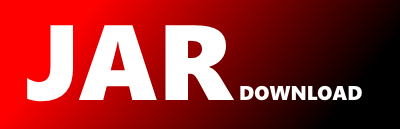
com.pulumi.azure.compute.kotlin.inputs.ImageOsDiskArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.ImageOsDiskArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property blobUri Specifies the URI in Azure storage of the blob that you want to use to create the image. Changing this forces a new resource to be created.
* @property caching Specifies the caching mode as `ReadWrite`, `ReadOnly`, or `None`. The default is `None`.
* @property diskEncryptionSetId The ID of the Disk Encryption Set which should be used to encrypt this image. Changing this forces a new resource to be created.
* @property managedDiskId Specifies the ID of the managed disk resource that you want to use to create the image.
* @property osState Specifies the state of the operating system contained in the blob. Currently, the only value is Generalized. Possible values are `Generalized` and `Specialized`.
* @property osType Specifies the type of operating system contained in the virtual machine image. Possible values are: `Windows` or `Linux`.
* @property sizeGb Specifies the size of the image to be created. Changing this forces a new resource to be created.
*/
public data class ImageOsDiskArgs(
public val blobUri: Output? = null,
public val caching: Output? = null,
public val diskEncryptionSetId: Output? = null,
public val managedDiskId: Output? = null,
public val osState: Output? = null,
public val osType: Output? = null,
public val sizeGb: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.ImageOsDiskArgs =
com.pulumi.azure.compute.inputs.ImageOsDiskArgs.builder()
.blobUri(blobUri?.applyValue({ args0 -> args0 }))
.caching(caching?.applyValue({ args0 -> args0 }))
.diskEncryptionSetId(diskEncryptionSetId?.applyValue({ args0 -> args0 }))
.managedDiskId(managedDiskId?.applyValue({ args0 -> args0 }))
.osState(osState?.applyValue({ args0 -> args0 }))
.osType(osType?.applyValue({ args0 -> args0 }))
.sizeGb(sizeGb?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ImageOsDiskArgs].
*/
@PulumiTagMarker
public class ImageOsDiskArgsBuilder internal constructor() {
private var blobUri: Output? = null
private var caching: Output? = null
private var diskEncryptionSetId: Output? = null
private var managedDiskId: Output? = null
private var osState: Output? = null
private var osType: Output? = null
private var sizeGb: Output? = null
/**
* @param value Specifies the URI in Azure storage of the blob that you want to use to create the image. Changing this forces a new resource to be created.
*/
@JvmName("eyhppajvyjleuqpm")
public suspend fun blobUri(`value`: Output) {
this.blobUri = value
}
/**
* @param value Specifies the caching mode as `ReadWrite`, `ReadOnly`, or `None`. The default is `None`.
*/
@JvmName("hftkseihdrpjqvkg")
public suspend fun caching(`value`: Output) {
this.caching = value
}
/**
* @param value The ID of the Disk Encryption Set which should be used to encrypt this image. Changing this forces a new resource to be created.
*/
@JvmName("bbbpukkkbhhklpml")
public suspend fun diskEncryptionSetId(`value`: Output) {
this.diskEncryptionSetId = value
}
/**
* @param value Specifies the ID of the managed disk resource that you want to use to create the image.
*/
@JvmName("igixuwwvrsaytqpn")
public suspend fun managedDiskId(`value`: Output) {
this.managedDiskId = value
}
/**
* @param value Specifies the state of the operating system contained in the blob. Currently, the only value is Generalized. Possible values are `Generalized` and `Specialized`.
*/
@JvmName("wbvbtdiamckpiyot")
public suspend fun osState(`value`: Output) {
this.osState = value
}
/**
* @param value Specifies the type of operating system contained in the virtual machine image. Possible values are: `Windows` or `Linux`.
*/
@JvmName("tyoquyxyfkelktbu")
public suspend fun osType(`value`: Output) {
this.osType = value
}
/**
* @param value Specifies the size of the image to be created. Changing this forces a new resource to be created.
*/
@JvmName("wenthhdpqnenvmys")
public suspend fun sizeGb(`value`: Output) {
this.sizeGb = value
}
/**
* @param value Specifies the URI in Azure storage of the blob that you want to use to create the image. Changing this forces a new resource to be created.
*/
@JvmName("vrkhnvjcafbigyef")
public suspend fun blobUri(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.blobUri = mapped
}
/**
* @param value Specifies the caching mode as `ReadWrite`, `ReadOnly`, or `None`. The default is `None`.
*/
@JvmName("swgxiqsdwodcsdqi")
public suspend fun caching(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.caching = mapped
}
/**
* @param value The ID of the Disk Encryption Set which should be used to encrypt this image. Changing this forces a new resource to be created.
*/
@JvmName("hljayjkmxunbxuqf")
public suspend fun diskEncryptionSetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskEncryptionSetId = mapped
}
/**
* @param value Specifies the ID of the managed disk resource that you want to use to create the image.
*/
@JvmName("vleoomalaqpfulue")
public suspend fun managedDiskId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.managedDiskId = mapped
}
/**
* @param value Specifies the state of the operating system contained in the blob. Currently, the only value is Generalized. Possible values are `Generalized` and `Specialized`.
*/
@JvmName("kxabsnqeocwxsjsy")
public suspend fun osState(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.osState = mapped
}
/**
* @param value Specifies the type of operating system contained in the virtual machine image. Possible values are: `Windows` or `Linux`.
*/
@JvmName("fokknklwxptkpfee")
public suspend fun osType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.osType = mapped
}
/**
* @param value Specifies the size of the image to be created. Changing this forces a new resource to be created.
*/
@JvmName("sdlxsnlbafnfltlg")
public suspend fun sizeGb(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.sizeGb = mapped
}
internal fun build(): ImageOsDiskArgs = ImageOsDiskArgs(
blobUri = blobUri,
caching = caching,
diskEncryptionSetId = diskEncryptionSetId,
managedDiskId = managedDiskId,
osState = osState,
osType = osType,
sizeGb = sizeGb,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy