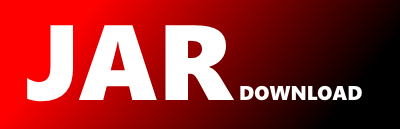
com.pulumi.azure.containerservice.kotlin.ContainerserviceFunctions.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pulumi-azure-kotlin Show documentation
Show all versions of pulumi-azure-kotlin Show documentation
Build cloud applications and infrastructure by combining the safety and reliability of infrastructure as code with the power of the Kotlin programming language.
@file:Suppress("NAME_SHADOWING", "DEPRECATION")
package com.pulumi.azure.containerservice.kotlin
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getClusterNodePoolPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getGroupPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getKubernetesClusterPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getKubernetesNodePoolSnapshotPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getKubernetesServiceVersionsPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getRegistryPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getRegistryScopeMapPlain
import com.pulumi.azure.containerservice.ContainerserviceFunctions.getRegistryTokenPlain
import com.pulumi.azure.containerservice.kotlin.inputs.GetClusterNodePoolPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetClusterNodePoolPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetGroupPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetGroupPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesClusterPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesClusterPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesNodePoolSnapshotPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesNodePoolSnapshotPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesServiceVersionsPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesServiceVersionsPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryScopeMapPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryScopeMapPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryTokenPlainArgs
import com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryTokenPlainArgsBuilder
import com.pulumi.azure.containerservice.kotlin.outputs.GetClusterNodePoolResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetGroupResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesNodePoolSnapshotResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesServiceVersionsResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetRegistryResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetRegistryScopeMapResult
import com.pulumi.azure.containerservice.kotlin.outputs.GetRegistryTokenResult
import kotlinx.coroutines.future.await
import kotlin.Boolean
import kotlin.String
import kotlin.Suppress
import kotlin.Unit
import kotlin.collections.List
import com.pulumi.azure.containerservice.kotlin.outputs.GetClusterNodePoolResult.Companion.toKotlin as getClusterNodePoolResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetGroupResult.Companion.toKotlin as getGroupResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesClusterResult.Companion.toKotlin as getKubernetesClusterResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesNodePoolSnapshotResult.Companion.toKotlin as getKubernetesNodePoolSnapshotResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetKubernetesServiceVersionsResult.Companion.toKotlin as getKubernetesServiceVersionsResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetRegistryResult.Companion.toKotlin as getRegistryResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetRegistryScopeMapResult.Companion.toKotlin as getRegistryScopeMapResultToKotlin
import com.pulumi.azure.containerservice.kotlin.outputs.GetRegistryTokenResult.Companion.toKotlin as getRegistryTokenResultToKotlin
public object ContainerserviceFunctions {
/**
* Use this data source to access information about an existing Kubernetes Cluster Node Pool.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getClusterNodePool({
* name: "existing",
* kubernetesClusterName: "existing-cluster",
* resourceGroupName: "existing-resource-group",
* });
* export const id = example.then(example => example.id);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_cluster_node_pool(name="existing",
* kubernetes_cluster_name="existing-cluster",
* resource_group_name="existing-resource-group")
* pulumi.export("id", example.id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetClusterNodePool.Invoke(new()
* {
* Name = "existing",
* KubernetesClusterName = "existing-cluster",
* ResourceGroupName = "existing-resource-group",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getClusterNodePoolResult => getClusterNodePoolResult.Id),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := containerservice.GetClusterNodePool(ctx, &containerservice.GetClusterNodePoolArgs{
* Name: "existing",
* KubernetesClusterName: "existing-cluster",
* ResourceGroupName: "existing-resource-group",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetClusterNodePoolArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getClusterNodePool(GetClusterNodePoolArgs.builder()
* .name("existing")
* .kubernetesClusterName("existing-cluster")
* .resourceGroupName("existing-resource-group")
* .build());
* ctx.export("id", example.applyValue(getClusterNodePoolResult -> getClusterNodePoolResult.id()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getClusterNodePool
* Arguments:
* name: existing
* kubernetesClusterName: existing-cluster
* resourceGroupName: existing-resource-group
* outputs:
* id: ${example.id}
* ```
*
* @param argument A collection of arguments for invoking getClusterNodePool.
* @return A collection of values returned by getClusterNodePool.
*/
public suspend fun getClusterNodePool(argument: GetClusterNodePoolPlainArgs):
GetClusterNodePoolResult =
getClusterNodePoolResultToKotlin(getClusterNodePoolPlain(argument.toJava()).await())
/**
* @see [getClusterNodePool].
* @param kubernetesClusterName The Name of the Kubernetes Cluster where this Node Pool is located.
* @param name The name of this Kubernetes Cluster Node Pool.
* @param resourceGroupName The name of the Resource Group where the Kubernetes Cluster exists.
* @return A collection of values returned by getClusterNodePool.
*/
public suspend fun getClusterNodePool(
kubernetesClusterName: String,
name: String,
resourceGroupName: String,
): GetClusterNodePoolResult {
val argument = GetClusterNodePoolPlainArgs(
kubernetesClusterName = kubernetesClusterName,
name = name,
resourceGroupName = resourceGroupName,
)
return getClusterNodePoolResultToKotlin(getClusterNodePoolPlain(argument.toJava()).await())
}
/**
* @see [getClusterNodePool].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetClusterNodePoolPlainArgs].
* @return A collection of values returned by getClusterNodePool.
*/
public suspend
fun getClusterNodePool(argument: suspend GetClusterNodePoolPlainArgsBuilder.() -> Unit):
GetClusterNodePoolResult {
val builder = GetClusterNodePoolPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getClusterNodePoolResultToKotlin(getClusterNodePoolPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Container Group instance.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getGroup({
* name: "existing",
* resourceGroupName: "existing",
* });
* export const id = example.then(example => example.id);
* export const ipAddress = example.then(example => example.ipAddress);
* export const fqdn = example.then(example => example.fqdn);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_group(name="existing",
* resource_group_name="existing")
* pulumi.export("id", example.id)
* pulumi.export("ipAddress", example.ip_address)
* pulumi.export("fqdn", example.fqdn)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetGroup.Invoke(new()
* {
* Name = "existing",
* ResourceGroupName = "existing",
* });
* return new Dictionary
* {
* ["id"] = example.Apply(getGroupResult => getGroupResult.Id),
* ["ipAddress"] = example.Apply(getGroupResult => getGroupResult.IpAddress),
* ["fqdn"] = example.Apply(getGroupResult => getGroupResult.Fqdn),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := containerservice.LookupGroup(ctx, &containerservice.LookupGroupArgs{
* Name: "existing",
* ResourceGroupName: "existing",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("id", example.Id)
* ctx.Export("ipAddress", example.IpAddress)
* ctx.Export("fqdn", example.Fqdn)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetGroupArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getGroup(GetGroupArgs.builder()
* .name("existing")
* .resourceGroupName("existing")
* .build());
* ctx.export("id", example.applyValue(getGroupResult -> getGroupResult.id()));
* ctx.export("ipAddress", example.applyValue(getGroupResult -> getGroupResult.ipAddress()));
* ctx.export("fqdn", example.applyValue(getGroupResult -> getGroupResult.fqdn()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getGroup
* Arguments:
* name: existing
* resourceGroupName: existing
* outputs:
* id: ${example.id}
* ipAddress: ${example.ipAddress}
* fqdn: ${example.fqdn}
* ```
*
* @param argument A collection of arguments for invoking getGroup.
* @return A collection of values returned by getGroup.
*/
public suspend fun getGroup(argument: GetGroupPlainArgs): GetGroupResult =
getGroupResultToKotlin(getGroupPlain(argument.toJava()).await())
/**
* @see [getGroup].
* @param name The name of this Container Group instance.
* @param resourceGroupName The name of the Resource Group where the Container Group instance exists.
* @param zones A list of Availability Zones in which this Container Group is located.
* @return A collection of values returned by getGroup.
*/
public suspend fun getGroup(
name: String,
resourceGroupName: String,
zones: List? = null,
): GetGroupResult {
val argument = GetGroupPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
zones = zones,
)
return getGroupResultToKotlin(getGroupPlain(argument.toJava()).await())
}
/**
* @see [getGroup].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetGroupPlainArgs].
* @return A collection of values returned by getGroup.
*/
public suspend fun getGroup(argument: suspend GetGroupPlainArgsBuilder.() -> Unit):
GetGroupResult {
val builder = GetGroupPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getGroupResultToKotlin(getGroupPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Managed Kubernetes Cluster (AKS).
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getKubernetesCluster({
* name: "myakscluster",
* resourceGroupName: "my-example-resource-group",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_kubernetes_cluster(name="myakscluster",
* resource_group_name="my-example-resource-group")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetKubernetesCluster.Invoke(new()
* {
* Name = "myakscluster",
* ResourceGroupName = "my-example-resource-group",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerservice.LookupKubernetesCluster(ctx, &containerservice.LookupKubernetesClusterArgs{
* Name: "myakscluster",
* ResourceGroupName: "my-example-resource-group",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetKubernetesClusterArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getKubernetesCluster(GetKubernetesClusterArgs.builder()
* .name("myakscluster")
* .resourceGroupName("my-example-resource-group")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getKubernetesCluster
* Arguments:
* name: myakscluster
* resourceGroupName: my-example-resource-group
* ```
*
* @param argument A collection of arguments for invoking getKubernetesCluster.
* @return A collection of values returned by getKubernetesCluster.
*/
public suspend fun getKubernetesCluster(argument: GetKubernetesClusterPlainArgs):
GetKubernetesClusterResult =
getKubernetesClusterResultToKotlin(getKubernetesClusterPlain(argument.toJava()).await())
/**
* @see [getKubernetesCluster].
* @param name The name of the managed Kubernetes Cluster.
* @param resourceGroupName The name of the Resource Group in which the managed Kubernetes Cluster exists.
* @return A collection of values returned by getKubernetesCluster.
*/
public suspend fun getKubernetesCluster(name: String, resourceGroupName: String):
GetKubernetesClusterResult {
val argument = GetKubernetesClusterPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getKubernetesClusterResultToKotlin(getKubernetesClusterPlain(argument.toJava()).await())
}
/**
* @see [getKubernetesCluster].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesClusterPlainArgs].
* @return A collection of values returned by getKubernetesCluster.
*/
public suspend
fun getKubernetesCluster(argument: suspend GetKubernetesClusterPlainArgsBuilder.() -> Unit):
GetKubernetesClusterResult {
val builder = GetKubernetesClusterPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getKubernetesClusterResultToKotlin(getKubernetesClusterPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Kubernetes Node Pool Snapshot.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getKubernetesNodePoolSnapshot({
* name: "example",
* resourceGroupName: "example-resources",
* });
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_kubernetes_node_pool_snapshot(name="example",
* resource_group_name="example-resources")
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetKubernetesNodePoolSnapshot.Invoke(new()
* {
* Name = "example",
* ResourceGroupName = "example-resources",
* });
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* _, err := containerservice.GetKubernetesNodePoolSnapshot(ctx, &containerservice.GetKubernetesNodePoolSnapshotArgs{
* Name: "example",
* ResourceGroupName: "example-resources",
* }, nil)
* if err != nil {
* return err
* }
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetKubernetesNodePoolSnapshotArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getKubernetesNodePoolSnapshot(GetKubernetesNodePoolSnapshotArgs.builder()
* .name("example")
* .resourceGroupName("example-resources")
* .build());
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getKubernetesNodePoolSnapshot
* Arguments:
* name: example
* resourceGroupName: example-resources
* ```
*
* @param argument A collection of arguments for invoking getKubernetesNodePoolSnapshot.
* @return A collection of values returned by getKubernetesNodePoolSnapshot.
*/
public suspend
fun getKubernetesNodePoolSnapshot(argument: GetKubernetesNodePoolSnapshotPlainArgs):
GetKubernetesNodePoolSnapshotResult =
getKubernetesNodePoolSnapshotResultToKotlin(getKubernetesNodePoolSnapshotPlain(argument.toJava()).await())
/**
* @see [getKubernetesNodePoolSnapshot].
* @param name The name of the Kubernetes Node Pool Snapshot.
* @param resourceGroupName The name of the Resource Group in which the Kubernetes Node Pool Snapshot exists.
* @return A collection of values returned by getKubernetesNodePoolSnapshot.
*/
public suspend fun getKubernetesNodePoolSnapshot(name: String, resourceGroupName: String):
GetKubernetesNodePoolSnapshotResult {
val argument = GetKubernetesNodePoolSnapshotPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getKubernetesNodePoolSnapshotResultToKotlin(getKubernetesNodePoolSnapshotPlain(argument.toJava()).await())
}
/**
* @see [getKubernetesNodePoolSnapshot].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesNodePoolSnapshotPlainArgs].
* @return A collection of values returned by getKubernetesNodePoolSnapshot.
*/
public suspend
fun getKubernetesNodePoolSnapshot(argument: suspend GetKubernetesNodePoolSnapshotPlainArgsBuilder.() -> Unit):
GetKubernetesNodePoolSnapshotResult {
val builder = GetKubernetesNodePoolSnapshotPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getKubernetesNodePoolSnapshotResultToKotlin(getKubernetesNodePoolSnapshotPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to retrieve the version of Kubernetes supported by Azure Kubernetes Service.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const current = azure.containerservice.getKubernetesServiceVersions({
* location: "West Europe",
* });
* export const versions = current.then(current => current.versions);
* export const latestVersion = current.then(current => current.latestVersion);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* current = azure.containerservice.get_kubernetes_service_versions(location="West Europe")
* pulumi.export("versions", current.versions)
* pulumi.export("latestVersion", current.latest_version)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var current = Azure.ContainerService.GetKubernetesServiceVersions.Invoke(new()
* {
* Location = "West Europe",
* });
* return new Dictionary
* {
* ["versions"] = current.Apply(getKubernetesServiceVersionsResult => getKubernetesServiceVersionsResult.Versions),
* ["latestVersion"] = current.Apply(getKubernetesServiceVersionsResult => getKubernetesServiceVersionsResult.LatestVersion),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* current, err := containerservice.GetKubernetesServiceVersions(ctx, &containerservice.GetKubernetesServiceVersionsArgs{
* Location: "West Europe",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("versions", current.Versions)
* ctx.Export("latestVersion", current.LatestVersion)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetKubernetesServiceVersionsArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var current = ContainerserviceFunctions.getKubernetesServiceVersions(GetKubernetesServiceVersionsArgs.builder()
* .location("West Europe")
* .build());
* ctx.export("versions", current.applyValue(getKubernetesServiceVersionsResult -> getKubernetesServiceVersionsResult.versions()));
* ctx.export("latestVersion", current.applyValue(getKubernetesServiceVersionsResult -> getKubernetesServiceVersionsResult.latestVersion()));
* }
* }
* ```
* ```yaml
* variables:
* current:
* fn::invoke:
* Function: azure:containerservice:getKubernetesServiceVersions
* Arguments:
* location: West Europe
* outputs:
* versions: ${current.versions}
* latestVersion: ${current.latestVersion}
* ```
*
* @param argument A collection of arguments for invoking getKubernetesServiceVersions.
* @return A collection of values returned by getKubernetesServiceVersions.
*/
public suspend fun getKubernetesServiceVersions(argument: GetKubernetesServiceVersionsPlainArgs):
GetKubernetesServiceVersionsResult =
getKubernetesServiceVersionsResultToKotlin(getKubernetesServiceVersionsPlain(argument.toJava()).await())
/**
* @see [getKubernetesServiceVersions].
* @param includePreview Should Preview versions of Kubernetes in AKS be included? Defaults to `true`
* @param location Specifies the location in which to query for versions.
* @param versionPrefix A prefix filter for the versions of Kubernetes which should be returned; for example `1.` will return `1.9` to `1.14`, whereas `1.12` will return `1.12.2`.
* @return A collection of values returned by getKubernetesServiceVersions.
*/
public suspend fun getKubernetesServiceVersions(
includePreview: Boolean? = null,
location: String,
versionPrefix: String? = null,
): GetKubernetesServiceVersionsResult {
val argument = GetKubernetesServiceVersionsPlainArgs(
includePreview = includePreview,
location = location,
versionPrefix = versionPrefix,
)
return getKubernetesServiceVersionsResultToKotlin(getKubernetesServiceVersionsPlain(argument.toJava()).await())
}
/**
* @see [getKubernetesServiceVersions].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetKubernetesServiceVersionsPlainArgs].
* @return A collection of values returned by getKubernetesServiceVersions.
*/
public suspend
fun getKubernetesServiceVersions(argument: suspend GetKubernetesServiceVersionsPlainArgsBuilder.() -> Unit):
GetKubernetesServiceVersionsResult {
val builder = GetKubernetesServiceVersionsPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getKubernetesServiceVersionsResultToKotlin(getKubernetesServiceVersionsPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Container Registry.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getRegistry({
* name: "testacr",
* resourceGroupName: "test",
* });
* export const loginServer = example.then(example => example.loginServer);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_registry(name="testacr",
* resource_group_name="test")
* pulumi.export("loginServer", example.login_server)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetRegistry.Invoke(new()
* {
* Name = "testacr",
* ResourceGroupName = "test",
* });
* return new Dictionary
* {
* ["loginServer"] = example.Apply(getRegistryResult => getRegistryResult.LoginServer),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := containerservice.LookupRegistry(ctx, &containerservice.LookupRegistryArgs{
* Name: "testacr",
* ResourceGroupName: "test",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("loginServer", example.LoginServer)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetRegistryArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getRegistry(GetRegistryArgs.builder()
* .name("testacr")
* .resourceGroupName("test")
* .build());
* ctx.export("loginServer", example.applyValue(getRegistryResult -> getRegistryResult.loginServer()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getRegistry
* Arguments:
* name: testacr
* resourceGroupName: test
* outputs:
* loginServer: ${example.loginServer}
* ```
*
* @param argument A collection of arguments for invoking getRegistry.
* @return A collection of values returned by getRegistry.
*/
public suspend fun getRegistry(argument: GetRegistryPlainArgs): GetRegistryResult =
getRegistryResultToKotlin(getRegistryPlain(argument.toJava()).await())
/**
* @see [getRegistry].
* @param name The name of the Container Registry.
* @param resourceGroupName The Name of the Resource Group where this Container Registry exists.
* @return A collection of values returned by getRegistry.
*/
public suspend fun getRegistry(name: String, resourceGroupName: String): GetRegistryResult {
val argument = GetRegistryPlainArgs(
name = name,
resourceGroupName = resourceGroupName,
)
return getRegistryResultToKotlin(getRegistryPlain(argument.toJava()).await())
}
/**
* @see [getRegistry].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryPlainArgs].
* @return A collection of values returned by getRegistry.
*/
public suspend fun getRegistry(argument: suspend GetRegistryPlainArgsBuilder.() -> Unit):
GetRegistryResult {
val builder = GetRegistryPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRegistryResultToKotlin(getRegistryPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Container Registry scope map.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getRegistryScopeMap({
* name: "example-scope-map",
* resourceGroupName: "example-resource-group",
* containerRegistryName: "example-registry",
* });
* export const actions = example.then(example => example.actions);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_registry_scope_map(name="example-scope-map",
* resource_group_name="example-resource-group",
* container_registry_name="example-registry")
* pulumi.export("actions", example.actions)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetRegistryScopeMap.Invoke(new()
* {
* Name = "example-scope-map",
* ResourceGroupName = "example-resource-group",
* ContainerRegistryName = "example-registry",
* });
* return new Dictionary
* {
* ["actions"] = example.Apply(getRegistryScopeMapResult => getRegistryScopeMapResult.Actions),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := containerservice.LookupRegistryScopeMap(ctx, &containerservice.LookupRegistryScopeMapArgs{
* Name: "example-scope-map",
* ResourceGroupName: "example-resource-group",
* ContainerRegistryName: "example-registry",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("actions", example.Actions)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetRegistryScopeMapArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getRegistryScopeMap(GetRegistryScopeMapArgs.builder()
* .name("example-scope-map")
* .resourceGroupName("example-resource-group")
* .containerRegistryName("example-registry")
* .build());
* ctx.export("actions", example.applyValue(getRegistryScopeMapResult -> getRegistryScopeMapResult.actions()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getRegistryScopeMap
* Arguments:
* name: example-scope-map
* resourceGroupName: example-resource-group
* containerRegistryName: example-registry
* outputs:
* actions: ${example.actions}
* ```
*
* @param argument A collection of arguments for invoking getRegistryScopeMap.
* @return A collection of values returned by getRegistryScopeMap.
*/
public suspend fun getRegistryScopeMap(argument: GetRegistryScopeMapPlainArgs):
GetRegistryScopeMapResult =
getRegistryScopeMapResultToKotlin(getRegistryScopeMapPlain(argument.toJava()).await())
/**
* @see [getRegistryScopeMap].
* @param containerRegistryName The Name of the Container Registry where the token exists.
* @param name The name of the Container Registry token.
* @param resourceGroupName The Name of the Resource Group where this Container Registry token exists.
* @return A collection of values returned by getRegistryScopeMap.
*/
public suspend fun getRegistryScopeMap(
containerRegistryName: String,
name: String,
resourceGroupName: String,
): GetRegistryScopeMapResult {
val argument = GetRegistryScopeMapPlainArgs(
containerRegistryName = containerRegistryName,
name = name,
resourceGroupName = resourceGroupName,
)
return getRegistryScopeMapResultToKotlin(getRegistryScopeMapPlain(argument.toJava()).await())
}
/**
* @see [getRegistryScopeMap].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryScopeMapPlainArgs].
* @return A collection of values returned by getRegistryScopeMap.
*/
public suspend
fun getRegistryScopeMap(argument: suspend GetRegistryScopeMapPlainArgsBuilder.() -> Unit):
GetRegistryScopeMapResult {
val builder = GetRegistryScopeMapPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRegistryScopeMapResultToKotlin(getRegistryScopeMapPlain(builtArgument.toJava()).await())
}
/**
* Use this data source to access information about an existing Container Registry token.
* ## Example Usage
*
* ```typescript
* import * as pulumi from "@pulumi/pulumi";
* import * as azure from "@pulumi/azure";
* const example = azure.containerservice.getRegistryToken({
* name: "exampletoken",
* resourceGroupName: "example-resource-group",
* containerRegistryName: "example-registry",
* });
* export const scopeMapId = example.then(example => example.scopeMapId);
* ```
* ```python
* import pulumi
* import pulumi_azure as azure
* example = azure.containerservice.get_registry_token(name="exampletoken",
* resource_group_name="example-resource-group",
* container_registry_name="example-registry")
* pulumi.export("scopeMapId", example.scope_map_id)
* ```
* ```csharp
* using System.Collections.Generic;
* using System.Linq;
* using Pulumi;
* using Azure = Pulumi.Azure;
* return await Deployment.RunAsync(() =>
* {
* var example = Azure.ContainerService.GetRegistryToken.Invoke(new()
* {
* Name = "exampletoken",
* ResourceGroupName = "example-resource-group",
* ContainerRegistryName = "example-registry",
* });
* return new Dictionary
* {
* ["scopeMapId"] = example.Apply(getRegistryTokenResult => getRegistryTokenResult.ScopeMapId),
* };
* });
* ```
* ```go
* package main
* import (
* "github.com/pulumi/pulumi-azure/sdk/v5/go/azure/containerservice"
* "github.com/pulumi/pulumi/sdk/v3/go/pulumi"
* )
* func main() {
* pulumi.Run(func(ctx *pulumi.Context) error {
* example, err := containerservice.LookupRegistryToken(ctx, &containerservice.LookupRegistryTokenArgs{
* Name: "exampletoken",
* ResourceGroupName: "example-resource-group",
* ContainerRegistryName: "example-registry",
* }, nil)
* if err != nil {
* return err
* }
* ctx.Export("scopeMapId", example.ScopeMapId)
* return nil
* })
* }
* ```
* ```java
* package generated_program;
* import com.pulumi.Context;
* import com.pulumi.Pulumi;
* import com.pulumi.core.Output;
* import com.pulumi.azure.containerservice.ContainerserviceFunctions;
* import com.pulumi.azure.containerservice.inputs.GetRegistryTokenArgs;
* import java.util.List;
* import java.util.ArrayList;
* import java.util.Map;
* import java.io.File;
* import java.nio.file.Files;
* import java.nio.file.Paths;
* public class App {
* public static void main(String[] args) {
* Pulumi.run(App::stack);
* }
* public static void stack(Context ctx) {
* final var example = ContainerserviceFunctions.getRegistryToken(GetRegistryTokenArgs.builder()
* .name("exampletoken")
* .resourceGroupName("example-resource-group")
* .containerRegistryName("example-registry")
* .build());
* ctx.export("scopeMapId", example.applyValue(getRegistryTokenResult -> getRegistryTokenResult.scopeMapId()));
* }
* }
* ```
* ```yaml
* variables:
* example:
* fn::invoke:
* Function: azure:containerservice:getRegistryToken
* Arguments:
* name: exampletoken
* resourceGroupName: example-resource-group
* containerRegistryName: example-registry
* outputs:
* scopeMapId: ${example.scopeMapId}
* ```
*
* @param argument A collection of arguments for invoking getRegistryToken.
* @return A collection of values returned by getRegistryToken.
*/
public suspend fun getRegistryToken(argument: GetRegistryTokenPlainArgs): GetRegistryTokenResult =
getRegistryTokenResultToKotlin(getRegistryTokenPlain(argument.toJava()).await())
/**
* @see [getRegistryToken].
* @param containerRegistryName The Name of the Container Registry where the token exists.
* @param name The name of the Container Registry token.
* @param resourceGroupName The Name of the Resource Group where this Container Registry token exists.
* @return A collection of values returned by getRegistryToken.
*/
public suspend fun getRegistryToken(
containerRegistryName: String,
name: String,
resourceGroupName: String,
): GetRegistryTokenResult {
val argument = GetRegistryTokenPlainArgs(
containerRegistryName = containerRegistryName,
name = name,
resourceGroupName = resourceGroupName,
)
return getRegistryTokenResultToKotlin(getRegistryTokenPlain(argument.toJava()).await())
}
/**
* @see [getRegistryToken].
* @param argument Builder for [com.pulumi.azure.containerservice.kotlin.inputs.GetRegistryTokenPlainArgs].
* @return A collection of values returned by getRegistryToken.
*/
public suspend
fun getRegistryToken(argument: suspend GetRegistryTokenPlainArgsBuilder.() -> Unit):
GetRegistryTokenResult {
val builder = GetRegistryTokenPlainArgsBuilder()
builder.argument()
val builtArgument = builder.build()
return getRegistryTokenResultToKotlin(getRegistryTokenPlain(builtArgument.toJava()).await())
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy