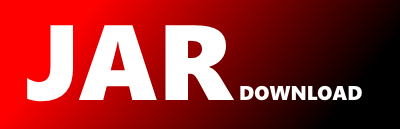
com.pulumi.azure.compute.kotlin.inputs.LinuxVirtualMachineScaleSetOsDiskArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetOsDiskArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.jvm.JvmName
/**
*
* @property caching The Type of Caching which should be used for the Internal OS Disk. Possible values are `None`, `ReadOnly` and `ReadWrite`.
* @property diffDiskSettings A `diff_disk_settings` block as defined above. Changing this forces a new resource to be created.
* @property diskEncryptionSetId The ID of the Disk Encryption Set which should be used to encrypt this OS Disk. Conflicts with `secure_vm_disk_encryption_set_id`. Changing this forces a new resource to be created.
* > **NOTE:** The Disk Encryption Set must have the `Reader` Role Assignment scoped on the Key Vault - in addition to an Access Policy to the Key Vault
* > **NOTE:** Disk Encryption Sets are in Public Preview in a limited set of regions
* @property diskSizeGb The Size of the Internal OS Disk in GB, if you wish to vary from the size used in the image this Virtual Machine Scale Set is sourced from.
* > **NOTE:** If specified this must be equal to or larger than the size of the Image the VM Scale Set is based on. When creating a larger disk than exists in the image you'll need to repartition the disk to use the remaining space.
* @property secureVmDiskEncryptionSetId The ID of the Disk Encryption Set which should be used to Encrypt the OS Disk when the Virtual Machine Scale Set is Confidential VMSS. Conflicts with `disk_encryption_set_id`. Changing this forces a new resource to be created.
* > **NOTE:** `secure_vm_disk_encryption_set_id` can only be specified when `security_encryption_type` is set to `DiskWithVMGuestState`.
* @property securityEncryptionType Encryption Type when the Virtual Machine Scale Set is Confidential VMSS. Possible values are `VMGuestStateOnly` and `DiskWithVMGuestState`. Changing this forces a new resource to be created.
* > **NOTE:** `vtpm_enabled` must be set to `true` when `security_encryption_type` is specified.
* > **NOTE:** `encryption_at_host_enabled` cannot be set to `true` when `security_encryption_type` is set to `DiskWithVMGuestState`.
* @property storageAccountType The Type of Storage Account which should back this the Internal OS Disk. Possible values include `Standard_LRS`, `StandardSSD_LRS`, `StandardSSD_ZRS`, `Premium_LRS` and `Premium_ZRS`. Changing this forces a new resource to be created.
* @property writeAcceleratorEnabled Should Write Accelerator be Enabled for this OS Disk? Defaults to `false`.
* > **NOTE:** This requires that the `storage_account_type` is set to `Premium_LRS` and that `caching` is set to `None`.
*/
public data class LinuxVirtualMachineScaleSetOsDiskArgs(
public val caching: Output,
public val diffDiskSettings: Output? =
null,
public val diskEncryptionSetId: Output? = null,
public val diskSizeGb: Output? = null,
public val secureVmDiskEncryptionSetId: Output? = null,
public val securityEncryptionType: Output? = null,
public val storageAccountType: Output,
public val writeAcceleratorEnabled: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetOsDiskArgs =
com.pulumi.azure.compute.inputs.LinuxVirtualMachineScaleSetOsDiskArgs.builder()
.caching(caching.applyValue({ args0 -> args0 }))
.diffDiskSettings(diffDiskSettings?.applyValue({ args0 -> args0.let({ args0 -> args0.toJava() }) }))
.diskEncryptionSetId(diskEncryptionSetId?.applyValue({ args0 -> args0 }))
.diskSizeGb(diskSizeGb?.applyValue({ args0 -> args0 }))
.secureVmDiskEncryptionSetId(secureVmDiskEncryptionSetId?.applyValue({ args0 -> args0 }))
.securityEncryptionType(securityEncryptionType?.applyValue({ args0 -> args0 }))
.storageAccountType(storageAccountType.applyValue({ args0 -> args0 }))
.writeAcceleratorEnabled(writeAcceleratorEnabled?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [LinuxVirtualMachineScaleSetOsDiskArgs].
*/
@PulumiTagMarker
public class LinuxVirtualMachineScaleSetOsDiskArgsBuilder internal constructor() {
private var caching: Output? = null
private var diffDiskSettings: Output? =
null
private var diskEncryptionSetId: Output? = null
private var diskSizeGb: Output? = null
private var secureVmDiskEncryptionSetId: Output? = null
private var securityEncryptionType: Output? = null
private var storageAccountType: Output? = null
private var writeAcceleratorEnabled: Output? = null
/**
* @param value The Type of Caching which should be used for the Internal OS Disk. Possible values are `None`, `ReadOnly` and `ReadWrite`.
*/
@JvmName("hnqoxxgwfhhhovtn")
public suspend fun caching(`value`: Output) {
this.caching = value
}
/**
* @param value A `diff_disk_settings` block as defined above. Changing this forces a new resource to be created.
*/
@JvmName("riicrmehvugfavlk")
public suspend
fun diffDiskSettings(`value`: Output) {
this.diffDiskSettings = value
}
/**
* @param value The ID of the Disk Encryption Set which should be used to encrypt this OS Disk. Conflicts with `secure_vm_disk_encryption_set_id`. Changing this forces a new resource to be created.
* > **NOTE:** The Disk Encryption Set must have the `Reader` Role Assignment scoped on the Key Vault - in addition to an Access Policy to the Key Vault
* > **NOTE:** Disk Encryption Sets are in Public Preview in a limited set of regions
*/
@JvmName("tkfrxwfksbpjnqvd")
public suspend fun diskEncryptionSetId(`value`: Output) {
this.diskEncryptionSetId = value
}
/**
* @param value The Size of the Internal OS Disk in GB, if you wish to vary from the size used in the image this Virtual Machine Scale Set is sourced from.
* > **NOTE:** If specified this must be equal to or larger than the size of the Image the VM Scale Set is based on. When creating a larger disk than exists in the image you'll need to repartition the disk to use the remaining space.
*/
@JvmName("mywfiwbsbfdnxoqb")
public suspend fun diskSizeGb(`value`: Output) {
this.diskSizeGb = value
}
/**
* @param value The ID of the Disk Encryption Set which should be used to Encrypt the OS Disk when the Virtual Machine Scale Set is Confidential VMSS. Conflicts with `disk_encryption_set_id`. Changing this forces a new resource to be created.
* > **NOTE:** `secure_vm_disk_encryption_set_id` can only be specified when `security_encryption_type` is set to `DiskWithVMGuestState`.
*/
@JvmName("gqdmxqardwqijsah")
public suspend fun secureVmDiskEncryptionSetId(`value`: Output) {
this.secureVmDiskEncryptionSetId = value
}
/**
* @param value Encryption Type when the Virtual Machine Scale Set is Confidential VMSS. Possible values are `VMGuestStateOnly` and `DiskWithVMGuestState`. Changing this forces a new resource to be created.
* > **NOTE:** `vtpm_enabled` must be set to `true` when `security_encryption_type` is specified.
* > **NOTE:** `encryption_at_host_enabled` cannot be set to `true` when `security_encryption_type` is set to `DiskWithVMGuestState`.
*/
@JvmName("sqcprnovgvoqiaet")
public suspend fun securityEncryptionType(`value`: Output) {
this.securityEncryptionType = value
}
/**
* @param value The Type of Storage Account which should back this the Internal OS Disk. Possible values include `Standard_LRS`, `StandardSSD_LRS`, `StandardSSD_ZRS`, `Premium_LRS` and `Premium_ZRS`. Changing this forces a new resource to be created.
*/
@JvmName("npojmxkqaaenyelp")
public suspend fun storageAccountType(`value`: Output) {
this.storageAccountType = value
}
/**
* @param value Should Write Accelerator be Enabled for this OS Disk? Defaults to `false`.
* > **NOTE:** This requires that the `storage_account_type` is set to `Premium_LRS` and that `caching` is set to `None`.
*/
@JvmName("nvrquegmcdoshbpo")
public suspend fun writeAcceleratorEnabled(`value`: Output) {
this.writeAcceleratorEnabled = value
}
/**
* @param value The Type of Caching which should be used for the Internal OS Disk. Possible values are `None`, `ReadOnly` and `ReadWrite`.
*/
@JvmName("ttadomfuptlluign")
public suspend fun caching(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.caching = mapped
}
/**
* @param value A `diff_disk_settings` block as defined above. Changing this forces a new resource to be created.
*/
@JvmName("vqmelvbyufojgldk")
public suspend
fun diffDiskSettings(`value`: LinuxVirtualMachineScaleSetOsDiskDiffDiskSettingsArgs?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diffDiskSettings = mapped
}
/**
* @param argument A `diff_disk_settings` block as defined above. Changing this forces a new resource to be created.
*/
@JvmName("yjvvgyuncomoechh")
public suspend
fun diffDiskSettings(argument: suspend LinuxVirtualMachineScaleSetOsDiskDiffDiskSettingsArgsBuilder.() -> Unit) {
val toBeMapped = LinuxVirtualMachineScaleSetOsDiskDiffDiskSettingsArgsBuilder().applySuspend {
argument()
}.build()
val mapped = of(toBeMapped)
this.diffDiskSettings = mapped
}
/**
* @param value The ID of the Disk Encryption Set which should be used to encrypt this OS Disk. Conflicts with `secure_vm_disk_encryption_set_id`. Changing this forces a new resource to be created.
* > **NOTE:** The Disk Encryption Set must have the `Reader` Role Assignment scoped on the Key Vault - in addition to an Access Policy to the Key Vault
* > **NOTE:** Disk Encryption Sets are in Public Preview in a limited set of regions
*/
@JvmName("gbmitgedlqtqfmet")
public suspend fun diskEncryptionSetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskEncryptionSetId = mapped
}
/**
* @param value The Size of the Internal OS Disk in GB, if you wish to vary from the size used in the image this Virtual Machine Scale Set is sourced from.
* > **NOTE:** If specified this must be equal to or larger than the size of the Image the VM Scale Set is based on. When creating a larger disk than exists in the image you'll need to repartition the disk to use the remaining space.
*/
@JvmName("fhkvmhjnnexvnhjm")
public suspend fun diskSizeGb(`value`: Int?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.diskSizeGb = mapped
}
/**
* @param value The ID of the Disk Encryption Set which should be used to Encrypt the OS Disk when the Virtual Machine Scale Set is Confidential VMSS. Conflicts with `disk_encryption_set_id`. Changing this forces a new resource to be created.
* > **NOTE:** `secure_vm_disk_encryption_set_id` can only be specified when `security_encryption_type` is set to `DiskWithVMGuestState`.
*/
@JvmName("dqjtbvoekujohbyd")
public suspend fun secureVmDiskEncryptionSetId(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.secureVmDiskEncryptionSetId = mapped
}
/**
* @param value Encryption Type when the Virtual Machine Scale Set is Confidential VMSS. Possible values are `VMGuestStateOnly` and `DiskWithVMGuestState`. Changing this forces a new resource to be created.
* > **NOTE:** `vtpm_enabled` must be set to `true` when `security_encryption_type` is specified.
* > **NOTE:** `encryption_at_host_enabled` cannot be set to `true` when `security_encryption_type` is set to `DiskWithVMGuestState`.
*/
@JvmName("wuwojtwawohjgmah")
public suspend fun securityEncryptionType(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.securityEncryptionType = mapped
}
/**
* @param value The Type of Storage Account which should back this the Internal OS Disk. Possible values include `Standard_LRS`, `StandardSSD_LRS`, `StandardSSD_ZRS`, `Premium_LRS` and `Premium_ZRS`. Changing this forces a new resource to be created.
*/
@JvmName("yrepsjytgusaqtss")
public suspend fun storageAccountType(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.storageAccountType = mapped
}
/**
* @param value Should Write Accelerator be Enabled for this OS Disk? Defaults to `false`.
* > **NOTE:** This requires that the `storage_account_type` is set to `Premium_LRS` and that `caching` is set to `None`.
*/
@JvmName("nvkpctrxpifgbkvd")
public suspend fun writeAcceleratorEnabled(`value`: Boolean?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.writeAcceleratorEnabled = mapped
}
internal fun build(): LinuxVirtualMachineScaleSetOsDiskArgs =
LinuxVirtualMachineScaleSetOsDiskArgs(
caching = caching ?: throw PulumiNullFieldException("caching"),
diffDiskSettings = diffDiskSettings,
diskEncryptionSetId = diskEncryptionSetId,
diskSizeGb = diskSizeGb,
secureVmDiskEncryptionSetId = secureVmDiskEncryptionSetId,
securityEncryptionType = securityEncryptionType,
storageAccountType = storageAccountType ?: throw PulumiNullFieldException("storageAccountType"),
writeAcceleratorEnabled = writeAcceleratorEnabled,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy