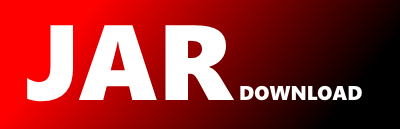
com.pulumi.azure.consumption.kotlin.inputs.BudgetSubscriptionNotificationArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.consumption.kotlin.inputs
import com.pulumi.azure.consumption.inputs.BudgetSubscriptionNotificationArgs.builder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiNullFieldException
import com.pulumi.kotlin.PulumiTagMarker
import kotlin.Boolean
import kotlin.Int
import kotlin.String
import kotlin.Suppress
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
*
* @property contactEmails Specifies a list of email addresses to send the budget notification to when the threshold is exceeded.
* @property contactGroups Specifies a list of Action Group IDs to send the budget notification to when the threshold is exceeded.
* @property contactRoles Specifies a list of contact roles to send the budget notification to when the threshold is exceeded.
* @property enabled Should the notification be enabled? Defaults to `true`.
* > **NOTE:** A `notification` block cannot have all of `contact_emails`, `contact_roles`, and `contact_groups` empty. This means that at least one of the three must be specified.
* @property operator The comparison operator for the notification. Must be one of `EqualTo`, `GreaterThan`, or `GreaterThanOrEqualTo`.
* @property threshold Threshold value associated with a notification. Notification is sent when the cost exceeded the threshold. It is always percent and has to be between 0 and 1000.
* @property thresholdType The type of threshold for the notification. This determines whether the notification is triggered by forecasted costs or actual costs. The allowed values are `Actual` and `Forecasted`. Default is `Actual`. Changing this forces a new resource to be created.
*/
public data class BudgetSubscriptionNotificationArgs(
public val contactEmails: Output>? = null,
public val contactGroups: Output>? = null,
public val contactRoles: Output>? = null,
public val enabled: Output? = null,
public val `operator`: Output,
public val threshold: Output,
public val thresholdType: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.consumption.inputs.BudgetSubscriptionNotificationArgs =
com.pulumi.azure.consumption.inputs.BudgetSubscriptionNotificationArgs.builder()
.contactEmails(contactEmails?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.contactGroups(contactGroups?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.contactRoles(contactRoles?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.enabled(enabled?.applyValue({ args0 -> args0 }))
.`operator`(`operator`.applyValue({ args0 -> args0 }))
.threshold(threshold.applyValue({ args0 -> args0 }))
.thresholdType(thresholdType?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [BudgetSubscriptionNotificationArgs].
*/
@PulumiTagMarker
public class BudgetSubscriptionNotificationArgsBuilder internal constructor() {
private var contactEmails: Output>? = null
private var contactGroups: Output>? = null
private var contactRoles: Output>? = null
private var enabled: Output? = null
private var `operator`: Output? = null
private var threshold: Output? = null
private var thresholdType: Output? = null
/**
* @param value Specifies a list of email addresses to send the budget notification to when the threshold is exceeded.
*/
@JvmName("hopoblkflgggsbad")
public suspend fun contactEmails(`value`: Output>) {
this.contactEmails = value
}
@JvmName("ubogcennrnfankqc")
public suspend fun contactEmails(vararg values: Output) {
this.contactEmails = Output.all(values.asList())
}
/**
* @param values Specifies a list of email addresses to send the budget notification to when the threshold is exceeded.
*/
@JvmName("ppmktpmnlvsyxngn")
public suspend fun contactEmails(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy