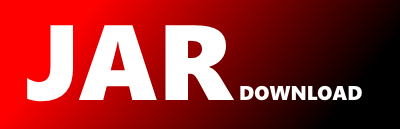
com.pulumi.azure.cdn.kotlin.inputs.FrontdoorRuleActionsRouteConfigurationOverrideActionArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.cdn.kotlin.inputs
import com.pulumi.azure.cdn.inputs.FrontdoorRuleActionsRouteConfigurationOverrideActionArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property cacheBehavior `HonorOrigin` the Front Door will always honor origin response header directive. If the origin directive is missing, Front Door will cache contents anywhere from `1` to `3` days. `OverrideAlways` the TTL value returned from your Front Door Origin is overwritten with the value specified in the action. This behavior will only be applied if the response is cacheable. `OverrideIfOriginMissing` if no TTL value gets returned from your Front Door Origin, the rule sets the TTL to the value specified in the action. This behavior will only be applied if the response is cacheable. `Disabled` the Front Door will not cache the response contents, irrespective of Front Door Origin response directives. Possible values include `HonorOrigin`, `OverrideAlways`, `OverrideIfOriginMissing` or `Disabled`.
* @property cacheDuration When Cache behavior is set to `Override` or `SetIfMissing`, this field specifies the cache duration to use. The maximum duration is 366 days specified in the `d.HH:MM:SS` format(e.g. `365.23:59:59`). If the desired maximum cache duration is less than 1 day then the maximum cache duration should be specified in the `HH:MM:SS` format(e.g. `23:59:59`).
* @property cdnFrontdoorOriginGroupId The Front Door Origin Group resource ID that the request should be routed to. This overrides the configuration specified in the Front Door Endpoint route.
* @property compressionEnabled Should the Front Door dynamically compress the content? Possible values include `true` or `false`.
* ->**NOTE:** Content won't be compressed on AzureFrontDoor when requested content is smaller than `1 byte` or larger than `1 MB`.
* @property forwardingProtocol The forwarding protocol the request will be redirected as. This overrides the configuration specified in the route to be associated with. Possible values include `MatchRequest`, `HttpOnly` or `HttpsOnly`.
* ->**NOTE:** If the `cdn_frontdoor_origin_group_id` is not defined you cannot set the `forwarding_protocol`.
* @property queryStringCachingBehavior `IncludeSpecifiedQueryStrings` query strings specified in the `query_string_parameters` field get included when the cache key gets generated. `UseQueryString` cache every unique URL, each unique URL will have its own cache key. `IgnoreSpecifiedQueryStrings` query strings specified in the `query_string_parameters` field get excluded when the cache key gets generated. `IgnoreQueryString` query strings aren't considered when the cache key gets generated. Possible values include `IgnoreQueryString`, `UseQueryString`, `IgnoreSpecifiedQueryStrings` or `IncludeSpecifiedQueryStrings`.
* @property queryStringParameters A list of query string parameter names.
* ->**NOTE:** `query_string_parameters` is a required field when the `query_string_caching_behavior` is set to `IncludeSpecifiedQueryStrings` or `IgnoreSpecifiedQueryStrings`.
*/
public data class FrontdoorRuleActionsRouteConfigurationOverrideActionArgs(
public val cacheBehavior: Output? = null,
public val cacheDuration: Output? = null,
public val cdnFrontdoorOriginGroupId: Output? = null,
public val compressionEnabled: Output? = null,
public val forwardingProtocol: Output? = null,
public val queryStringCachingBehavior: Output? = null,
public val queryStringParameters: Output>? = null,
) :
ConvertibleToJava {
override fun toJava():
com.pulumi.azure.cdn.inputs.FrontdoorRuleActionsRouteConfigurationOverrideActionArgs =
com.pulumi.azure.cdn.inputs.FrontdoorRuleActionsRouteConfigurationOverrideActionArgs.builder()
.cacheBehavior(cacheBehavior?.applyValue({ args0 -> args0 }))
.cacheDuration(cacheDuration?.applyValue({ args0 -> args0 }))
.cdnFrontdoorOriginGroupId(cdnFrontdoorOriginGroupId?.applyValue({ args0 -> args0 }))
.compressionEnabled(compressionEnabled?.applyValue({ args0 -> args0 }))
.forwardingProtocol(forwardingProtocol?.applyValue({ args0 -> args0 }))
.queryStringCachingBehavior(queryStringCachingBehavior?.applyValue({ args0 -> args0 }))
.queryStringParameters(
queryStringParameters?.applyValue({ args0 ->
args0.map({ args0 ->
args0
})
}),
).build()
}
/**
* Builder for [FrontdoorRuleActionsRouteConfigurationOverrideActionArgs].
*/
@PulumiTagMarker
public class FrontdoorRuleActionsRouteConfigurationOverrideActionArgsBuilder internal constructor() {
private var cacheBehavior: Output? = null
private var cacheDuration: Output? = null
private var cdnFrontdoorOriginGroupId: Output? = null
private var compressionEnabled: Output? = null
private var forwardingProtocol: Output? = null
private var queryStringCachingBehavior: Output? = null
private var queryStringParameters: Output>? = null
/**
* @param value `HonorOrigin` the Front Door will always honor origin response header directive. If the origin directive is missing, Front Door will cache contents anywhere from `1` to `3` days. `OverrideAlways` the TTL value returned from your Front Door Origin is overwritten with the value specified in the action. This behavior will only be applied if the response is cacheable. `OverrideIfOriginMissing` if no TTL value gets returned from your Front Door Origin, the rule sets the TTL to the value specified in the action. This behavior will only be applied if the response is cacheable. `Disabled` the Front Door will not cache the response contents, irrespective of Front Door Origin response directives. Possible values include `HonorOrigin`, `OverrideAlways`, `OverrideIfOriginMissing` or `Disabled`.
*/
@JvmName("fdftudcytqrbbjqn")
public suspend fun cacheBehavior(`value`: Output) {
this.cacheBehavior = value
}
/**
* @param value When Cache behavior is set to `Override` or `SetIfMissing`, this field specifies the cache duration to use. The maximum duration is 366 days specified in the `d.HH:MM:SS` format(e.g. `365.23:59:59`). If the desired maximum cache duration is less than 1 day then the maximum cache duration should be specified in the `HH:MM:SS` format(e.g. `23:59:59`).
*/
@JvmName("bkludkltqwedmufv")
public suspend fun cacheDuration(`value`: Output) {
this.cacheDuration = value
}
/**
* @param value The Front Door Origin Group resource ID that the request should be routed to. This overrides the configuration specified in the Front Door Endpoint route.
*/
@JvmName("qyvfadotmdpxxicf")
public suspend fun cdnFrontdoorOriginGroupId(`value`: Output) {
this.cdnFrontdoorOriginGroupId = value
}
/**
* @param value Should the Front Door dynamically compress the content? Possible values include `true` or `false`.
* ->**NOTE:** Content won't be compressed on AzureFrontDoor when requested content is smaller than `1 byte` or larger than `1 MB`.
*/
@JvmName("hboqhxteskbpxwwa")
public suspend fun compressionEnabled(`value`: Output) {
this.compressionEnabled = value
}
/**
* @param value The forwarding protocol the request will be redirected as. This overrides the configuration specified in the route to be associated with. Possible values include `MatchRequest`, `HttpOnly` or `HttpsOnly`.
* ->**NOTE:** If the `cdn_frontdoor_origin_group_id` is not defined you cannot set the `forwarding_protocol`.
*/
@JvmName("ctgcrgovhlybnfku")
public suspend fun forwardingProtocol(`value`: Output) {
this.forwardingProtocol = value
}
/**
* @param value `IncludeSpecifiedQueryStrings` query strings specified in the `query_string_parameters` field get included when the cache key gets generated. `UseQueryString` cache every unique URL, each unique URL will have its own cache key. `IgnoreSpecifiedQueryStrings` query strings specified in the `query_string_parameters` field get excluded when the cache key gets generated. `IgnoreQueryString` query strings aren't considered when the cache key gets generated. Possible values include `IgnoreQueryString`, `UseQueryString`, `IgnoreSpecifiedQueryStrings` or `IncludeSpecifiedQueryStrings`.
*/
@JvmName("oqeckgplfrnqsooa")
public suspend fun queryStringCachingBehavior(`value`: Output) {
this.queryStringCachingBehavior = value
}
/**
* @param value A list of query string parameter names.
* ->**NOTE:** `query_string_parameters` is a required field when the `query_string_caching_behavior` is set to `IncludeSpecifiedQueryStrings` or `IgnoreSpecifiedQueryStrings`.
*/
@JvmName("lvbhachxyoutiikg")
public suspend fun queryStringParameters(`value`: Output>) {
this.queryStringParameters = value
}
@JvmName("sbulolffbgkfqvvb")
public suspend fun queryStringParameters(vararg values: Output) {
this.queryStringParameters = Output.all(values.asList())
}
/**
* @param values A list of query string parameter names.
* ->**NOTE:** `query_string_parameters` is a required field when the `query_string_caching_behavior` is set to `IncludeSpecifiedQueryStrings` or `IgnoreSpecifiedQueryStrings`.
*/
@JvmName("lpeutcuwbkcrwriv")
public suspend fun queryStringParameters(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy