExportExportDataStorageLocationArgs(
* container_id=example_container.resource_manager_id,
* root_folder_path="/root/updated",
* ),
* export_data_options=azure.billing.AccountCostManagementExportExportDataOptionsArgs(
* type="Usage",
* time_frame="WeekToDate",
* ))
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-resources",
* Location = "West Europe",
* });
* var exampleAccount = new Azure.Storage.Account("example", new()
* {
* Name = "example",
* ResourceGroupName = example.Name,
* Location = example.Location,
* AccountTier = "Standard",
* AccountReplicationType = "LRS",
* });
* var exampleContainer = new Azure.Storage.Container("example", new()
* {
* Name = "examplecontainer",
* StorageAccountName = exampleAccount.Name,
* });
* var exampleAccountCostManagementExport = new Azure.Billing.AccountCostManagementExport("example", new()
* {
* Name = "example",
* BillingAccountId = "example",
* RecurrenceType = "Monthly",
* RecurrencePeriodStartDate = "2020-08-18T00:00:00Z",
* RecurrencePeriodEndDate = "2020-09-18T00:00:00Z",
* ExportDataStorageLocation = new Azure.Billing.Inputs.AccountCostManagementExportExportDataStorageLocationArgs
* {
* ContainerId = exampleContainer.ResourceManagerId,
* RootFolderPath = "/root/updated",
* },
* ExportDataOptions = new Azure.Billing.Inputs.AccountCostManagementExportExportDataOptionsArgs
* {
* Type = "Usage",
* TimeFrame = "WeekToDate",
* },
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/billing"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/storage"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-resources"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleAccount, err := storage.NewAccount(ctx, "example", &storage.AccountArgs{
* Name: pulumi.String("example"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* AccountTier: pulumi.String("Standard"),
* AccountReplicationType: pulumi.String("LRS"),
* })
* if err != nil {
* return err
* }
* exampleContainer, err := storage.NewContainer(ctx, "example", &storage.ContainerArgs{
* Name: pulumi.String("examplecontainer"),
* StorageAccountName: exampleAccount.Name,
* })
* if err != nil {
* return err
* }
* _, err = billing.NewAccountCostManagementExport(ctx, "example", &billing.AccountCostManagementExportArgs{
* Name: pulumi.String("example"),
* BillingAccountId: pulumi.String("example"),
* RecurrenceType: pulumi.String("Monthly"),
* RecurrencePeriodStartDate: pulumi.String("2020-08-18T00:00:00Z"),
* RecurrencePeriodEndDate: pulumi.String("2020-09-18T00:00:00Z"),
* ExportDataStorageLocation: &billing.AccountCostManagementExportExportDataStorageLocationArgs{
* ContainerId: exampleContainer.ResourceManagerId,
* RootFolderPath: pulumi.String("/root/updated"),
* },
* ExportDataOptions: &billing.AccountCostManagementExportExportDataOptionsArgs{
* Type: pulumi.String("Usage"),
* TimeFrame: pulumi.String("WeekToDate"),
* },
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.storage.Account;
* import com.pulumi.azure.storage.AccountArgs;
* import com.pulumi.azure.storage.Container;
* import com.pulumi.azure.storage.ContainerArgs;
* import com.pulumi.azure.billing.AccountCostManagementExport;
* import com.pulumi.azure.billing.AccountCostManagementExportArgs;
* import com.pulumi.azure.billing.inputs.AccountCostManagementExportExportDataStorageLocationArgs;
* import com.pulumi.azure.billing.inputs.AccountCostManagementExportExportDataOptionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-resources")
* .location("West Europe")
* .build());
* var exampleAccount = new Account("exampleAccount", AccountArgs.builder()
* .name("example")
* .resourceGroupName(example.name())
* .location(example.location())
* .accountTier("Standard")
* .accountReplicationType("LRS")
* .build());
* var exampleContainer = new Container("exampleContainer", ContainerArgs.builder()
* .name("examplecontainer")
* .storageAccountName(exampleAccount.name())
* .build());
* var exampleAccountCostManagementExport = new AccountCostManagementExport("exampleAccountCostManagementExport", AccountCostManagementExportArgs.builder()
* .name("example")
* .billingAccountId("example")
* .recurrenceType("Monthly")
* .recurrencePeriodStartDate("2020-08-18T00:00:00Z")
* .recurrencePeriodEndDate("2020-09-18T00:00:00Z")
* .exportDataStorageLocation(AccountCostManagementExportExportDataStorageLocationArgs.builder()
* .containerId(exampleContainer.resourceManagerId())
* .rootFolderPath("/root/updated")
* .build())
* .exportDataOptions(AccountCostManagementExportExportDataOptionsArgs.builder()
* .type("Usage")
* .timeFrame("WeekToDate")
* .build())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-resources
* location: West Europe
* exampleAccount:
* type: azure:storage:Account
* name: example
* properties:
* name: example
* resourceGroupName: ${example.name}
* location: ${example.location}
* accountTier: Standard
* accountReplicationType: LRS
* exampleContainer:
* type: azure:storage:Container
* name: example
* properties:
* name: examplecontainer
* storageAccountName: ${exampleAccount.name}
* exampleAccountCostManagementExport:
* type: azure:billing:AccountCostManagementExport
* name: example
* properties:
* name: example
* billingAccountId: example
* recurrenceType: Monthly
* recurrencePeriodStartDate: 2020-08-18T00:00:00Z
* recurrencePeriodEndDate: 2020-09-18T00:00:00Z
* exportDataStorageLocation:
* containerId: ${exampleContainer.resourceManagerId}
* rootFolderPath: /root/updated
* exportDataOptions:
* type: Usage
* timeFrame: WeekToDate
* ```
*
* ## Import
* Billing Account Cost Management Exports can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:billing/accountCostManagementExport:AccountCostManagementExport example /providers/Microsoft.Billing/billingAccounts/12345678/providers/Microsoft.CostManagement/exports/export1
* ```
*/
public class AccountCostManagementExport internal constructor(
override val javaResource: com.pulumi.azure.billing.AccountCostManagementExport,
) : KotlinCustomResource(javaResource, AccountCostManagementExportMapper) {
/**
* Is the cost management export active? Default is `true`.
*/
public val active: Output?
get() = javaResource.active().applyValue({ args0 -> args0.map({ args0 -> args0 }).orElse(null) })
/**
* The id of the billing account on which to create an export. Changing this forces a new resource to be created.
*/
public val billingAccountId: Output
get() = javaResource.billingAccountId().applyValue({ args0 -> args0 })
/**
* A `export_data_options` block as defined below.
*/
public val exportDataOptions: Output
get() = javaResource.exportDataOptions().applyValue({ args0 ->
args0.let({ args0 ->
accountCostManagementExportExportDataOptionsToKotlin(args0)
})
})
/**
* A `export_data_storage_location` block as defined below.
*/
public val exportDataStorageLocation: Output
get() = javaResource.exportDataStorageLocation().applyValue({ args0 ->
args0.let({ args0 ->
accountCostManagementExportExportDataStorageLocationToKotlin(args0)
})
})
/**
* Specifies the name of the Cost Management Export. Changing this forces a new resource to be created.
*/
public val name: Output
get() = javaResource.name().applyValue({ args0 -> args0 })
/**
* The date the export will stop capturing information.
*/
public val recurrencePeriodEndDate: Output
get() = javaResource.recurrencePeriodEndDate().applyValue({ args0 -> args0 })
/**
* The date the export will start capturing information.
*/
public val recurrencePeriodStartDate: Output
get() = javaResource.recurrencePeriodStartDate().applyValue({ args0 -> args0 })
/**
* How often the requested information will be exported. Valid values include `Annually`, `Daily`, `Monthly`, `Weekly`.
*/
public val recurrenceType: Output
get() = javaResource.recurrenceType().applyValue({ args0 -> args0 })
}
public object AccountCostManagementExportMapper : ResourceMapper {
override fun supportsMappingOfType(javaResource: Resource): Boolean =
com.pulumi.azure.billing.AccountCostManagementExport::class == javaResource::class
override fun map(javaResource: Resource): AccountCostManagementExport =
AccountCostManagementExport(
javaResource as
com.pulumi.azure.billing.AccountCostManagementExport,
)
}
/**
* @see [AccountCostManagementExport].
* @param name The _unique_ name of the resulting resource.
* @param block Builder for [AccountCostManagementExport].
*/
public suspend fun accountCostManagementExport(
name: String,
block: suspend AccountCostManagementExportResourceBuilder.() -> Unit,
):
AccountCostManagementExport {
val builder = AccountCostManagementExportResourceBuilder()
builder.name(name)
block(builder)
return builder.build()
}
/**
* @see [AccountCostManagementExport].
* @param name The _unique_ name of the resulting resource.
*/
public fun accountCostManagementExport(name: String): AccountCostManagementExport {
val builder = AccountCostManagementExportResourceBuilder()
builder.name(name)
return builder.build()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy