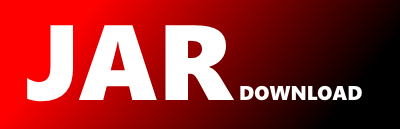
com.pulumi.azure.containerservice.kotlin.ConnectedRegistryArgs.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.ConnectedRegistryArgs.builder
import com.pulumi.azure.containerservice.kotlin.inputs.ConnectedRegistryNotificationArgs
import com.pulumi.azure.containerservice.kotlin.inputs.ConnectedRegistryNotificationArgsBuilder
import com.pulumi.core.Output
import com.pulumi.core.Output.of
import com.pulumi.kotlin.ConvertibleToJava
import com.pulumi.kotlin.PulumiTagMarker
import com.pulumi.kotlin.applySuspend
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import kotlin.jvm.JvmName
/**
* Manages a Container Connected Registry.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = new azure.core.ResourceGroup("example", {
* name: "example-rg",
* location: "West Europe",
* });
* const exampleRegistry = new azure.containerservice.Registry("example", {
* name: "exampleacr",
* resourceGroupName: example.name,
* location: example.location,
* sku: "Premium",
* dataEndpointEnabled: true,
* });
* const exampleRegistryScopeMap = new azure.containerservice.RegistryScopeMap("example", {
* name: "examplescopemap",
* containerRegistryName: exampleRegistry.name,
* resourceGroupName: exampleRegistry.resourceGroupName,
* actions: [
* "repositories/hello-world/content/delete",
* "repositories/hello-world/content/read",
* "repositories/hello-world/content/write",
* "repositories/hello-world/metadata/read",
* "repositories/hello-world/metadata/write",
* "gateway/examplecr/config/read",
* "gateway/examplecr/config/write",
* "gateway/examplecr/message/read",
* "gateway/examplecr/message/write",
* ],
* });
* const exampleRegistryToken = new azure.containerservice.RegistryToken("example", {
* name: "exampletoken",
* containerRegistryName: exampleRegistry.name,
* resourceGroupName: exampleRegistry.resourceGroupName,
* scopeMapId: exampleRegistryScopeMap.id,
* });
* const exampleConnectedRegistry = new azure.containerservice.ConnectedRegistry("example", {
* name: "examplecr",
* containerRegistryId: exampleRegistry.id,
* syncTokenId: exampleRegistryToken.id,
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.core.ResourceGroup("example",
* name="example-rg",
* location="West Europe")
* example_registry = azure.containerservice.Registry("example",
* name="exampleacr",
* resource_group_name=example.name,
* location=example.location,
* sku="Premium",
* data_endpoint_enabled=True)
* example_registry_scope_map = azure.containerservice.RegistryScopeMap("example",
* name="examplescopemap",
* container_registry_name=example_registry.name,
* resource_group_name=example_registry.resource_group_name,
* actions=[
* "repositories/hello-world/content/delete",
* "repositories/hello-world/content/read",
* "repositories/hello-world/content/write",
* "repositories/hello-world/metadata/read",
* "repositories/hello-world/metadata/write",
* "gateway/examplecr/config/read",
* "gateway/examplecr/config/write",
* "gateway/examplecr/message/read",
* "gateway/examplecr/message/write",
* ])
* example_registry_token = azure.containerservice.RegistryToken("example",
* name="exampletoken",
* container_registry_name=example_registry.name,
* resource_group_name=example_registry.resource_group_name,
* scope_map_id=example_registry_scope_map.id)
* example_connected_registry = azure.containerservice.ConnectedRegistry("example",
* name="examplecr",
* container_registry_id=example_registry.id,
* sync_token_id=example_registry_token.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = new Azure.Core.ResourceGroup("example", new()
* {
* Name = "example-rg",
* Location = "West Europe",
* });
* var exampleRegistry = new Azure.ContainerService.Registry("example", new()
* {
* Name = "exampleacr",
* ResourceGroupName = example.Name,
* Location = example.Location,
* Sku = "Premium",
* DataEndpointEnabled = true,
* });
* var exampleRegistryScopeMap = new Azure.ContainerService.RegistryScopeMap("example", new()
* {
* Name = "examplescopemap",
* ContainerRegistryName = exampleRegistry.Name,
* ResourceGroupName = exampleRegistry.ResourceGroupName,
* Actions = new[]
* {
* "repositories/hello-world/content/delete",
* "repositories/hello-world/content/read",
* "repositories/hello-world/content/write",
* "repositories/hello-world/metadata/read",
* "repositories/hello-world/metadata/write",
* "gateway/examplecr/config/read",
* "gateway/examplecr/config/write",
* "gateway/examplecr/message/read",
* "gateway/examplecr/message/write",
* },
* });
* var exampleRegistryToken = new Azure.ContainerService.RegistryToken("example", new()
* {
* Name = "exampletoken",
* ContainerRegistryName = exampleRegistry.Name,
* ResourceGroupName = exampleRegistry.ResourceGroupName,
* ScopeMapId = exampleRegistryScopeMap.Id,
* });
* var exampleConnectedRegistry = new Azure.ContainerService.ConnectedRegistry("example", new()
* {
* Name = "examplecr",
* ContainerRegistryId = exampleRegistry.Id,
* SyncTokenId = exampleRegistryToken.Id,
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/core"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := core.NewResourceGroup(ctx, "example", &core.ResourceGroupArgs{
* Name: pulumi.String("example-rg"),
* Location: pulumi.String("West Europe"),
* })
* if err != nil {
* return err
* }
* exampleRegistry, err := containerservice.NewRegistry(ctx, "example", &containerservice.RegistryArgs{
* Name: pulumi.String("exampleacr"),
* ResourceGroupName: example.Name,
* Location: example.Location,
* Sku: pulumi.String("Premium"),
* DataEndpointEnabled: pulumi.Bool(true),
* })
* if err != nil {
* return err
* }
* exampleRegistryScopeMap, err := containerservice.NewRegistryScopeMap(ctx, "example", &containerservice.RegistryScopeMapArgs{
* Name: pulumi.String("examplescopemap"),
* ContainerRegistryName: exampleRegistry.Name,
* ResourceGroupName: exampleRegistry.ResourceGroupName,
* Actions: pulumi.StringArray{
* pulumi.String("repositories/hello-world/content/delete"),
* pulumi.String("repositories/hello-world/content/read"),
* pulumi.String("repositories/hello-world/content/write"),
* pulumi.String("repositories/hello-world/metadata/read"),
* pulumi.String("repositories/hello-world/metadata/write"),
* pulumi.String("gateway/examplecr/config/read"),
* pulumi.String("gateway/examplecr/config/write"),
* pulumi.String("gateway/examplecr/message/read"),
* pulumi.String("gateway/examplecr/message/write"),
* },
* })
* if err != nil {
* return err
* }
* exampleRegistryToken, err := containerservice.NewRegistryToken(ctx, "example", &containerservice.RegistryTokenArgs{
* Name: pulumi.String("exampletoken"),
* ContainerRegistryName: exampleRegistry.Name,
* ResourceGroupName: exampleRegistry.ResourceGroupName,
* ScopeMapId: exampleRegistryScopeMap.ID(),
* })
* if err != nil {
* return err
* }
* _, err = containerservice.NewConnectedRegistry(ctx, "example", &containerservice.ConnectedRegistryArgs{
* Name: pulumi.String("examplecr"),
* ContainerRegistryId: exampleRegistry.ID(),
* SyncTokenId: exampleRegistryToken.ID(),
* })
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.core.ResourceGroup;
* import com.pulumi.azure.core.ResourceGroupArgs;
* import com.pulumi.azure.containerservice.Registry;
* import com.pulumi.azure.containerservice.RegistryArgs;
* import com.pulumi.azure.containerservice.RegistryScopeMap;
* import com.pulumi.azure.containerservice.RegistryScopeMapArgs;
* import com.pulumi.azure.containerservice.RegistryToken;
* import com.pulumi.azure.containerservice.RegistryTokenArgs;
* import com.pulumi.azure.containerservice.ConnectedRegistry;
* import com.pulumi.azure.containerservice.ConnectedRegistryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* var example = new ResourceGroup("example", ResourceGroupArgs.builder()
* .name("example-rg")
* .location("West Europe")
* .build());
* var exampleRegistry = new Registry("exampleRegistry", RegistryArgs.builder()
* .name("exampleacr")
* .resourceGroupName(example.name())
* .location(example.location())
* .sku("Premium")
* .dataEndpointEnabled(true)
* .build());
* var exampleRegistryScopeMap = new RegistryScopeMap("exampleRegistryScopeMap", RegistryScopeMapArgs.builder()
* .name("examplescopemap")
* .containerRegistryName(exampleRegistry.name())
* .resourceGroupName(exampleRegistry.resourceGroupName())
* .actions(
* "repositories/hello-world/content/delete",
* "repositories/hello-world/content/read",
* "repositories/hello-world/content/write",
* "repositories/hello-world/metadata/read",
* "repositories/hello-world/metadata/write",
* "gateway/examplecr/config/read",
* "gateway/examplecr/config/write",
* "gateway/examplecr/message/read",
* "gateway/examplecr/message/write")
* .build());
* var exampleRegistryToken = new RegistryToken("exampleRegistryToken", RegistryTokenArgs.builder()
* .name("exampletoken")
* .containerRegistryName(exampleRegistry.name())
* .resourceGroupName(exampleRegistry.resourceGroupName())
* .scopeMapId(exampleRegistryScopeMap.id())
* .build());
* var exampleConnectedRegistry = new ConnectedRegistry("exampleConnectedRegistry", ConnectedRegistryArgs.builder()
* .name("examplecr")
* .containerRegistryId(exampleRegistry.id())
* .syncTokenId(exampleRegistryToken.id())
* .build());
* }
* }
* ```
* ```yaml
* resources:
* example:
* type: azure:core:ResourceGroup
* properties:
* name: example-rg
* location: West Europe
* exampleRegistry:
* type: azure:containerservice:Registry
* name: example
* properties:
* name: exampleacr
* resourceGroupName: ${example.name}
* location: ${example.location}
* sku: Premium
* dataEndpointEnabled: true
* exampleRegistryScopeMap:
* type: azure:containerservice:RegistryScopeMap
* name: example
* properties:
* name: examplescopemap
* containerRegistryName: ${exampleRegistry.name}
* resourceGroupName: ${exampleRegistry.resourceGroupName}
* actions:
* - repositories/hello-world/content/delete
* - repositories/hello-world/content/read
* - repositories/hello-world/content/write
* - repositories/hello-world/metadata/read
* - repositories/hello-world/metadata/write
* - gateway/examplecr/config/read
* - gateway/examplecr/config/write
* - gateway/examplecr/message/read
* - gateway/examplecr/message/write
* exampleRegistryToken:
* type: azure:containerservice:RegistryToken
* name: example
* properties:
* name: exampletoken
* containerRegistryName: ${exampleRegistry.name}
* resourceGroupName: ${exampleRegistry.resourceGroupName}
* scopeMapId: ${exampleRegistryScopeMap.id}
* exampleConnectedRegistry:
* type: azure:containerservice:ConnectedRegistry
* name: example
* properties:
* name: examplecr
* containerRegistryId: ${exampleRegistry.id}
* syncTokenId: ${exampleRegistryToken.id}
* ```
*
* ## Import
* Container Connected Registries can be imported using the `resource id`, e.g.
* ```sh
* $ pulumi import azure:containerservice/connectedRegistry:ConnectedRegistry example /subscriptions/12345678-1234-9876-4563-123456789012/resourceGroups/group1/providers/Microsoft.ContainerRegistry/registries/registry1/connectedRegistries/registry1
* ```
* @property auditLogEnabled Should the log auditing be enabled?
* @property clientTokenIds Specifies a list of IDs of Container Registry Tokens, which are meant to be used by the clients to connect to the Connected Registry.
* @property containerRegistryId The ID of the Container Registry that this Connected Registry will reside in. Changing this forces a new Container Connected Registry to be created.
* > If `parent_registry_id` is not specified, the Connected Registry will be connected to the Container Registry identified by `container_registry_id`.
* @property logLevel The verbosity of the logs. Possible values are `None`, `Debug`, `Information`, `Warning` and `Error`. Defaults to `None`.
* @property mode The mode of the Connected Registry. Possible values are `Mirror`, `ReadOnly`, `ReadWrite` and `Registry`. Changing this forces a new Container Connected Registry to be created. Defaults to `ReadWrite`.
* @property name The name which should be used for this Container Connected Registry. Changing this forces a new Container Connected Registry to be created.
* @property notifications One or more `notification` blocks as defined below.
* @property parentRegistryId The ID of the parent registry. This can be either a Container Registry ID or a Connected Registry ID. Changing this forces a new Container Connected Registry to be created.
* @property syncMessageTtl The period of time (in form of ISO8601) for which a message is available to sync before it is expired. Allowed range is from `P1D` to `P90D`. Defaults to `P1D`.
* @property syncSchedule The cron expression indicating the schedule that the Connected Registry will sync with its parent. Defaults to `* * * * *`.
* @property syncTokenId The ID of the Container Registry Token which is used for synchronizing the Connected Registry. Changing this forces a new Container Connected Registry to be created.
* @property syncWindow The time window (in form of ISO8601) during which sync is enabled for each schedule occurrence. Allowed range is from `PT3H` to `P7D`.
*/
public data class ConnectedRegistryArgs(
public val auditLogEnabled: Output? = null,
public val clientTokenIds: Output>? = null,
public val containerRegistryId: Output? = null,
public val logLevel: Output? = null,
public val mode: Output? = null,
public val name: Output? = null,
public val notifications: Output>? = null,
public val parentRegistryId: Output? = null,
public val syncMessageTtl: Output? = null,
public val syncSchedule: Output? = null,
public val syncTokenId: Output? = null,
public val syncWindow: Output? = null,
) : ConvertibleToJava {
override fun toJava(): com.pulumi.azure.containerservice.ConnectedRegistryArgs =
com.pulumi.azure.containerservice.ConnectedRegistryArgs.builder()
.auditLogEnabled(auditLogEnabled?.applyValue({ args0 -> args0 }))
.clientTokenIds(clientTokenIds?.applyValue({ args0 -> args0.map({ args0 -> args0 }) }))
.containerRegistryId(containerRegistryId?.applyValue({ args0 -> args0 }))
.logLevel(logLevel?.applyValue({ args0 -> args0 }))
.mode(mode?.applyValue({ args0 -> args0 }))
.name(name?.applyValue({ args0 -> args0 }))
.notifications(
notifications?.applyValue({ args0 ->
args0.map({ args0 ->
args0.let({ args0 ->
args0.toJava()
})
})
}),
)
.parentRegistryId(parentRegistryId?.applyValue({ args0 -> args0 }))
.syncMessageTtl(syncMessageTtl?.applyValue({ args0 -> args0 }))
.syncSchedule(syncSchedule?.applyValue({ args0 -> args0 }))
.syncTokenId(syncTokenId?.applyValue({ args0 -> args0 }))
.syncWindow(syncWindow?.applyValue({ args0 -> args0 })).build()
}
/**
* Builder for [ConnectedRegistryArgs].
*/
@PulumiTagMarker
public class ConnectedRegistryArgsBuilder internal constructor() {
private var auditLogEnabled: Output? = null
private var clientTokenIds: Output>? = null
private var containerRegistryId: Output? = null
private var logLevel: Output? = null
private var mode: Output? = null
private var name: Output? = null
private var notifications: Output>? = null
private var parentRegistryId: Output? = null
private var syncMessageTtl: Output? = null
private var syncSchedule: Output? = null
private var syncTokenId: Output? = null
private var syncWindow: Output? = null
/**
* @param value Should the log auditing be enabled?
*/
@JvmName("fxixyxycvbjpfnbe")
public suspend fun auditLogEnabled(`value`: Output) {
this.auditLogEnabled = value
}
/**
* @param value Specifies a list of IDs of Container Registry Tokens, which are meant to be used by the clients to connect to the Connected Registry.
*/
@JvmName("dtrrgalcjnfhhduw")
public suspend fun clientTokenIds(`value`: Output>) {
this.clientTokenIds = value
}
@JvmName("hfpehtojyyqhxdpw")
public suspend fun clientTokenIds(vararg values: Output) {
this.clientTokenIds = Output.all(values.asList())
}
/**
* @param values Specifies a list of IDs of Container Registry Tokens, which are meant to be used by the clients to connect to the Connected Registry.
*/
@JvmName("ltycyfsyarxqdtyo")
public suspend fun clientTokenIds(values: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy