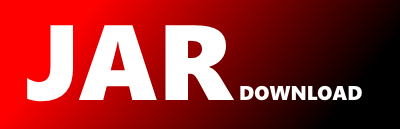
com.pulumi.azure.cdn.kotlin.inputs.FrontdoorOriginGroupHealthProbeArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.cdn.kotlin.inputs
import com.pulumi.azure.cdn.inputs.FrontdoorOriginGroupHealthProbeArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property intervalInSeconds Specifies the number of seconds between health probes. Possible values are between `5` and `31536000` seconds (inclusive).
* @property path Specifies the path relative to the origin that is used to determine the health of the origin. Defaults to `/`.
* > **NOTE:** Health probes can only be disabled if there is a single enabled origin in a single enabled origin group. For more information about the `health_probe` settings please see the [product documentation](https://docs.microsoft.com/azure/frontdoor/health-probes).
* @property protocol Specifies the protocol to use for health probe. Possible values are `Http` and `Https`.
* @property requestType Specifies the type of health probe request that is made. Possible values are `GET` and `HEAD`. Defaults to `HEAD`.
*/
public data class FrontdoorOriginGroupHealthProbeArgs(
public val intervalInSeconds: Output,
public val path: Output? = null,
public val protocol: Output,
public val requestType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.cdn.inputs.FrontdoorOriginGroupHealthProbeArgs =
com.pulumi.azure.cdn.inputs.FrontdoorOriginGroupHealthProbeArgs.builder()
.intervalInSeconds(intervalInSeconds.applyValue({ args0 -> args0 }))
.path(path?.applyValue({ args0 -> args0 }))
.protocol(protocol.applyValue({ args0 -> args0 }))
.requestType(requestType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [FrontdoorOriginGroupHealthProbeArgs].
*/
@PulumiTagMarker
public class FrontdoorOriginGroupHealthProbeArgsBuilder internal constructor() {
private var intervalInSeconds: Output? = null
private var path: Output? = null
private var protocol: Output? = null
private var requestType: Output? = null
/**
* @param value Specifies the number of seconds between health probes. Possible values are between `5` and `31536000` seconds (inclusive).
*/
@JvmName("yflatqwjmpwpjmnn")
public suspend fun intervalInSeconds(`value`: Output) {
this.intervalInSeconds = value
}
/**
* @param value Specifies the path relative to the origin that is used to determine the health of the origin. Defaults to `/`.
* > **NOTE:** Health probes can only be disabled if there is a single enabled origin in a single enabled origin group. For more information about the `health_probe` settings please see the [product documentation](https://docs.microsoft.com/azure/frontdoor/health-probes).
*/
@JvmName("pxluhwlpyxfjsbyw")
public suspend fun path(`value`: Output) {
this.path = value
}
/**
* @param value Specifies the protocol to use for health probe. Possible values are `Http` and `Https`.
*/
@JvmName("pcvldbersrjxtloq")
public suspend fun protocol(`value`: Output) {
this.protocol = value
}
/**
* @param value Specifies the type of health probe request that is made. Possible values are `GET` and `HEAD`. Defaults to `HEAD`.
*/
@JvmName("yrfbdnqrqnwubjqm")
public suspend fun requestType(`value`: Output) {
this.requestType = value
}
/**
* @param value Specifies the number of seconds between health probes. Possible values are between `5` and `31536000` seconds (inclusive).
*/
@JvmName("eiiyvfryekcwuxtj")
public suspend fun intervalInSeconds(`value`: Int) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.intervalInSeconds = mapped
}
/**
* @param value Specifies the path relative to the origin that is used to determine the health of the origin. Defaults to `/`.
* > **NOTE:** Health probes can only be disabled if there is a single enabled origin in a single enabled origin group. For more information about the `health_probe` settings please see the [product documentation](https://docs.microsoft.com/azure/frontdoor/health-probes).
*/
@JvmName("bflxvvxkroxbmkrc")
public suspend fun path(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.path = mapped
}
/**
* @param value Specifies the protocol to use for health probe. Possible values are `Http` and `Https`.
*/
@JvmName("upweheppbywgwtrh")
public suspend fun protocol(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.protocol = mapped
}
/**
* @param value Specifies the type of health probe request that is made. Possible values are `GET` and `HEAD`. Defaults to `HEAD`.
*/
@JvmName("bxwjwfyjgsjitxnu")
public suspend fun requestType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.requestType = mapped
}
internal fun build(): FrontdoorOriginGroupHealthProbeArgs = FrontdoorOriginGroupHealthProbeArgs(
intervalInSeconds = intervalInSeconds ?: throw PulumiNullFieldException("intervalInSeconds"),
path = path,
protocol = protocol ?: throw PulumiNullFieldException("protocol"),
requestType = requestType,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy