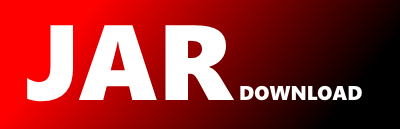
com.pulumi.azure.compute.kotlin.inputs.ScaleSetStorageProfileOsDiskArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.compute.kotlin.inputs
import com.pulumi.azure.compute.inputs.ScaleSetStorageProfileOsDiskArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property caching Specifies the caching requirements. Possible values include: `None` (default), `ReadOnly`, `ReadWrite`.
* @property createOption Specifies how the virtual machine should be created. The only possible option is `FromImage`.
* @property image Specifies the blob URI for user image. A virtual machine scale set creates an os disk in the same container as the user image.
* Updating the osDisk image causes the existing disk to be deleted and a new one created with the new image. If the VM scale set is in Manual upgrade mode then the virtual machines are not updated until they have manualUpgrade applied to them.
* When setting this field `os_type` needs to be specified. Cannot be used when `vhd_containers`, `managed_disk_type` or `storage_profile_image_reference` are specified.
* @property managedDiskType Specifies the type of managed disk to create. Value you must be either `Standard_LRS`, `StandardSSD_LRS` or `Premium_LRS`. Cannot be used when `vhd_containers` or `image` is specified.
* @property name Specifies the disk name. Must be specified when using unmanaged disk ('managed_disk_type' property not set).
* @property osType Specifies the operating system Type, valid values are windows, Linux.
* @property vhdContainers Specifies the VHD URI. Cannot be used when `image` or `managed_disk_type` is specified.
*/
public data class ScaleSetStorageProfileOsDiskArgs(
public val caching: Output? = null,
public val createOption: Output,
public val image: Output? = null,
public val managedDiskType: Output? = null,
public val name: Output? = null,
public val osType: Output? = null,
public val vhdContainers: Output>? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.compute.inputs.ScaleSetStorageProfileOsDiskArgs =
com.pulumi.azure.compute.inputs.ScaleSetStorageProfileOsDiskArgs.builder()
.caching(caching?.applyValue({ args0 -> args0 }))
.createOption(createOption.applyValue({ args0 -> args0 }))
.image(image?.applyValue({ args0 -> args0 }))
.managedDiskType(managedDiskType?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.osType(osType?.applyValue({ args0 -> args0 }))
.vhdContainers(vhdContainers?.applyValue({ args0 -> args0.map({ args0 -> args0 }) })).build()
}
/**
* Builder for [ScaleSetStorageProfileOsDiskArgs].
*/
@PulumiTagMarker
public class ScaleSetStorageProfileOsDiskArgsBuilder internal constructor() {
private var caching: Output? = null
private var createOption: Output? = null
private var image: Output? = null
private var managedDiskType: Output? = null
private var name: Output? = null
private var osType: Output? = null
private var vhdContainers: Output>? = null
/**
* @param value Specifies the caching requirements. Possible values include: `None` (default), `ReadOnly`, `ReadWrite`.
*/
@JvmName("fuxysvvnmevkvbhi")
public suspend fun caching(`value`: Output) {
this.caching = value
}
/**
* @param value Specifies how the virtual machine should be created. The only possible option is `FromImage`.
*/
@JvmName("dehcurhlfotvcaro")
public suspend fun createOption(`value`: Output) {
this.createOption = value
}
/**
* @param value Specifies the blob URI for user image. A virtual machine scale set creates an os disk in the same container as the user image.
* Updating the osDisk image causes the existing disk to be deleted and a new one created with the new image. If the VM scale set is in Manual upgrade mode then the virtual machines are not updated until they have manualUpgrade applied to them.
* When setting this field `os_type` needs to be specified. Cannot be used when `vhd_containers`, `managed_disk_type` or `storage_profile_image_reference` are specified.
*/
@JvmName("evsbnpvxacqsnorm")
public suspend fun image(`value`: Output) {
this.image = value
}
/**
* @param value Specifies the type of managed disk to create. Value you must be either `Standard_LRS`, `StandardSSD_LRS` or `Premium_LRS`. Cannot be used when `vhd_containers` or `image` is specified.
*/
@JvmName("qikrcjaqeaecbvec")
public suspend fun managedDiskType(`value`: Output) {
this.managedDiskType = value
}
/**
* @param value Specifies the disk name. Must be specified when using unmanaged disk ('managed_disk_type' property not set).
*/
@JvmName("rnnayvbppcstygyj")
public suspend fun name(`value`: Output) {
this.name = value
}
/**
* @param value Specifies the operating system Type, valid values are windows, Linux.
*/
@JvmName("afrfipccmikesklp")
public suspend fun osType(`value`: Output) {
this.osType = value
}
/**
* @param value Specifies the VHD URI. Cannot be used when `image` or `managed_disk_type` is specified.
*/
@JvmName("desqwcyqgxmrdyfm")
public suspend fun vhdContainers(`value`: Output>) {
this.vhdContainers = value
}
@JvmName("plawfayablnwvdbp")
public suspend fun vhdContainers(vararg values: Output) {
this.vhdContainers = Output.all(values.asList())
}
/**
* @param values Specifies the VHD URI. Cannot be used when `image` or `managed_disk_type` is specified.
*/
@JvmName("vtqawyquehjgmnny")
public suspend fun vhdContainers(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy