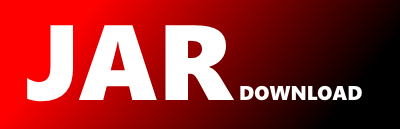
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRule.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.cdn.kotlin.outputs
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
/**
*
* @property cacheExpirationAction A `cache_expiration_action` block as defined above.
* @property cacheKeyQueryStringAction A `cache_key_query_string_action` block as defined above.
* @property cookiesConditions A `cookies_condition` block as defined above.
* @property deviceCondition A `device_condition` block as defined below.
* @property httpVersionConditions A `http_version_condition` block as defined below.
* @property modifyRequestHeaderActions A `modify_request_header_action` block as defined below.
* @property modifyResponseHeaderActions A `modify_response_header_action` block as defined below.
* @property name The Name which should be used for this Delivery Rule.
* @property order The order used for this rule. The order values should be sequential and begin at `1`.
* @property postArgConditions A `post_arg_condition` block as defined below.
* @property queryStringConditions A `query_string_condition` block as defined below.
* @property remoteAddressConditions A `remote_address_condition` block as defined below.
* @property requestBodyConditions A `request_body_condition` block as defined below.
* @property requestHeaderConditions A `request_header_condition` block as defined below.
* @property requestMethodCondition A `request_method_condition` block as defined below.
* @property requestSchemeCondition A `request_scheme_condition` block as defined below.
* @property requestUriConditions A `request_uri_condition` block as defined below.
* @property urlFileExtensionConditions A `url_file_extension_condition` block as defined below.
* @property urlFileNameConditions A `url_file_name_condition` block as defined below.
* @property urlPathConditions A `url_path_condition` block as defined below.
* @property urlRedirectAction A `url_redirect_action` block as defined below.
* @property urlRewriteAction A `url_rewrite_action` block as defined below.
*/
public data class EndpointDeliveryRule(
public val cacheExpirationAction: EndpointDeliveryRuleCacheExpirationAction? = null,
public val cacheKeyQueryStringAction: EndpointDeliveryRuleCacheKeyQueryStringAction? = null,
public val cookiesConditions: List? = null,
public val deviceCondition: EndpointDeliveryRuleDeviceCondition? = null,
public val httpVersionConditions: List? = null,
public val modifyRequestHeaderActions: List? =
null,
public val modifyResponseHeaderActions: List? =
null,
public val name: String,
public val order: Int,
public val postArgConditions: List? = null,
public val queryStringConditions: List? = null,
public val remoteAddressConditions: List? = null,
public val requestBodyConditions: List? = null,
public val requestHeaderConditions: List? = null,
public val requestMethodCondition: EndpointDeliveryRuleRequestMethodCondition? = null,
public val requestSchemeCondition: EndpointDeliveryRuleRequestSchemeCondition? = null,
public val requestUriConditions: List? = null,
public val urlFileExtensionConditions: List? =
null,
public val urlFileNameConditions: List? = null,
public val urlPathConditions: List? = null,
public val urlRedirectAction: EndpointDeliveryRuleUrlRedirectAction? = null,
public val urlRewriteAction: EndpointDeliveryRuleUrlRewriteAction? = null,
) {
public companion object {
public fun toKotlin(javaType: com.pulumi.azure.cdn.outputs.EndpointDeliveryRule):
EndpointDeliveryRule = EndpointDeliveryRule(
cacheExpirationAction = javaType.cacheExpirationAction().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleCacheExpirationAction.Companion.toKotlin(args0)
})
}).orElse(null),
cacheKeyQueryStringAction = javaType.cacheKeyQueryStringAction().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleCacheKeyQueryStringAction.Companion.toKotlin(args0)
})
}).orElse(null),
cookiesConditions = javaType.cookiesConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleCookiesCondition.Companion.toKotlin(args0)
})
}),
deviceCondition = javaType.deviceCondition().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleDeviceCondition.Companion.toKotlin(args0)
})
}).orElse(null),
httpVersionConditions = javaType.httpVersionConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleHttpVersionCondition.Companion.toKotlin(args0)
})
}),
modifyRequestHeaderActions = javaType.modifyRequestHeaderActions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleModifyRequestHeaderAction.Companion.toKotlin(args0)
})
}),
modifyResponseHeaderActions = javaType.modifyResponseHeaderActions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleModifyResponseHeaderAction.Companion.toKotlin(args0)
})
}),
name = javaType.name(),
order = javaType.order(),
postArgConditions = javaType.postArgConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRulePostArgCondition.Companion.toKotlin(args0)
})
}),
queryStringConditions = javaType.queryStringConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleQueryStringCondition.Companion.toKotlin(args0)
})
}),
remoteAddressConditions = javaType.remoteAddressConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleRemoteAddressCondition.Companion.toKotlin(args0)
})
}),
requestBodyConditions = javaType.requestBodyConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleRequestBodyCondition.Companion.toKotlin(args0)
})
}),
requestHeaderConditions = javaType.requestHeaderConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleRequestHeaderCondition.Companion.toKotlin(args0)
})
}),
requestMethodCondition = javaType.requestMethodCondition().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleRequestMethodCondition.Companion.toKotlin(args0)
})
}).orElse(null),
requestSchemeCondition = javaType.requestSchemeCondition().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleRequestSchemeCondition.Companion.toKotlin(args0)
})
}).orElse(null),
requestUriConditions = javaType.requestUriConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleRequestUriCondition.Companion.toKotlin(args0)
})
}),
urlFileExtensionConditions = javaType.urlFileExtensionConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleUrlFileExtensionCondition.Companion.toKotlin(args0)
})
}),
urlFileNameConditions = javaType.urlFileNameConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleUrlFileNameCondition.Companion.toKotlin(args0)
})
}),
urlPathConditions = javaType.urlPathConditions().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleUrlPathCondition.Companion.toKotlin(args0)
})
}),
urlRedirectAction = javaType.urlRedirectAction().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleUrlRedirectAction.Companion.toKotlin(args0)
})
}).orElse(null),
urlRewriteAction = javaType.urlRewriteAction().map({ args0 ->
args0.let({ args0 ->
com.pulumi.azure.cdn.kotlin.outputs.EndpointDeliveryRuleUrlRewriteAction.Companion.toKotlin(args0)
})
}).orElse(null),
)
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy