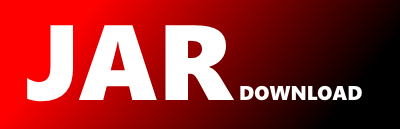
com.pulumi.azure.compute.kotlin.inputs.ScaleSetOsProfileArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.ScaleSetOsProfileArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.jvm.JvmName
/**
*
* @property adminPassword Specifies the administrator password to use for all the instances of virtual machines in a scale set.
* @property adminUsername Specifies the administrator account name to use for all the instances of virtual machines in the scale set.
* @property computerNamePrefix Specifies the computer name prefix for all of the virtual machines in the scale set. Computer name prefixes must be 1 to 9 characters long for windows images and 1 - 58 for Linux. Changing this forces a new resource to be created.
* @property customData Specifies custom data to supply to the machine. On Linux-based systems, this can be used as a cloud-init script. On other systems, this will be copied as a file on disk. Internally, this provider will base64 encode this value before sending it to the API. The maximum length of the binary array is 65535 bytes.
*/
public data class ScaleSetOsProfileArgs(
public val adminPassword: Output? = null,
public val adminUsername: Output,
public val computerNamePrefix: Output,
public val customData: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.ScaleSetOsProfileArgs =
com.pulumi.azure.compute.inputs.ScaleSetOsProfileArgs.builder()
.adminPassword(adminPassword?.applyValue({ args0 -> args0 }))
.adminUsername(adminUsername.applyValue({ args0 -> args0 }))
.computerNamePrefix(computerNamePrefix.applyValue({ args0 -> args0 }))
.customData(customData?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ScaleSetOsProfileArgs].
*/
@PulumiTagMarker
public class ScaleSetOsProfileArgsBuilder internal constructor() {
private var adminPassword: Output? = null
private var adminUsername: Output? = null
private var computerNamePrefix: Output? = null
private var customData: Output? = null
/**
* @param value Specifies the administrator password to use for all the instances of virtual machines in a scale set.
*/
@JvmName("hgxylbgbcdqfouhe")
public suspend fun adminPassword(`value`: Output) {
this.adminPassword = value
}
/**
* @param value Specifies the administrator account name to use for all the instances of virtual machines in the scale set.
*/
@JvmName("dlwqfpmrgwyiocjb")
public suspend fun adminUsername(`value`: Output) {
this.adminUsername = value
}
/**
* @param value Specifies the computer name prefix for all of the virtual machines in the scale set. Computer name prefixes must be 1 to 9 characters long for windows images and 1 - 58 for Linux. Changing this forces a new resource to be created.
*/
@JvmName("vbcxjdavnmofbblp")
public suspend fun computerNamePrefix(`value`: Output) {
this.computerNamePrefix = value
}
/**
* @param value Specifies custom data to supply to the machine. On Linux-based systems, this can be used as a cloud-init script. On other systems, this will be copied as a file on disk. Internally, this provider will base64 encode this value before sending it to the API. The maximum length of the binary array is 65535 bytes.
*/
@JvmName("tyaucxxmdopeqvtn")
public suspend fun customData(`value`: Output) {
this.customData = value
}
/**
* @param value Specifies the administrator password to use for all the instances of virtual machines in a scale set.
*/
@JvmName("xnhuunmdvypcdpqw")
public suspend fun adminPassword(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.adminPassword = mapped
}
/**
* @param value Specifies the administrator account name to use for all the instances of virtual machines in the scale set.
*/
@JvmName("oxhrrosmxgvvtgqn")
public suspend fun adminUsername(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.adminUsername = mapped
}
/**
* @param value Specifies the computer name prefix for all of the virtual machines in the scale set. Computer name prefixes must be 1 to 9 characters long for windows images and 1 - 58 for Linux. Changing this forces a new resource to be created.
*/
@JvmName("vofdcwlvnunvycje")
public suspend fun computerNamePrefix(`value`: String) {
val toBeMapped = value
val mapped = toBeMapped.let({ args0 -> of(args0) })
this.computerNamePrefix = mapped
}
/**
* @param value Specifies custom data to supply to the machine. On Linux-based systems, this can be used as a cloud-init script. On other systems, this will be copied as a file on disk. Internally, this provider will base64 encode this value before sending it to the API. The maximum length of the binary array is 65535 bytes.
*/
@JvmName("udkruolgyskrtnmf")
public suspend fun customData(`value`: String?) {
val toBeMapped = value
val mapped = toBeMapped?.let({ args0 -> of(args0) })
this.customData = mapped
}
internal fun build(): ScaleSetOsProfileArgs = ScaleSetOsProfileArgs(
adminPassword = adminPassword,
adminUsername = adminUsername ?: throw PulumiNullFieldException("adminUsername"),
computerNamePrefix = computerNamePrefix ?: throw PulumiNullFieldException("computerNamePrefix"),
customData = customData,
)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy